- Understanding Closures
- How Closures Work
- Practical Uses of Closures
- Closures and Memory Management
- Advanced Closure Concepts
JavaScript closures are a fundamental and powerful feature of the language, essential for every developer to understand. Closures allow functions to access and manipulate variables from an outer scope, even after that outer scope has closed. This extensive guide will explore closures in depth, providing a thorough understanding along with practical examples.
Understanding Closures
At its core, a closure is a function that remembers the environment in which it was created. This environment includes any variables that were in scope at the time of the closure's creation.
Basic Example of a Closure
function createGreeting(greeting) {
return function(name) {
return greeting + ', ' + name;
};
}
const sayHello = createGreeting('Hello');
console.log(sayHello('Alice')); // Outputs: Hello, Alice
In this example, sayHello
is a closure that remembers the value of greeting
from the outer function createGreeting
.
How Closures Work
Closures keep a reference to the outer scope in which they were created. JavaScript's garbage collection mechanism does not clean up variables that are referenced by closures, allowing these variables to persist.
Understanding Scope and Closure
function outerFunction() {
let outerVariable = 'I am outside!';
function innerFunction() {
console.log(outerVariable); // Accesses outerVariable
}
return innerFunction;
}
const myInnerFunction = outerFunction();
myInnerFunction(); // Outputs: I am outside!
The innerFunction
closure retains access to outerVariable
, even after outerFunction
has completed execution.
Practical Uses of Closures
Closures are not merely theoretical constructs; they have practical applications:
Data Privacy
Closures can create private variables and methods, a technique often used in the module pattern.
function createCounter() {
let count = 0;
return {
increment: function() { count++; },
getCount: function() { return count; }
};
}
const counter = createCounter();
counter.increment();
console.log(counter.getCount()); // Outputs: 1
In this example, count
is not accessible directly from outside the createCounter
function, providing a form of data privacy.
Event Handlers
Closures are commonly used in event handlers to maintain state.
for (var i = 0; i < 3; i++) {
(function(index) {
document.getElementById('button' + index).addEventListener('click', function() {
console.log('Button ' + index + ' clicked');
});
})(i);
}
Each event handler is a closure that captures the current value of i
.
Function Factories
Closures can be used to create functions dynamically.
function createMultiplier(multiplier) {
return function(x) {
return x * multiplier;
};
}
const double = createMultiplier(2);
console.log(double(5)); // Outputs: 10
The createMultiplier
function generates new functions that multiply a number by a specified multiplier.
Closures and Memory Management
While closures are powerful, they can lead to memory leaks if not managed carefully. Since closures retain references to outer variables, these variables are not garbage collected as long as the closure exists.
Example of a Potential Memory Leak
function attachData(data) {
const element = document.getElementById('myElement');
element.onclick = function() {
console.log('Data:', data);
};
}
If the element is removed from the DOM, the closure created by the onclick
handler still retains a reference to element
, preventing it from being garbage collected.
Advanced Closure Concepts
IIFE and Closures
Immediately Invoked Function Expressions (IIFE) often use closures to create private scopes.
(function() {
var privateVar = 'Secret';
window.myFunction = function() {
console.log(privateVar); // Accesses privateVar
};
})();
Closures in Asynchronous Programming
Closures play a crucial role in asynchronous programming, especially in callbacks and promises.
function fetchData(callback) {
// Simulate async data fetch
setTimeout(function() {
callback('Data loaded');
}, 1000);
}
function onDataLoaded(data) {
console.log(data); // Closure accessing 'data'
}
fetchData(onDataLoaded);
In this example, onDataLoaded
is a closure used as a callback in an asynchronous operation.
Closures are a central concept in JavaScript, offering powerful capabilities for managing scope, data privacy, and creating more dynamic, functional code.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
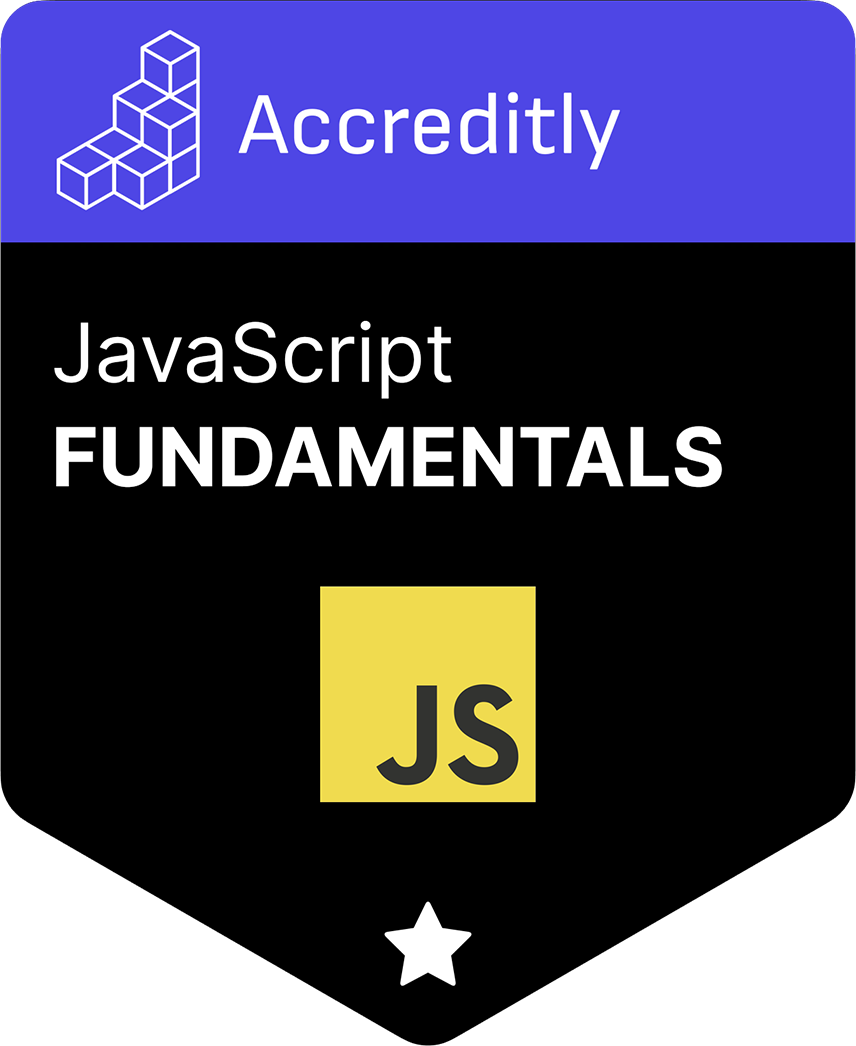