- Understanding the Filter Method
- Simple Usage of the Filter Method
- Advanced Usage of the Filter Method
- Chaining Filter with Other Array Methods
- Performance Considerations
- filter(), done.
The JavaScript array filter()
method is an integral part of a JavaScript developer's toolkit, yet it's often underused or misunderstood. It provides a robust, concise, and declarative way to filter elements in an array, transforming complex loops into single, readable lines of code. This article presents a comprehensive look at the JavaScript filter()
method and explores how it can be utilized effectively in your code.
Already comfortable with filter
? You're probably a good candidate for our JavaScript Fundamentals certification.
Understanding the Filter Method
The filter()
method creates a new array with all elements that pass the test implemented by the provided function. The original array remains unmodified.
Here's the basic syntax:
let newArray = arr.filter(callback(element[, index[, array]])[, thisArg])
The callback
function is invoked with three arguments:
-
element
: The current element being processed in the array. -
index
(optional): The index of the current element being processed in the array. -
array
(optional): The arrayfilter
was called upon.
The callback
function should return true
for elements that should be in the new array and false
otherwise.
Simple Usage of the Filter Method
Let's say you have an array of numbers and you want to filter out the even numbers. Here's how you can achieve that:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
let evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // [2, 4, 6, 8]
This function makes use of the modulus/remainer operator (%
) to ensure there are no remaining numbers when dividing by 2, this is an easy way to check whether a number is odd or even.
Advanced Usage of the Filter Method
The filter()
method can be used with objects as well. Suppose we have an array of users and we want to filter users who are older than 18.
let users = [
{ name: 'Alice', age: 20 },
{ name: 'Bob', age: 15 },
{ name: 'Charlie', age: 25 },
{ name: 'Dave', age: 16 }
];
let adults = users.filter(user => user.age > 18);
console.log(adults);
// [{ name: 'Alice', age: 20 }, { name: 'Charlie', age: 25 }]
The filter itself here is quite straightforward, but you can see how you can use it to access object keys and values.
Chaining Filter with Other Array Methods
One of the powerful features of the filter()
method (and many other array methods) is that you can chain them together. For example, you might want to filter an array and then map the filtered elements to a new form:
let users = [
{ name: 'Alice', age: 20 },
{ name: 'Bob', age: 15 },
{ name: 'Charlie', age: 25 },
{ name: 'Dave', age: 16 }
];
let adultNames = users
.filter(user => user.age > 18)
.map(user => user.name);
console.log(adultNames); // ['Alice', 'Charlie']
In the above example, we first filter out the adults and then map them to an array of names.
Performance Considerations
While filter()
is a powerful method, it's not always the most efficient. The filter()
function traverses the entire array, even if it has already found the elements you wanted. If you only need the first element that matches your criteria, consider using find()
instead.
filter()
, done.
Mastering the JavaScript filter()
method can greatly improve your code quality, making it more readable, maintainable, and declarative. By understanding its usage and features, you can leverage filter()
to its full potential.
Remember, like any tool, filter()
is not always the best fit for every situation. Always consider the requirements and performance implications before deciding which JavaScript method to use.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
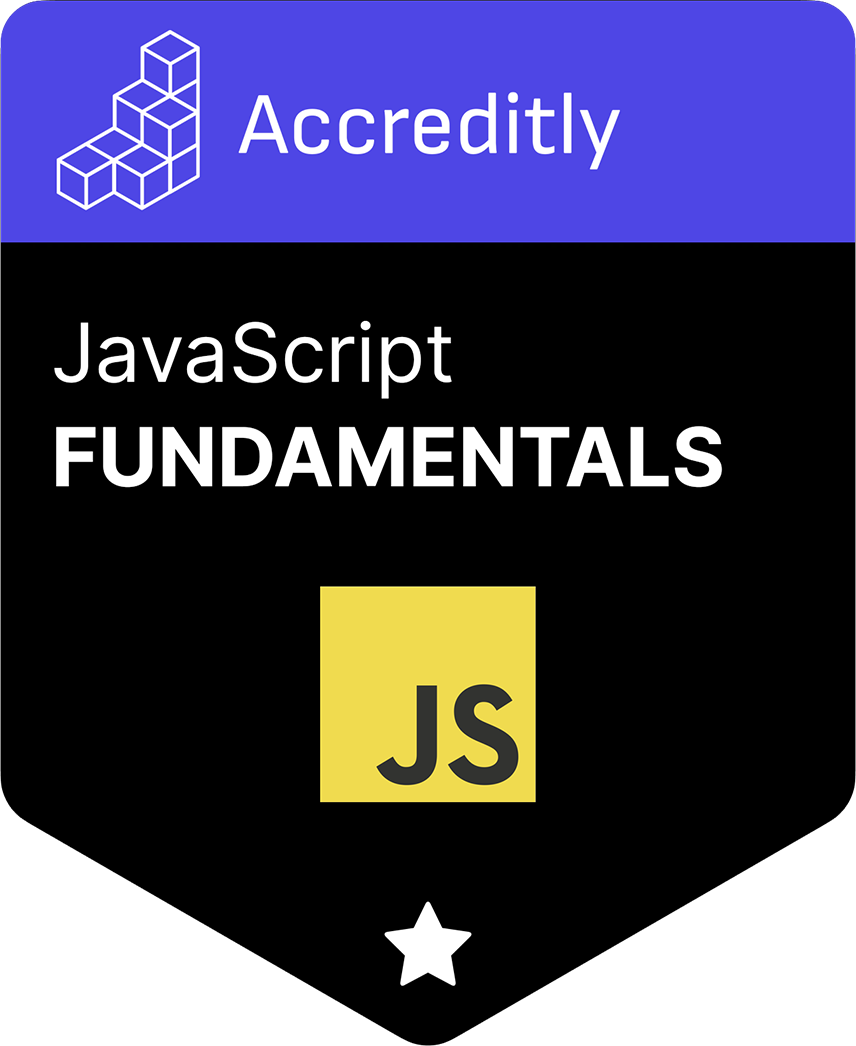