- 1. What is a Form Request?
- 2. Benefits of Using Form Requests
- 3. Creating a Form Request
- 4. Implementing Validation Rules
- 5. Authorization Logic
- 6. Custom Error Messages
- 7. Using Form Requests in Controllers
- 8. Conclusion
Laravel, a prominent PHP framework, has consistently been at the forefront of web development due to its robust features. One such feature is the Form Request, which provides an intuitive approach to handle validation and authorization for form submissions. In this guide, we'll dive deep into Form Requests, covering its benefits, implementation, and best practices.
1. What is a Form Request?
In Laravel, a Form Request is a custom request class that contains validation logic. It's a way to encapsulate validation rules, error messages, and even authorization logic in a dedicated class, rather than writing this logic directly in controllers.
2. Benefits of Using Form Requests
-
Cleaner Controllers: By moving validation logic out of controllers, they become more concise and focused on their primary task.
-
Reusable Validation: Form Requests can be reused across different controllers and methods, promoting the DRY (Don't Repeat Yourself) principle.
-
Centralized Authorization: With Form Requests, you can also handle authorization, ensuring that the incoming request is allowed by the authenticated user.
3. Creating a Form Request
To generate a new Form Request, use the make:request
Artisan command:
php artisan make:request StoreBlogPost
This will create a new request file in app/Http/Requests/StoreBlogPost.php
.
4. Implementing Validation Rules
Within the generated Form Request, you'll find two methods: authorize()
and rules()
.
In the rules()
method, return an array of validation rules:
public function rules() {
return [
'title' => 'required|unique:posts|max:255',
'body' => 'required',
];
}
5. Authorization Logic
The authorize()
method is where you can add any authorization logic. Return true
if the user is authorized, and false
otherwise:
public function authorize() {
return true; // for demonstration purposes. Typically, you'd include some logic here.
}
6. Custom Error Messages
You can override the default error messages by defining a messages()
method:
public function messages() {
return [
'title.required' => 'A title is required',
'body.required' => 'A message is required',
];
}
7. Using Form Requests in Controllers
To use the Form Request in a controller, type-hint it in the method where you want to apply validation:
public function store(StoreBlogPost $request) {
// The incoming request is valid...
// Retrieve the validated input data...
$validated = $request->validated();
}
Laravel will automatically validate the incoming request against the rules defined in the Form Request and will redirect the user back if validation fails.
8. Conclusion
Form Requests in Laravel provide a centralized and organized method to handle both validation and authorization, ensuring that your application's data integrity is maintained and only authorized users can perform certain actions. By leveraging this feature, developers can write cleaner, more maintainable, and more secure code.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
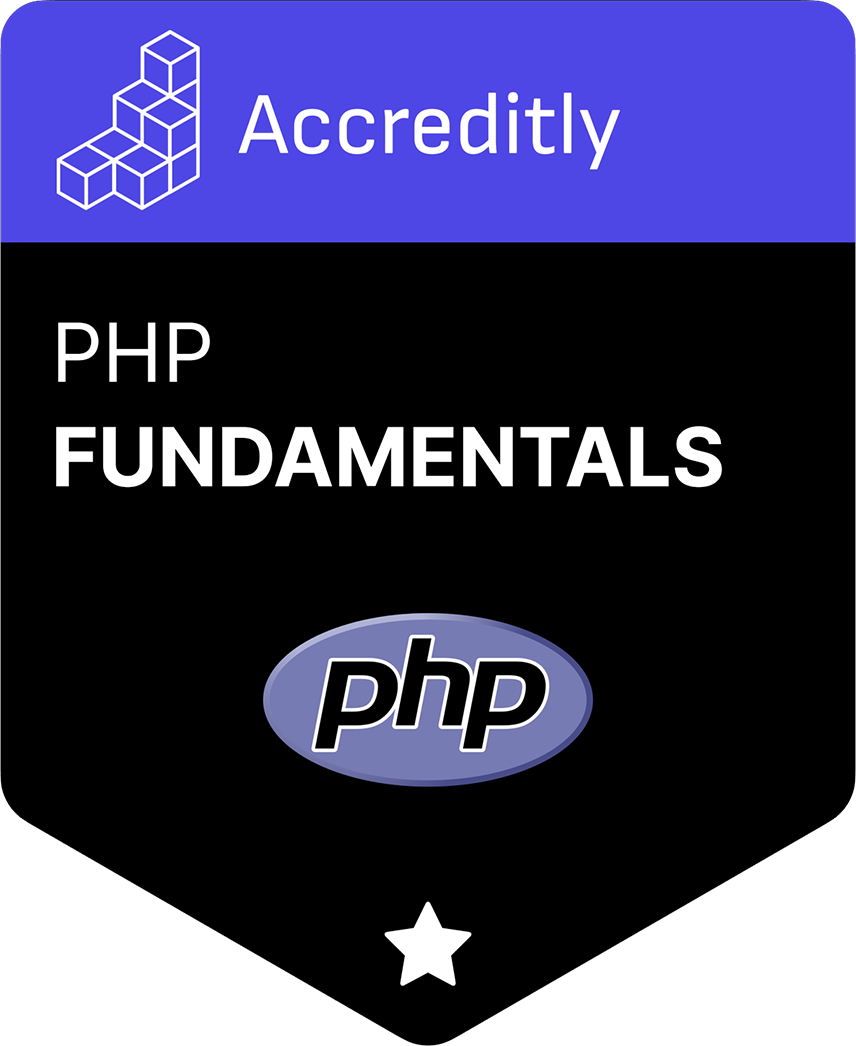