Laravel Livewire offers a unique and robust approach to modern full-stack web development. One of its key features is its event-driven components, providing a system of inter-component communication. This system is primarily based on Livewire's various emit functions, namely emit
, emitSelf
, and emitUp
.
In this article, we will dive deep into these emit functions, exploring how they work, their differences, and when to use each. We'll accompany each explanation with practical code examples for a better understanding.
Laravel Livewire's Event System
Before we delve into the specifics of each function, it's essential to understand Livewire's event system. Similar to front-end frameworks like Vue.js or React.js, Livewire uses an event-driven architecture. This means components communicate by emitting and listening to events, ensuring a decoupled yet interactive user interface.
emit
Function
The emit
is the primary event emission function in Laravel Livewire. It allows a component to broadcast an event, which other components (including itself) can listen to and respond.
Here's an example:
class ParentComponent extends Component
{
protected $listeners = ['childUpdated' => 'handleChildUpdated'];
public function handleChildUpdated($data)
{
// Handle the event...
}
// ...
}
class ChildComponent extends Component
{
public function update()
{
// Perform some updates...
$this->emit('childUpdated', $data);
}
// ...
}
In the example above, when the update
method in the ChildComponent
is called, it emits a childUpdated
event. The ParentComponent
listens for this event and calls the handleChildUpdated
method when it is fired.
emitSelf
Function
The While emit broadcasts the event to all listeners, emitSelf
restricts this emission to the component instance that fired the event. This function is useful when you need to trigger a re-render of the component or need to call an action within the same component.
Here's how you can use it:
class ExampleComponent extends Component
{
public function doSomething()
{
// Perform some actions...
$this->emitSelf('doSomethingElse');
}
public function doSomethingElse()
{
// This will be called...
}
// ...
}
emitUp
Function
The emitUp
is a directional emit function. It broadcasts an event up the component tree. It's useful in nested component situations when a child component needs to communicate an event to its parent component.
Here's an example:
class GrandParentComponent extends Component
{
protected $listeners = ['childUpdated' => 'handleChildUpdated'];
public function handleChildUpdated($data)
{
// Handle the event...
}
// ...
}
class ParentComponent extends Component
{
// ...
}
class ChildComponent extends Component
{
public function update()
{
// Perform some updates...
$this->emitUp('childUpdated', $data);
}
// ...
}
In this example, when the ChildComponent
emits the childUpdated
event, only the GrandParentComponent
will handle it. The ParentComponent
in between does not get involved.
Summary
Understanding these emit functions is vital for mastering Laravel Livewire's event-driven system. They offer a way to create loosely coupled yet highly interactive components, making your application more scalable and maintainable.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
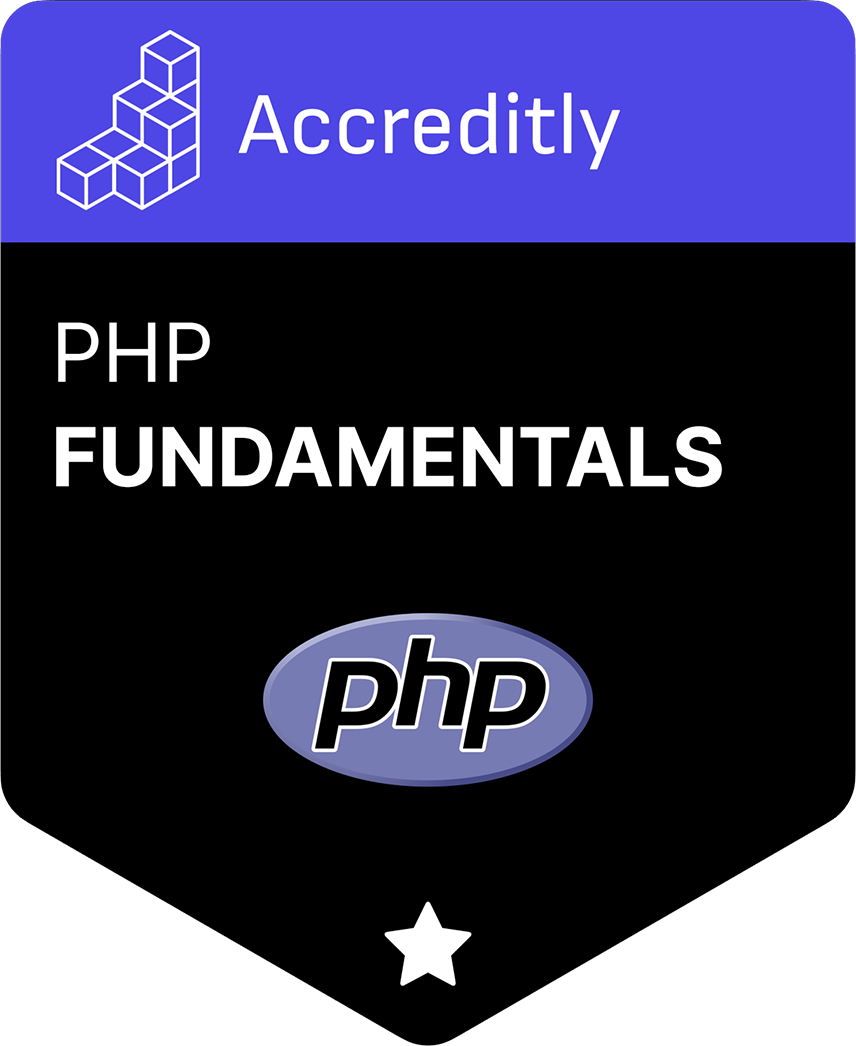