developers can utilize to make their code more efficient, readable, and maintainable. However, amidst the vast library, there are a few functions that often get overlooked despite their usefulness. We're going to take a look at the top 5.
(This list is just our opinion. Sorry, no hard data on this one!)
array_filter()
1. The array_filter()
function is incredibly handy when you need to filter elements in an array. Yet, it's often overlooked in favour of manual array iterations. This function filters elements of an array using a callback function, returning an array with only elements that pass a truth test in the callback.
$numbers = [1, 2, 3, 4, 5, 6];
$evenNumbers = array_filter($numbers, function($value) {
return $value % 2 == 0;
});
// Result: Array([1] => 2, [3] => 4, [5] => 6)
2. list() or []
The list()
function, or the shorthand [] syntax, is an often overlooked but useful feature for array destructuring or unpacking. It allows you to assign variables as if they were an array.
$data = ['Alice', 'Bob', 'Charlie'];
list($first, $second, $third) = $data;
// The variables now hold the values
// $first: 'Alice'
// $second: 'Bob'
// $third: 'Charlie'
3. compact()
The compact()
function is used to create an array from variables and their values. It is often overlooked, but it can be very handy, particularly when working with databases or templates where you need to pass an associative array.
$name = 'Alice';
$age = 25;
$city = 'Wonderland';
$data = compact('name', 'age', 'city');
// Result: Array([name] => Alice, [age] => 25, [city] => Wonderland)
4. str_split()
Often overlooked for string manipulation, the str_split()
function splits a string into an array. This function is handy when you need to work with a string character by character.
$string = 'hello';
$array = str_split($string);
// Result: Array([0] => h, [1] => e, [2] => l, [3] => l, [4] => o)
5. usort()
PHP's usort()
function is a powerful tool that allows developers to sort arrays based on user-defined comparison functions. This function gives you a lot more control over how your arrays are sorted, but it's often overlooked in favor of simpler, less flexible sorting functions.
$users = [
['name' => 'Alice', 'age' => 25],
['name' => 'Bob', 'age' => 30],
['name' => 'Charlie', 'age' => 20],
];
usort($users, function($a, $b) {
return $a['age'] <=> $b['age'];
});
// Users array is now sorted by age
From array manipulation to string handling, these are just a few of the many powerful, yet often overlooked, functions that PHP has to offer. So the next time you find yourself manually manipulating data or writing redundant code, remember these functions. They can save you time, make your code cleaner, and optimize your applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
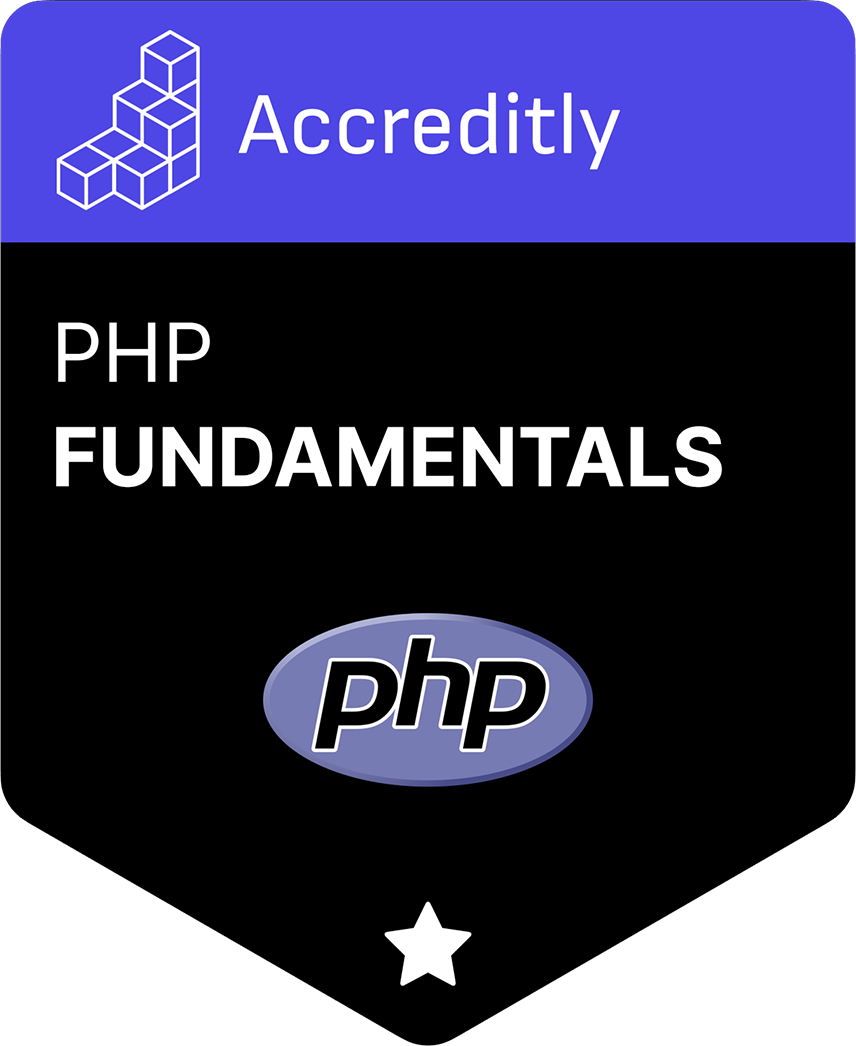