- Why Follow Coding Standards?
- PHP Coding Standards
- HTML Coding Standards
- CSS Coding Standards
- JavaScript Coding Standards
- SQL Coding Standards
- Best Practices and Additional Resources
- Conclusion: Mastering WordPress Coding Standards
- PHP Coding Standards Deep Dive
- HTML Coding Standards Deep Dive
- CSS Coding Standards Deep Dive
- JavaScript Coding Standards Deep Dive
- SQL Coding Standards Deep Dive
- Final Thoughts: Crafting Quality Code
WordPress, as one of the most popular content management systems (CMS), has established a set of coding standards and conventions to ensure consistency, readability, and maintainability of its codebase. These standards are crucial for anyone looking to contribute to WordPress or develop themes and plugins that integrate seamlessly with the platform. In this article, we will delve deeply into the WordPress coding standards, covering PHP, HTML, CSS, JavaScript, and SQL, providing numerous examples to illustrate these principles.
Why Follow Coding Standards?
Adhering to coding standards offers several benefits:
- Consistency: Ensures that code looks the same, making it easier to read and maintain.
- Collaboration: Facilitates collaboration among developers by providing a common set of rules.
- Quality: Improves the overall quality and security of the code.
- Debugging: Makes it easier to spot and fix bugs.
For an in-depth look at WordPress coding standards, refer to the official WordPress Coding Standards.
PHP Coding Standards
WordPress is primarily written in PHP, so adhering to PHP coding standards is critical. Here are some key aspects:
1. File Formatting
-
Opening PHP Tag: Always use the
<?php
tag. - File Encoding: UTF-8 without BOM.
- Indentation: Use tabs, not spaces.
- Line Endings: Unix-style (LF).
<?php
// Correct PHP opening tag and indentation
echo 'Hello, WordPress!';
?>
2. Naming Conventions
- Variables: Use lowercase letters and underscores.
- Functions: Use lowercase letters and underscores.
-
Classes: Use
CamelCase
. - Constants: Use uppercase letters and underscores.
<?php
// Variable
$variable_name = 'value';
// Function
function function_name() {
return true;
}
// Class
class ClassName {
const CONSTANT_NAME = 'constant';
}
?>
3. Control Structures
- Spacing: One space between control structure keyword and opening parenthesis.
- Braces: Opening brace on the same line, closing brace on a new line.
<?php
// If-else statement
if ( true ) {
echo 'This is true';
} else {
echo 'This is false';
}
// For loop
for ( $i = 0; $i < 10; $i++ ) {
echo $i;
}
?>
4. Function Calls
- Spacing: No space between function name and opening parenthesis.
- Arguments: Separate with a single space after the comma.
<?php
// Correct function call
my_function( $arg1, $arg2 );
?>
HTML Coding Standards
HTML is the backbone of WordPress themes and plugins. Consistent HTML helps maintain a clean and accessible codebase.
1. Doctype
Always use the HTML5 doctype.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
</body>
</html>
2. Indentation
Use tabs for indentation, aligning nested elements properly.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div>
<p>Hello, World!</p>
</div>
</body>
</html>
3. Attribute Formatting
Use lowercase for attribute names, and always use quotes around attribute values.
<a href="https://example.com" title="Example">Link</a>
4. Self-closing Tags
Self-closing tags should not have a closing slash.
<img src="image.jpg" alt="Image description">
CSS Coding Standards
CSS is crucial for styling WordPress themes. Following a consistent style guide ensures that styles are maintainable and scalable.
1. File Formatting
- Encoding: UTF-8 without BOM.
- Indentation: Use tabs.
2. Selectors
- Use lowercase and hyphens for class and ID names.
/* Correct class and ID naming */
.class-name {
color: blue;
}
#id-name {
color: red;
}
3. Properties
- One property per line, ending with a semicolon.
- One space after the colon.
/* Correct property formatting */
.class-name {
color: blue;
background-color: #fff;
font-size: 16px;
}
4. Comments
- Use comments to explain the purpose of a section or complex piece of code.
/* Primary Button Styles */
.button-primary {
background-color: #0073aa;
color: #fff;
padding: 10px 20px;
border-radius: 5px;
}
JavaScript Coding Standards
JavaScript is used extensively in WordPress for dynamic functionality. Adhering to coding standards ensures that scripts are clean and efficient.
1. File Formatting
- Encoding: UTF-8 without BOM.
- Indentation: Use tabs.
2. Variable Naming
- Use camelCase for variables and functions.
// Correct variable and function naming
var myVariable = 'value';
function myFunction() {
return true;
}
3. Control Structures
- Same principles as PHP: one space between keyword and parenthesis, braces on the same line.
