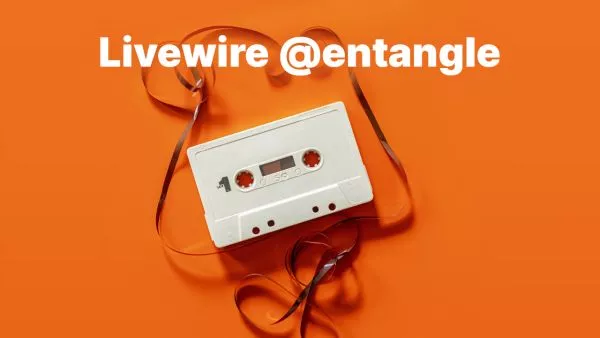
Laravel Livewire is a full-stack framework that allows you to create dynamic, interactive user interfaces using PHP, as if you were using JavaScript. One of its many interesting features is the @entangle
directive. In this tutorial, we will dive into this directive, discuss why you would use it, where to use it, and how to use it with code examples.
@entangle
?
What is In Livewire, @entangle
is a directive that allows you to seamlessly bind a Livewire component's property to an Alpine.js state property. This is particularly useful when you're working on an interface that requires a lot of interactive elements and you want to use the power of both Livewire (server-side) and Alpine.js (client-side) to create a seamless user experience.
@entangle
?
Why Use If you are using Alpine.js for your JavaScript and Livewire for your backend, there might be instances where you need both to interact. This is where @entangle
comes in. It allows you to bind Livewire component properties with Alpine.js, essentially linking server-side and client-side together. This way, changes in your Livewire component will reflect in your Alpine.js component and vice versa.
@entangle
How to Use Let's see a practical example. Assume you have a Livewire component with a boolean
property called showModal
. You also have a modal in your view, and you're using Alpine.js to handle showing and hiding the modal. Here's how you can use @entangle
to link the showModal
property with the modal's visibility:
// Livewire Component
class MyComponent extends \Livewire\Component
{
public $showModal = false;
public function render()
{
return view('livewire.my-component');
}
}
<!-- Livewire View -->
<div x-data="{ open: @entangle('showModal') }">
<button @click="open = true">Open Modal</button>
<div x-show="open" @click.away="open = false">
<!-- Modal Content -->
</div>
</div>
In the example above, when the button is clicked, the Alpine.js state property open is set to true. Since it's entangled with Livewire's showModal property, setting open to true also sets showModal to true. Conversely, any changes made to the showModal property on the server-side will also update the open property on the client-side.
@entangle
When to Use You would typically use @entangle
when you need to reflect a change in your Livewire component's state in your Alpine.js component without making a full roundtrip to the server, or vice versa. In other words, it's useful when you need real-time, bi-directional data binding between Livewire and Alpine.js.
In summary, the @entangle
directive provides a powerful way to integrate Livewire and Alpine.js, making it easier to create real-time, interactive user interfaces. While this tutorial provided a basic introduction, the potential applications are vast, so don't hesitate to experiment and explore further!
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
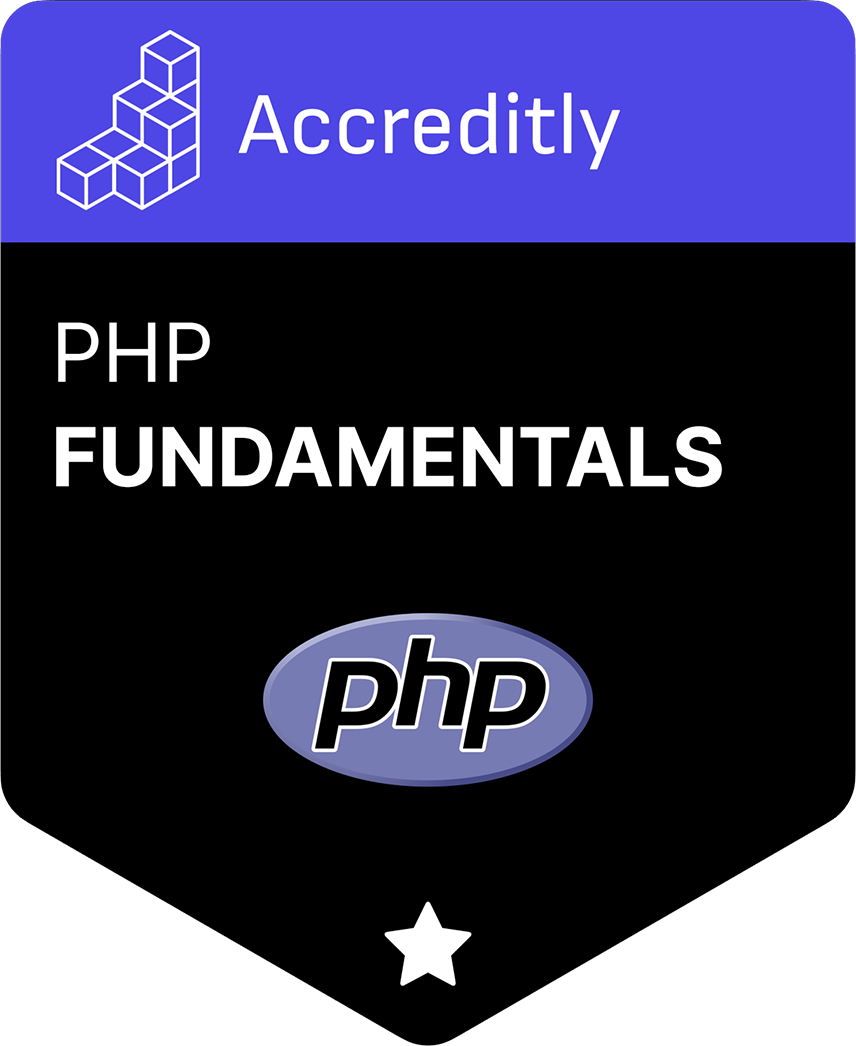