- Setting Up a Slack Webhook
- Configuring Laravel to Use the Slack Webhook
- Testing the Integration
- Customizing Error Notifications
- Best Practices
Quick responses to errors is crucial in any application. Laravel, being a robust PHP framework, offers extensive logging capabilities. By integrating Laravel with Slack using webhooks, you can receive real-time notifications of errors, enabling immediate action. This setup enhances your team's responsiveness to issues, ultimately leading to a more stable application. Let's explore how to send errors from Laravel to Slack using webhooks.
Setting Up a Slack Webhook
First, you need to create a webhook in Slack:
-
Create a New Slack App: Go to the Your Apps page on Slack API and create a new app. Choose the workspace where you want to send notifications.
-
Enable Incoming Webhooks: In the Slack app settings, go to "Incoming Webhooks" and activate the feature.
-
Create a Webhook URL: Select the channel or user to receive the notifications, then click on 'Add New Webhook to Workspace' and follow the prompts. Slack will generate a webhook URL.
Configuring Laravel to Use the Slack Webhook
With your Slack webhook URL in hand, you can now configure Laravel to send errors to Slack.
-
Set the Webhook URL in
.env
File:
LOG_SLACK_WEBHOOK_URL=https://hooks.slack.com/services/T00000000/B00000000/XXXXXXXXXXXXXXXXXXXXXXXX
Replace the URL with your Slack webhook URL.
-
Configure
logging.php
:
In your config/logging.php
file, set up a Slack channel configuration:
'channels' => [
// Other channels...
'slack' => [
'driver' => 'slack',
'url' => env('LOG_SLACK_WEBHOOK_URL'),
'username' => 'Laravel Error Bot',
'emoji' => ':boom:', // Slack emoji icon
'level' => 'error',
],
],
This configuration tells Laravel to use Slack for logging errors, using the webhook URL from your .env
file.
Testing the Integration
To test if errors are properly sent to Slack, trigger an exception in your Laravel application:
Route::get('/test-error', function () {
logger()->error('Test error from Laravel to Slack!');
});
Visit /test-error
in your application, and you should see the test error message in your designated Slack channel.
Customizing Error Notifications
You can customize how errors are reported to Slack. For instance, you might want to include additional details like the environment or URL where the error occurred:
Log::channel('slack')->error('An error occurred', [
'env' => app()->environment(),
'url' => request()->url(),
// Other details...
]);
Best Practices
- Use Different Channels for Environments: Consider using different Slack channels for different environments (e.g., production, staging) to organize error notifications effectively.
- Rate Limiting: Be mindful of Slack's rate limits. If your application generates a lot of errors, consider implementing a throttling mechanism to avoid spamming the channel.
- Security: Be cautious about the information sent to Slack. Avoid sending sensitive data in error messages.
Integrating Laravel with Slack for error reporting is a powerful way to enhance your application's monitoring and response systems. By setting up Slack webhooks and configuring Laravel to send errors to Slack, you can ensure that your team is always in the loop about the health and stability of your application, allowing for rapid response and resolution of issues.
Staying informed about errors in real-time can significantly improve your application's reliability and your team's efficiency. This integration is a testament to Laravel's flexibility and Slack's utility in building a robust error monitoring and alerting system.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
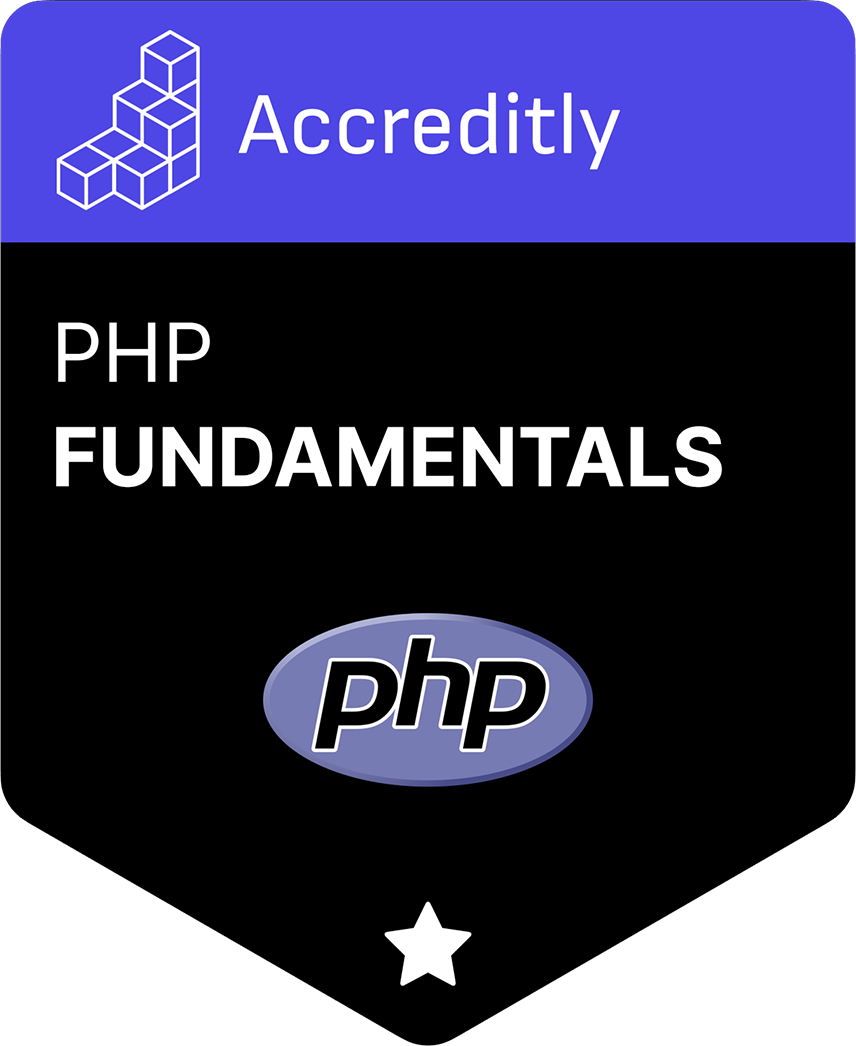