A user's internet connection speed can significantly impact their experience on your website or web application. Slow loading times may lead to frustration and potential disengagement. Detecting a slow internet connection can allow you to adapt your website to these users by optimizing data usage, enabling a 'lite' version of your site, or providing appropriate feedback to the user about their current internet connection. This article will guide you on how to detect a slow internet connection using JavaScript.
The Navigator Connection API
The most straightforward method to detect internet speed is by using the Navigator Connection API. This API provides information about the system's connection in terms of downlink, effective type, and round-trip time.
Here's a simple example:
if (navigator.connection) {
console.log(`Effective network type: ${navigator.connection.effectiveType}`);
console.log(`Downlink Speed: ${navigator.connection.downlink}Mb/s`);
console.log(`Round Trip Time: ${navigator.connection.rtt}ms`);
} else {
console.log('Navigator Connection API not supported');
}
In the code snippet above, the effectiveType
attribute provides a string that represents the effective type of the connection, and it could be 'slow-2g', '2g', '3g', or '4g'.
Perform a Speed Test
The Navigator Connection API may not be supported in all browsers or may not provide the level of detail you require. If you want a more accurate assessment of the user's internet speed, you can conduct a speed test using JavaScript. This method involves loading a file from your server and measuring how long it takes.
Here is a sample implementation:
let startTime, endTime;
let downloadSize = 1024 * 1024 * 5; // Size of download test file in bytes
function startDownloadTest() {
startTime = (new Date()).getTime();
var download = new Image();
download.src = 'path-to-your-test-image?' + startTime;
download.onload = function() {
endTime = (new Date()).getTime();
calculateDownloadSpeed();
}
}
function calculateDownloadSpeed() {
var duration = (endTime - startTime) / 1000;
var bitsLoaded = downloadSize * 8;
var bps = (bitsLoaded / duration).toFixed(2);
var kbps = (bps / 1024).toFixed(2);
var mbps = (kbps / 1024).toFixed(2);
console.log(`Your connection speed:
${bps} bps
${kbps} kbps
${mbps} mbps`);
}
startDownloadTest();
In the code above, an image is downloaded from the server, and the start and end times are tracked. By dividing the size of the file by the time it took to download, you can estimate the speed of the user's internet connection.
Remember that on slow connections downloading a file like this may make things even worse, so it may be worth adding some code to detect the slow connection and kill the transfer.
Considerations
It's important to note that both methods have limitations.
The Navigator Connection API does not provide an exact measurement of the user's current internet speed. It merely gives a general indication of their connection type. In contrast, the speed test method provides a more accurate assessment but can consume data, which might not be ideal for users with limited data plans. Therefore, it's crucial to inform users that a data-intensive operation is about to happen.
Always remember to enhance your user's experience and not hinder it when performing such operations.
With the methods discussed, you can now effectively detect a user's internet connection speed and adapt your web application accordingly. Remember, providing optimal user experience regardless of connection speed is a significant part of inclusive design; in other words, you shouldn't be designing something that a portion of your users can't access in the first place.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
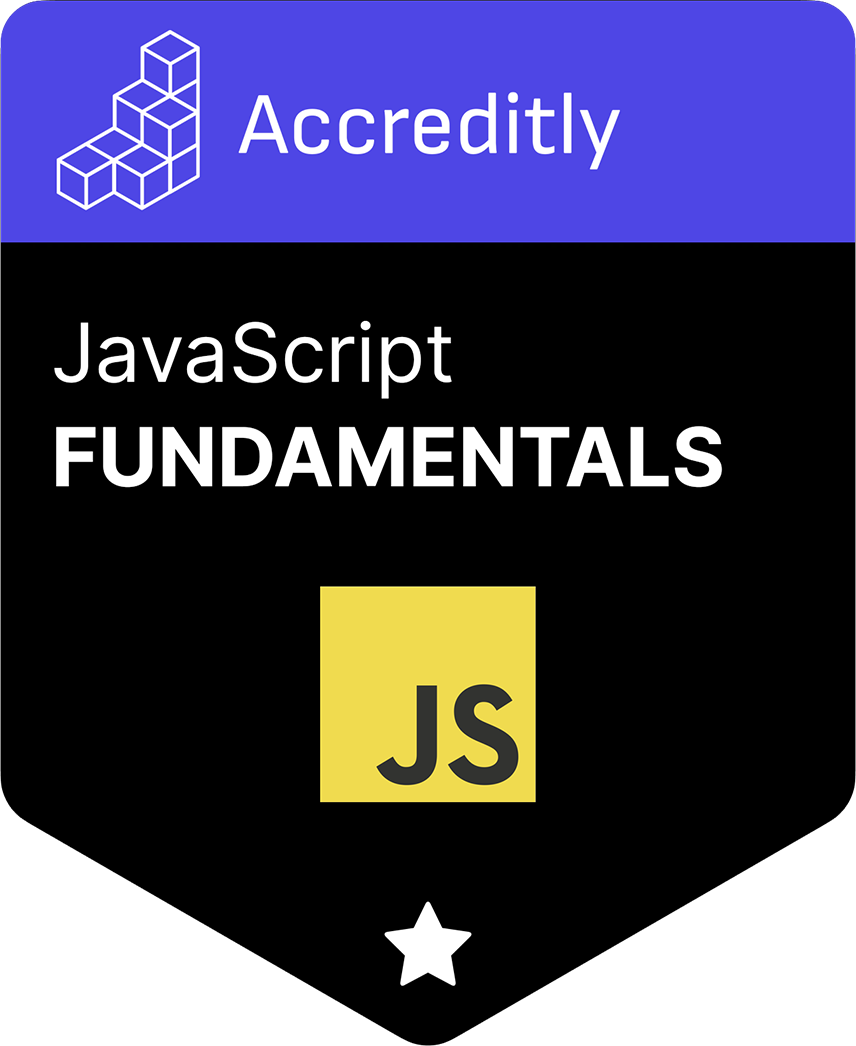