- 1. Setting Up Electron
- 2. Creating the Main Process
- 3. Building the Render Process
- 4. Storing Multiple Clipboard Entries
- 5. Adding Styles and Polishing (Optional)
- 6. Running the App
Electron JS offers a robust platform to build native desktop applications using JavaScript, HTML, and CSS. In this tutorial, we'll create a simple clipboard manager using Electron that will allow users to store and access multiple clipboard entries.
1. Setting Up Electron
Before starting, ensure you have Node.js installed.
Step 1: Initialize a new Node.js application:
mkdir electron-clipboard-manager
cd electron-clipboard-manager
npm init -y
Step 2: Install Electron:
npm install electron --save-dev
2. Creating the Main Process
Create a new file main.js
. This file will contain the logic for the main process of the Electron app.
In main.js
, set up a basic window:
const { app, BrowserWindow } = require('electron');
let mainWindow;
app.on('ready', function() {
mainWindow = new BrowserWindow({
width: 600,
height: 400,
webPreferences: {
nodeIntegration: true
}
});
mainWindow.loadFile('index.html');
});
app.on('window-all-closed', function() {
app.quit();
});
3. Building the Render Process
Create an index.html
file:
<!DOCTYPE html>
<html>
<head>
<title>Clipboard Manager</title>
</head>
<body>
<h1>Clipboard Manager</h1>
<button id="copy">Copy Text</button>
<button id="paste">Paste Text</button>
<div id="clipboard-contents"></div>
<script src="renderer.js"></script>
</body>
</html>
Now, let's handle the clipboard operations in the renderer process. Create a renderer.js
:
const { clipboard } = require('electron');
const copyButton = document.getElementById('copy');
const pasteButton = document.getElementById('paste');
const clipboardContents = document.getElementById('clipboard-contents');
copyButton.addEventListener('click', () => {
const textToCopy = 'Hello, Electron!';
clipboard.writeText(textToCopy);
clipboardContents.textContent = 'Copied: ' + textToCopy;
});
pasteButton.addEventListener('click', () => {
const pastedText = clipboard.readText();
clipboardContents.textContent = 'Pasted: ' + pastedText;
});
4. Storing Multiple Clipboard Entries
For simplicity, let's use an array to store multiple clipboard entries:
Modify the renderer.js
:
// ... other code ...
let clipboardHistory = [];
copyButton.addEventListener('click', () => {
const textToCopy = 'Hello, Electron!';
clipboard.writeText(textToCopy);
clipboardHistory.push(textToCopy);
renderClipboardHistory();
});
pasteButton.addEventListener('click', () => {
const pastedText = clipboard.readText();
clipboardHistory.push(pastedText);
renderClipboardHistory();
});
function renderClipboardHistory() {
clipboardContents.innerHTML = clipboardHistory.map(item => `<p>${item}</p>`).join('');
}
5. Adding Styles and Polishing (Optional)
Add some basic styles to your index.html
to make the application look better:
<head>
<!-- ... -->
<style> body {
font-family: Arial, sans-serif;
text-align: center;
padding: 2em;
}
button {
margin: 1em;
padding: 0.5em 1em;
} </style>
</head>
6. Running the App
In your package.json
, modify the "scripts" section:
"scripts": {
"start": "electron main.js"
}
Now, run the app:
npm start
Congratulations, you've built a basic clipboard manager using Electron JS! From here, you can expand the functionality, integrate with cloud services for synchronized clipboards across devices, or add more advanced features like clipboard history search. Happy coding!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
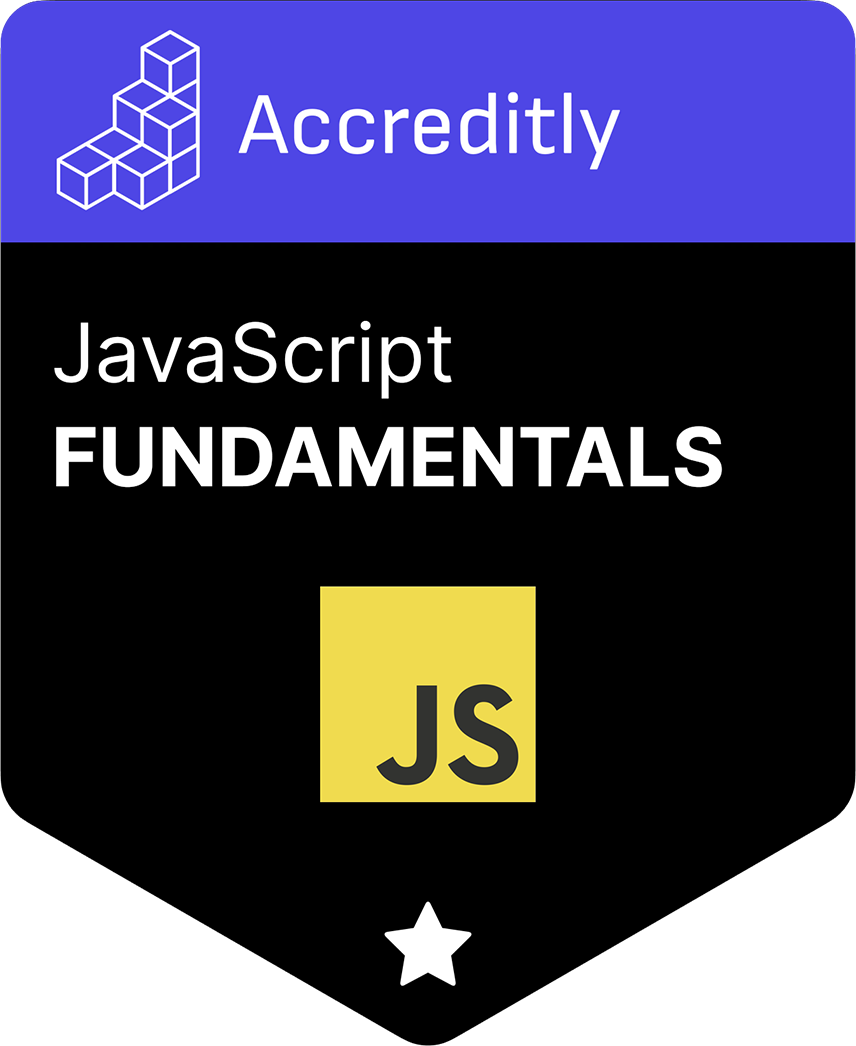
Related articles
Tutorials WordPress PHP Cyber Security
How to check your WordPress core installation for unauthorised changes
Safeguard your WordPress site by learning how to check your WordPress core installation for unauthorised changes. This article provides a step-by-step approach to maintaining the security and integrity of your WordPress core files.