// Correct if-else structure
if ( true ) {
console.log('This is true');
} else {
console.log('This is false');
}
4. Function Declarations
- Named functions and anonymous functions should follow the same spacing and formatting rules.
// Named function
function namedFunction() {
return true;
}
// Anonymous function
var anonymousFunction = function() {
return true;
};
5. Comments
- Use single-line comments (
//
) and multi-line comments (/* ... */
) appropriately.
// Single-line comment
/*
Multi-line comment
explaining a section of code
*/
SQL Coding Standards
SQL is often used in WordPress for database interactions. Proper formatting ensures readability and reduces the risk of SQL injection.
1. Keywords
- Use uppercase for SQL keywords.
SELECT * FROM wp_posts WHERE post_status = 'publish';
2. Table and Column Names
- Use lowercase with underscores.
SELECT post_title, post_date FROM wp_posts;
3. Indentation
- Indent code within SQL statements for readability.
SELECT post_title, post_date
FROM wp_posts
WHERE post_status = 'publish'
ORDER BY post_date DESC;
Best Practices and Additional Resources
Adhering to WordPress coding standards is a fundamental step in ensuring that your code is clean, readable, and maintainable. Beyond the basic standards, here are some best practices to consider:
1. Security
Always sanitize and validate user input to prevent security vulnerabilities like SQL injection and XSS.
// Sanitizing and validating user input
$input = sanitize_text_field( $_POST['input_field'] );
if ( !empty( $input ) && is_string( $input ) ) {
// Proceed with safe input
}
2. Performance
Optimize your code for performance by minimizing database queries, using caching, and avoiding unnecessary computations.
// Example of caching in WordPress
$transient_key = 'my_custom_data';
$custom_data = get_transient( $transient_key );
if ( false === $custom_data ) {
// Data not found in cache, perform the expensive operation
$custom_data = expensive_operation();
// Store the result in cache for 12 hours
set_transient( $transient_key, $custom_data, 12 * HOUR_IN_SECONDS );
}
// Use the cached data
3. Documentation
Document your code using comments and docblocks to make it easier for others (and yourself) to understand and maintain.
/**
* Calculate the sum of two numbers.
*
* @param int $a First number.
* @param int $b Second number.
* @return int The sum of the two numbers.
*/
function calculate_sum( $a, $b ) {
return $a + $b;
}
Conclusion: Mastering WordPress Coding Standards
Understanding and adhering to WordPress coding standards is essential for any developer aiming to create high-quality themes, plugins, or contributions to the WordPress core. By following these guidelines, you ensure that your code is consistent, readable, and maintainable, facilitating collaboration and improving the overall quality of your work.
For further reading and reference, consult the following resources:
- WordPress PHP Coding Standards
- WordPress HTML Coding Standards
- WordPress CSS Coding Standards
- WordPress JavaScript Coding Standards
- WordPress SQL Coding Standards
By integrating these standards into your workflow, you’ll be well on your way to becoming a proficient and respected WordPress developer.
PHP Coding Standards Deep Dive
Next, let's take a deeper dive into PHP coding standards, which form the backbone of WordPress development.
1. PHP Tags
Always start your PHP files with <?php
and avoid using the shorthand <?
or <?=
.
<?php
// Correct
echo 'Hello, World!';
// Incorrect
echo 'Hello, World!';
2. Indentation
WordPress uses tabs for indentation, not spaces. This ensures that the code is uniformly indented across different environments.
<?php
// Correct
if ( true ) {
echo 'Hello, World!';
}
// Incorrect
if ( true ) {
echo 'Hello, World!';
}
?>
3. Line Endings
Use Unix-style line endings (LF
) for consistency across different operating systems. Most modern code editors can be configured to enforce this.
4. Naming Conventions
- Variables: Use all lowercase letters and underscores to separate words.
<?php
// Correct
$my_variable = 'value';
// Incorrect
$myVariable = 'value';
$MyVariable = 'value';
?>
- Functions: Follow the same convention as variables.
<?php
// Correct
function my_function_name() {
// Function body
}
// Incorrect
function myFunctionName() {
// Function body
}
?>
-
Classes: Use
CamelCase
, starting with an uppercase letter.
<?php
// Correct
class MyClassName {
// Class body
}
// Incorrect
class myclassname {
// Class body
}
?>
- Constants: Use all uppercase letters with underscores.
<?php
// Correct
define( 'MY_CONSTANT', 'value' );
// Incorrect
define( 'myConstant', 'value' );
?>
5. Control Structures
Ensure there is a single space after the control structure keyword and before the opening parenthesis. Braces should be placed on the same line as the control structure, with the closing brace on a new line.
