- Understanding requestIdleCallback
- How Does requestIdleCallback Work?
- Using requestIdleCallback Effectively
- Limitations and Considerations
In the quest for optimal web performance, efficiently managing tasks without hampering the user experience is crucial. JavaScript offers a multitude of ways to handle asynchronous operations and timed events. Among these, requestIdleCallback
stands out as a powerful yet lesser-known function designed to run background tasks during idle periods. This article delves into the mechanics, uses, and nuances of requestIdleCallback
, providing insights into enhancing your web application's performance.
requestIdleCallback
Understanding The requestIdleCallback
API allows developers to schedule background tasks that run during the browser's idle periods, ensuring high-priority tasks like animations and user inputs are not delayed. It's a way to take advantage of the browser's downtime, improving the site's responsiveness and performance by deferring non-essential tasks.
MDN Documentation on requestIdleCallback
requestIdleCallback
Work?
How Does When you invoke requestIdleCallback
, you pass it a callback function that the browser will call during its idle periods. The browser also provides a deadline object, indicating how much time is available before it needs to take control back, typically for tasks like rendering.
window.requestIdleCallback((deadline) => {
while (deadline.timeRemaining() > 0 && tasks.length > 0) {
doWorkIfNeeded();
}
});
This mechanism allows your web application to stay responsive by ensuring that heavy tasks don't block the main thread when the user requires immediate feedback from the UI.
requestIdleCallback
Effectively
Using Task Scheduling:
The primary use case for requestIdleCallback
is scheduling tasks that are not time-critical. Examples include saving application state, pre-fetching resources for later use, or performing heavy calculations for non-immediate features.
Debouncing and Throttling:
While requestIdleCallback
is not a direct replacement for debouncing or throttling, it can be used in conjunction to reduce the load of repetitive tasks triggered by user actions, like resizing windows or scrolling.
Fallback for Unsupported Browsers:
Not all browsers support requestIdleCallback
. For those that don’t, you can create a simple fallback to setTimeout
:
window.requestIdleCallback = window.requestIdleCallback || function (cb) {
return setTimeout(() => {
var start = Date.now();
cb({
didTimeout: false,
timeRemaining: function () {
return Math.max(0, 50 - (Date.now() - start));
}
});
}, 1);
};
Limitations and Considerations
While requestIdleCallback
is a potent tool, there are limitations and best practices to consider:
- As of now, not all browsers support
requestIdleCallback
. Ensure you implement a fallback mechanism or feature detection to maintain functionality across browsers. - The browser may not always find an idle period to run your callback, especially under heavy load. Critical tasks should not rely solely on
requestIdleCallback
. - Overuse or misuse can lead to jank or delayed execution of tasks. It's best utilized for enhancing performance rather than as a primary mechanism for task management.
requestIdleCallback
offers a sophisticated way to improve your web application's performance by smartly utilizing the browser's idle times. By understanding its operation, benefits, and limitations, developers can enhance user experience without sacrificing responsiveness. As web technologies evolve, leveraging such browser APIs to optimize performance while maintaining smooth interaction will be key to building efficient, modern web applications. Remember to test across browsers and conditions to ensure your usage of requestIdleCallback
delivers the intended benefits without unforeseen drawbacks.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
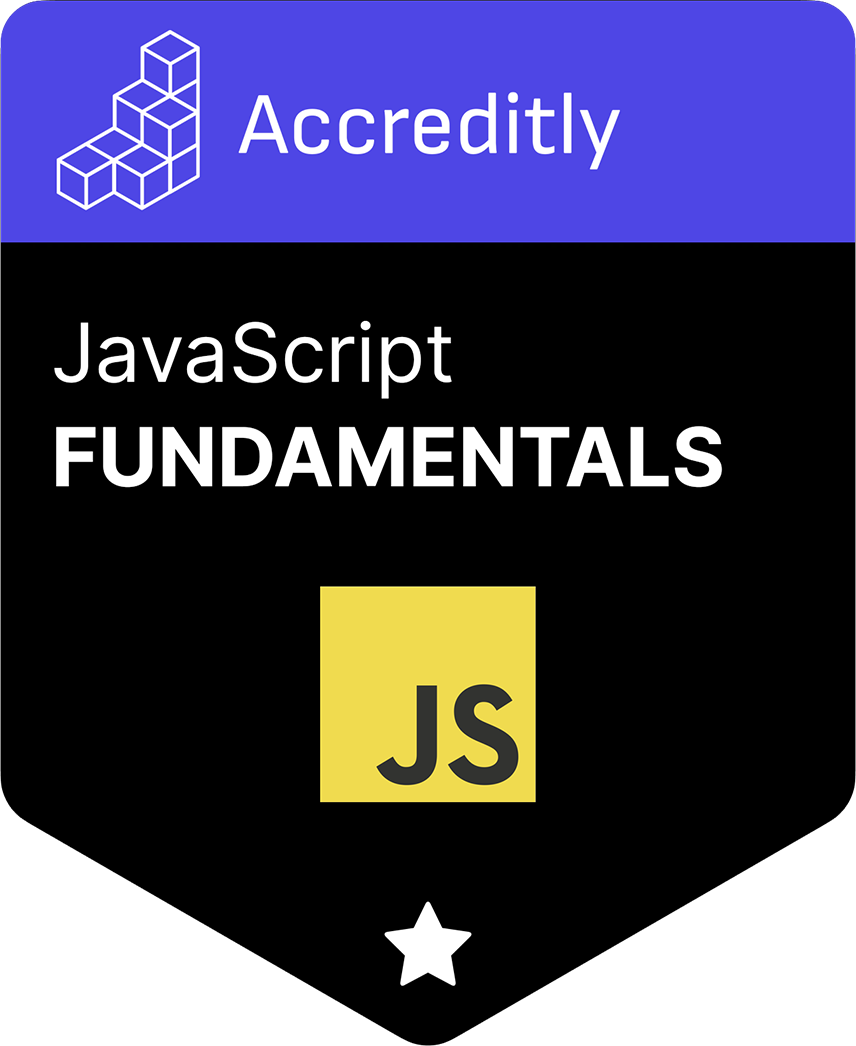