Laravel is a powerful and feature-rich PHP web application framework that provides numerous built-in functions, making it easier for developers to build and maintain their applications. While Laravel's official documentation covers many of its features, some lesser-known functions can be incredibly helpful in certain situations. In this article, we'll uncover some of these hidden gems and provide usage examples to help you make the most of Laravel's extensive capabilities.
These functions are all helpers which can be found in Laravel's helper documentation.
data_get()
The data_get()
function is a convenient way to retrieve a value from a nested array or object using "dot" notation, with the option to specify a default value if the desired key is not found. This can be especially helpful when working with deeply nested data structures.
Example:
$data = [
'user' => [
'name' => 'John Doe',
'email' => '[email protected]',
'preferences' => [
'theme' => 'dark',
'language' => 'en'
]
]
];
$theme = data_get($data, 'user.preferences.theme', 'default');
echo $theme; // Output: dark
optional()
The optional()
function is a handy utility to call a method on an object if it exists, otherwise, return a default value. This can help you write cleaner code by avoiding multiple checks for object existence before calling a method.
Example:
Suppose you have a User
model with a relationship to a Company
model:
class User extends Model {
public function company() {
return $this->belongsTo(Company::class);
}
}
To get the name of the user's company, you might typically write:
$companyName = $user->company ? $user->company->name : 'N/A';
With optional()
, you can simplify this code:
$companyName = optional($user->company)->name ?? 'N/A';
tap()
The tap()
function allows you to "tap" into an object, execute a closure, and then return the original object. This can be useful when you need to perform additional actions on an object without breaking the method chaining.
Example:
Imagine you want to create a new user and log an entry whenever a user is created. Without tap()
, you might write:
$user = new User(['name' => 'John Doe', 'email' => '[email protected]']);
$user->save();
\Log::info("User {$user->id} created.");
// Continue with other tasks
Using tap()
, you can streamline this code:
$user = tap(new User(['name' => 'John Doe', 'email' => '[email protected]']), function ($user) {
$user->save();
\Log::info("User {$user->id} created.");
});
// Continue with other tasks
rescue()
The rescue()
function is a useful tool for handling exceptions within your code. You can provide a closure that may throw an exception and a default value to return if an exception is caught. Optionally, you can pass a callback that will be executed if an exception occurs.
Example:
Imagine you have a function that fetches data from an API and may throw an exception if the API is unreachable:
function fetchData() {
// Code that fetches data and may throw an exception
}
Using rescue()
, you can handle exceptions gracefully and provide a default value:
$data = rescue(function () {
return fetchData();
}, [], function ($exception) {
\Log::error("Error fetching data: {$exception->getMessage()}");
});
In this example, if fetchData()
throws an exception, rescue()
will catch it, log the error message, and return an empty array as the default value.
with()
The with()
function can be used to return a value from a closure, making it useful for one-liners or inline assignments.
Example:
Suppose you have an array of user data and want to create a new instance of the User
model with that data:
$data = [
'name' => 'John Doe',
'email' => '[email protected]'
];
Without with()
, you might write:
$user = new User($data);
$user->save();
// Do something with $user
Using with()
, you can simplify the code:
$user = with(new User($data))->save();
// Do something with $user
throw_if()
and throw_unless()
The throw_if()
and throw_unless()
functions can be used to conditionally throw exceptions based on the truthiness of a given value. These functions help make your code more readable and expressive.
Example:
Consider a scenario where you want to throw an exception if a user's age is below a certain threshold:
$age = 15;
if ($age < 18) {
throw new \Exception('User is not old enough.');
}
Using throw_if()
, you can rewrite the code more expressively:
throw_if($age < 18, new \Exception('User is not old enough.'));
Similarly, you can use throw_unless()
to throw an exception when a condition is false:
throw_unless($age >= 18, new \Exception('User is not old enough.'));
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
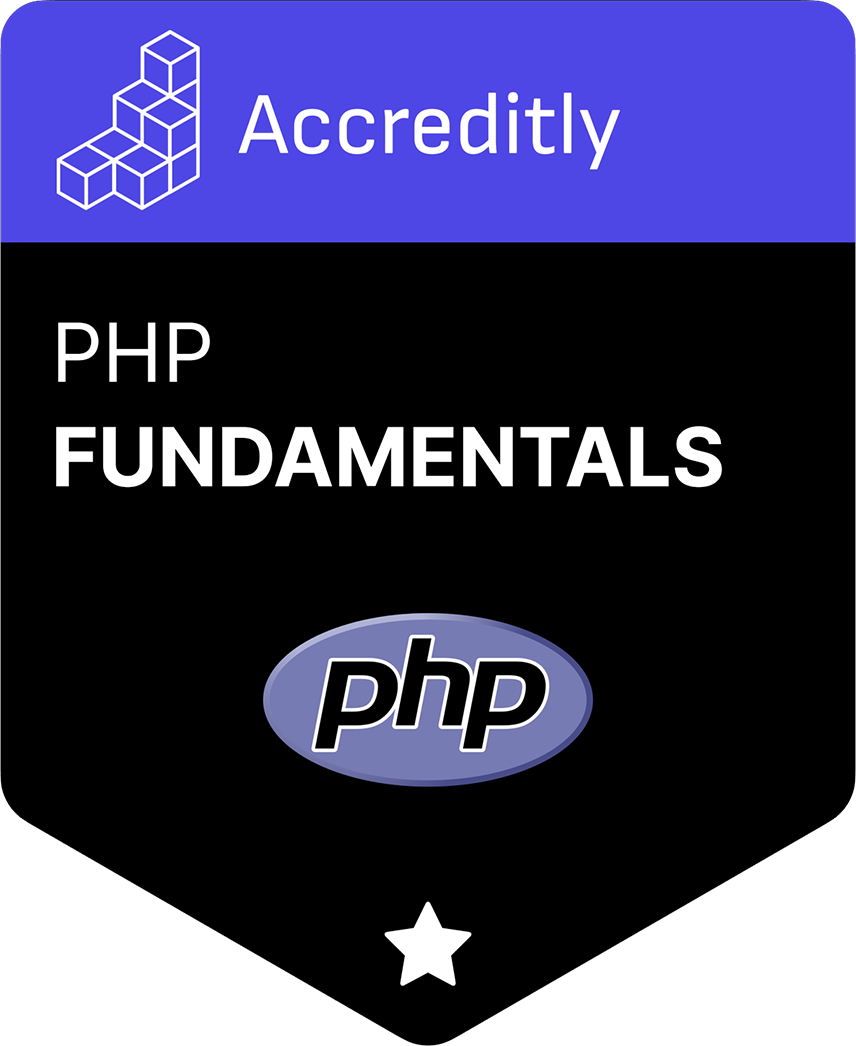
Related articles
Tutorials WordPress PHP Tooling
Optimizing WordPress YouTube Embeds with Lite YouTube Embed
Learn how to enhance your WordPress site's performance by replacing the native YouTube embed with Lite YouTube Embed. This guide takes you through the steps to override the default oEmbed behaviour for YouTube videos.