<?php
// Correct
if ( $condition ) {
// Code
} elseif ( $another_condition ) {
// Code
} else {
// Code
}
// Incorrect
if($condition)
{
// Code
}
elseif($another_condition)
{
// Code
}
else
{
// Code
}
?>
6. Function Calls
When calling a function, do not put a space between the function name and the opening parenthesis. However, separate arguments with a single space after the comma.
<?php
// Correct
my_function( $arg1, $arg2 );
// Incorrect
my_function ( $arg1 , $arg2 );
?>
7. Function Definitions
In function definitions, place the opening brace on the same line as the function name, with the closing brace on a new line.
<?php
// Correct
function my_function( $arg1, $arg2 ) {
// Function body
}
// Incorrect
function my_function( $arg1, $arg2 )
{
// Function body
}
?>
8. Arrays
Use the shorthand array syntax []
instead of array()
and align array items for readability.
<?php
// Correct
$array = [
'key1' => 'value1',
'key2' => 'value2',
];
// Incorrect
$array = array(
'key1' => 'value1',
'key2' => 'value2'
);
?>
9. Comments
Use single-line comments (//
) for short explanations and multi-line comments (/* ... */
) for longer explanations. DocBlocks (/** ... */
) should be used for documenting functions, classes, and methods.
<?php
// Single-line comment
/*
Multi-line comment
explaining a section of code
*/
/**
* Function documentation
*
* @param string $param Description.
* @return void
*/
function my_function( $param ) {
// Function body
}
?>
By following these PHP coding standards, you ensure that your code is consistent, readable, and maintainable, aligning with the broader WordPress development community's expectations.
HTML Coding Standards Deep Dive
HTML is the foundation of web content, and consistent HTML coding practices are essential for creating clean and maintainable web pages.
1. Doctype Declaration
Always start your HTML documents with the HTML5 doctype declaration to ensure standards compliance.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
</body>
</html>
2. Indentation and Nesting
Use tabs for indentation and properly nest elements to reflect the document structure.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
<main>
<article>
<h2>Article Title</h2>
<p>This is an article paragraph.</p>
</article>
</main>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
3. Attribute Quotation
Always use double quotes for attribute values and include attributes for elements.
<a href="https://example.com" title="Example">Visit Example</a>
4. Self-closing Tags
Self-closing tags should not include a closing slash.
<!-- Correct -->
<img src="image.jpg" alt="Description">
<!-- Incorrect -->
<img src="image.jpg" alt="Description" />
5. Semantic HTML
Use semantic HTML elements to enhance accessibility and SEO.
<!-- Correct -->
<article>
<header>
<h1>Article Title</h1>
</header>
<p>Article content.</p>
<footer>
<p>Written by Author</p>
</footer>
</article>
<!-- Incorrect -->
<div class="article">
<div class="header">
<h1>Article Title</h1>
</div>
<p>Article content.</p>
<div class="footer">
<p>Written by Author</p>
</div>
</div>
CSS Coding Standards Deep Dive
Consistent CSS coding practices are crucial for maintaining the visual consistency and usability of a website.
1. File Formatting and Encoding
Use UTF-8 encoding without BOM for CSS files. Use tabs for indentation.
2. Selectors and Properties
Use meaningful, lowercase selectors with hyphens. Align properties for readability.
/* Correct */
.header {
background-color: #f5f5f5;
padding: 20px;
}
/* Incorrect */
.Header {
background-color:#f5f5f5;padding:20px;
}
3. Colors
Use hexadecimal notation for colors, preferring shorthand where applicable.
/* Correct */
.color {
color: #fff;
background-color: #000;
}
/* Incorrect */
.color {
color: white;
background-color: black;
}
4. Comments
Use comments to explain complex sections of CSS, using a consistent comment style.
/* Primary Navigation */
.nav {
display: flex;
justify-content: space-between;
}
/* Navigation links */
.nav a {
color: #0073aa;
text-decoration: none;
}
JavaScript Coding Standards Deep Dive
JavaScript is essential for adding interactivity to WordPress sites. Consistent coding practices help maintain clarity and functionality.
1. File Formatting and Encoding
Use UTF-8 encoding without BOM for JavaScript files. Use tabs for indentation.
2. Variable and Function Naming
Use camelCase for variable and function names.
// Correct
var myVariable = 'value';
function myFunction() {
return true;
}
// Incorrect
var my_variable = 'value';
function my_function() {
return true;
}
3. Control Structures
Follow consistent formatting for control structures, using a single space after the keyword and placing braces correctly.
// Correct
if ( condition ) {
doSomething();
} else {
doSomethingElse();
}
// Incorrect
if(condition){
doSomething();
}else{
doSomethingElse();
}
4. Comments
Use single-line comments for brief explanations and multi-line comments for longer descriptions.
