- Step 1: Creating a New Page for Your Login Form
- Step 2: Building Your Login Form
- Step 3: Handling Form Submission
- Step 4: Redirecting Users After Successful Login
- Step 5: Adding Error Handling
In this WordPress developer tutorial, we will show you how to create a form that allows your users to log into WordPress using your own custom form, rather than the standard WordPress login. This can be useful when trying to create a more integrated experience on your website.
Step 1: Creating a New Page for Your Login Form
In your WordPress dashboard, navigate to Pages > Add New. Title your new page "Login". Leave the body of the page empty for now and publish the page.
Step 2: Building Your Login Form
For simplicity, we'll build our form using HTML. Below is a basic structure for your login form:
<form name="loginform" id="loginform" action="" method="post">
<p>
<label for="user_login">Username<br />
<input type="text" name="log" id="user_login" class="input" value="" size="20"></label>
</p>
<p>
<label for="user_pass">Password<br />
<input type="password" name="pwd" id="user_pass" class="input" value="" size="20"></label>
</p>
<p class="forgetmenot">
<label for="rememberme"><input name="rememberme" type="checkbox" id="rememberme" value="forever"> Remember Me</label>
</p>
<p class="submit">
<input type="submit" name="wp-submit" id="wp-submit" class="button button-primary button-large" value="Log In">
<input type="hidden" name="redirect_to" value="">
</p>
</form>
Paste this form into the body of the 'Login' page you created earlier. You can modify the HTML to match your website's style and branding.
Step 3: Handling Form Submission
WordPress provides the wp_login_form()
function, which generates the necessary HTML for a login form, and also handles the login and authentication process.
Replace the action=""
in the form HTML with <?php echo site_url('wp-login.php') ?>
to direct the form submissions to the wp-login.php
file in the WordPress core. This is the file that handles authentication.
<form name="loginform" id="loginform" action="<?php echo site_url('wp-login.php') ?>" method="post">
Step 4: Redirecting Users After Successful Login
WordPress also allows you to redirect users to a specific page after successful login. By using the redirect_to
hidden input field, you can specify the URL to which users should be redirected.
Replace the value=""
in the redirect_to
input with the URL of your choice:
<input type="hidden" name="redirect_to" value="<?php echo site_url('your-page') ?>">
Step 5: Adding Error Handling
You can also manage login errors by using the wp_login_failed
action hook. This hook lets you redirect users back to the login page and append a query string that indicates a login error:
function custom_login_fail( $username ) {
$referrer = $_SERVER['HTTP_REFERER'];
if ( !empty($referrer) && !strstr($referrer,'wp-login') && !strstr($referrer,'wp-admin') ) {
wp_redirect( add_query_arg('login', 'failed', $referrer) );
exit;
}
}
add_action( 'wp_login_failed', 'custom_login_fail' );
You can put this snippet in your theme's functions.php
file. Now, when a login attempt fails, the user is redirected back to the login page with ?login=failed
added to the URL. You can then display an error message based on this query string.
Remember, the more secure, user-friendly, and streamlined your login process, the better experience your users will have on your website. Give it a go by visiting your login screen. Your usual WordPress login credentials will work fine.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
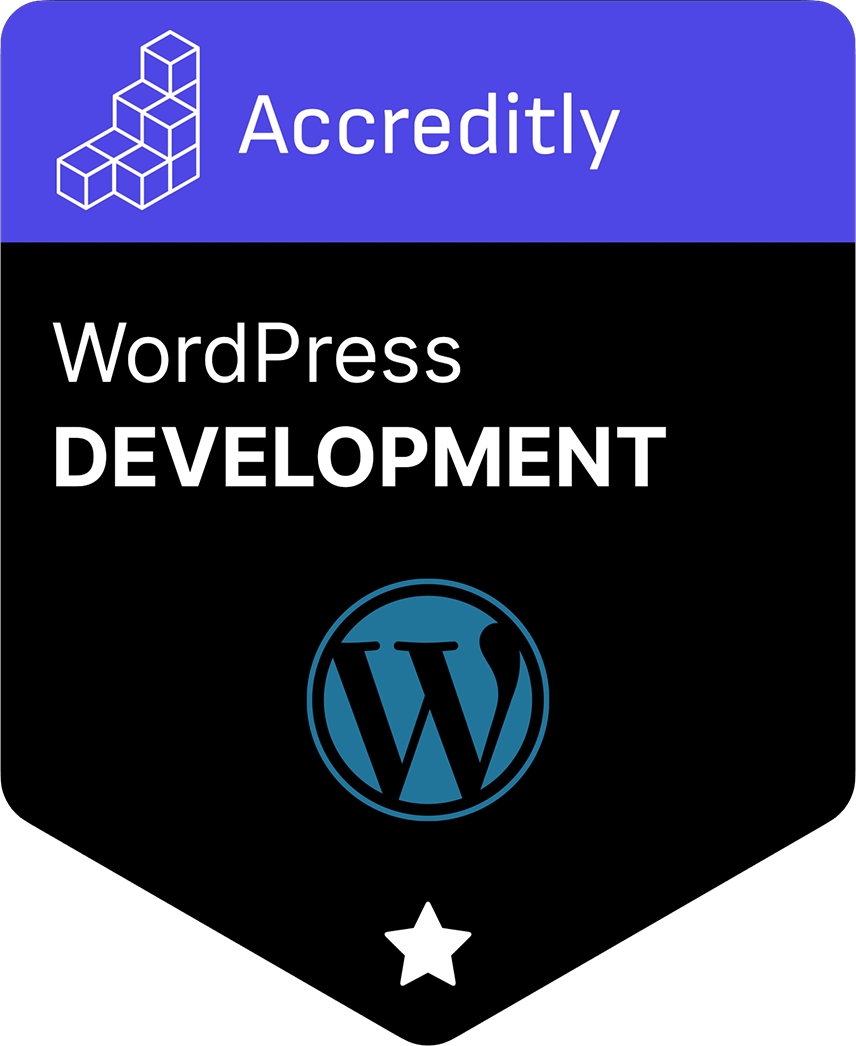