// Single-line comment
var myVariable = 'value';
/*
Multi-line comment
explaining a section of code
*/
function myFunction() {
return true;
}
SQL Coding Standards Deep Dive
SQL is used for database interactions in WordPress. Consistent SQL coding practices ensure efficient and secure database queries.
1. Keywords
Use uppercase for SQL keywords to distinguish them from table and column names.
-- Correct
SELECT * FROM wp_posts WHERE post_status = 'publish';
-- Incorrect
select * from wp_posts where post_status = 'publish
';
2. Table and Column Names
Use lowercase with underscores for table and column names.
-- Correct
SELECT post_title, post_date FROM wp_posts;
-- Incorrect
SELECT PostTitle, PostDate FROM wpPosts;
3. Indentation and Formatting
Indent code within SQL statements for readability, especially for complex queries.
-- Correct
SELECT post_title, post_date
FROM wp_posts
WHERE post_status = 'publish'
ORDER BY post_date DESC;
-- Incorrect
SELECT post_title, post_date FROM wp_posts WHERE post_status = 'publish' ORDER BY post_date DESC;
4. Comments
Use comments to explain complex queries or sections of SQL code.
-- Retrieve all published posts
SELECT post_title, post_date
FROM wp_posts
WHERE post_status = 'publish'
ORDER BY post_date DESC;
Final Thoughts: Crafting Quality Code
Adhering to coding standards is a cornerstone of professional WordPress development. It ensures that your code is not only functional but also maintainable, readable, and secure. By following these guidelines and incorporating best practices, you contribute to a higher standard of quality in the WordPress community.
For further reading and comprehensive guidelines, explore these resources:
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
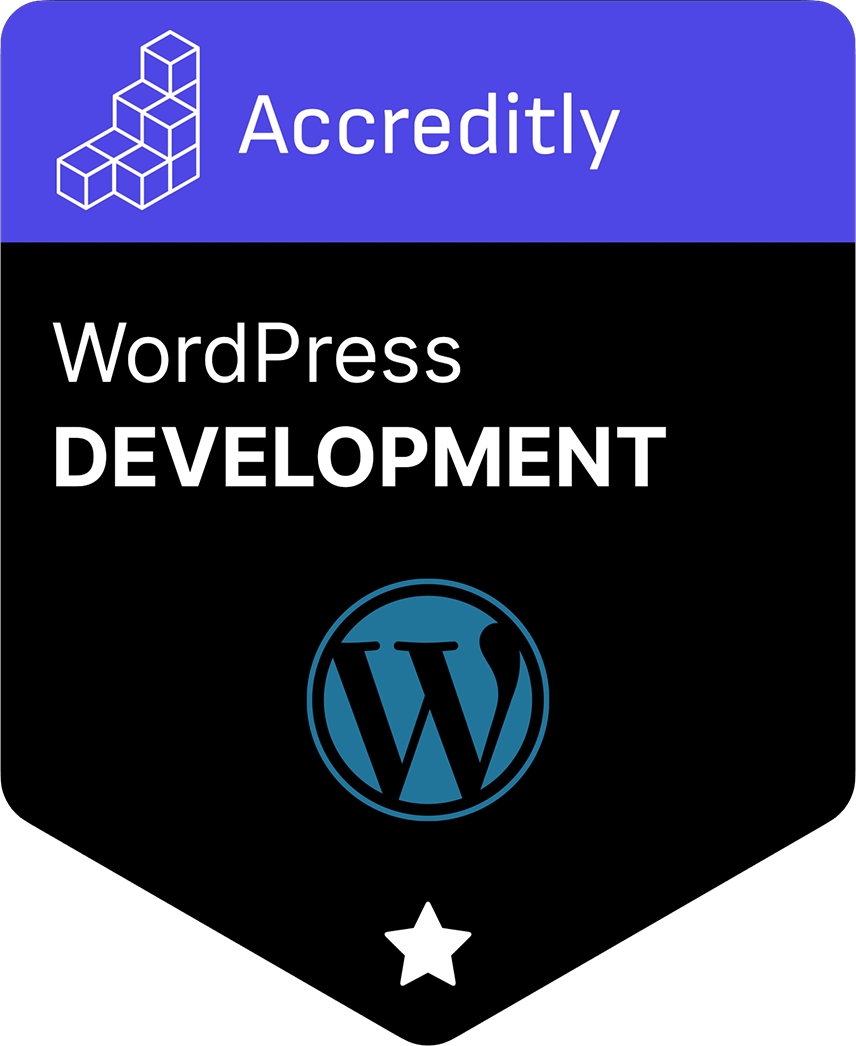