Learning a new programming language can sometimes feel like learning a foreign language. With JavaScript, this feeling is compounded by the multitude of terms that are thrown around in tutorials, forums, and stack traces. This glossary aims to help JavaScript developers, both newcomers and seasoned veterans, understand the myriad of terms that pervade the JavaScript ecosystem.
A
Array: An ordered list of values that can be of any type. In JavaScript, arrays can be created using brackets []
. JavaScript arrays are zero-indexed, meaning the first element is accessed with the index 0. Read more in the MDN Documentation.
Arrow Function: Introduced in ES6, arrow functions offer a shorter syntax for writing function expressions. They are anonymous functions that use the =>
syntax. They also have lexical this
value. Read more in the MDN Documentation.
Async/Await: A syntax sugar over Promises in JavaScript that makes asynchronous code look and behave like synchronous code. async
is used to declare an asynchronous function and await
is used to wait for a Promise. Read more in the MDN Documentation.
B
Boolean: A primitive data type in JavaScript that can hold two values: true
or false
. Often used in logical operations and comparisons. Read more in the MDN Documentation.
C
Callback: A function that is passed to another function as an argument and is expected to execute at a given time. This is a core concept related to asynchronous operations in JavaScript. Read more in the MDN Documentation.
Class: Introduced in ES6, classes are a template for creating objects. They encapsulate data with code. JavaScript classes are primarily syntactical sugar over JavaScript's existing prototype-based inheritance. Read more in the MDN Documentation.
Closure: A JavaScript feature where an inner function has access to the outer (enclosing) function's variables and parameters, even after the outer function has returned. Read more in the MDN Documentation.
Constructor: A special method used to create and initialize an object created within a class. Read more in the MDN Documentation.
Console: A built-in JavaScript object that provides access to the browser's debugging console. The specific syntax depends on your browser or operating system. Read more in the MDN Documentation.
D
DOM (Document Object Model): The data representation of the objects that comprise the structure and content of a document on the web. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects. Read more in the MDN Documentation.
E
Event Listener: A procedure in JavaScript that waits for an event to occur. The simple idea of "listen, then act" is more complex in JavaScript than you might initially think. Read more in the MDN Documentation.
ES6/ES2015: A major update to JavaScript that includes dozens of new features. With ES6, you can write object-oriented code, organize your code into modules, and replace callbacks with promises. Read more in the MDN Documentation.
Expression: In JavaScript, an expression is a piece of code that produces a value. Expressions are different from statements in that statements do something while expressions produce a value. Read more in the MDN Documentation.
F
Fetch API: A modern interface that allows you to make HTTP requests to servers from web browsers. This is a built-in browser API that was introduced to make HTTP requests (replacing the need for the XMLHttpRequest API). Read more in the MDN Documentation.
Function: A reusable set of instructions. Functions in JavaScript are objects, a special kind of objects. Functions can be assigned to variables, stored in an object, or passed as function arguments. Read more in the MDN Documentation.
Function Declaration: A function, defined using the function keyword. This is the traditional method used to declare a function in JavaScript. Read more in the MDN Documentation.
Function Expression: A function, defined inside an expression. You can store a function in a variable and then call the function using that variable. Function expressions are often stored in variables due to their result being a value. Read more in the MDN Documentation.
G
Generator: A type of function that can stop mid-way and then continue where it left off at any time. Generators are identified with an asterisk (*) after the function keyword and the yield keyword is used to pause and resume a generator function. Read more in the MDN Documentation.
Global Scope: Variables that are declared outside a function or simply declared without the var keyword become global variables. They can be accessed from any function throughout the web page. Read more in the MDN Documentation.
H
Hoisting: A JavaScript mechanism where variables and function declarations are moved to the top of their containing scope before code execution. Read more in the MDN Documentation.
I
Immediately-Invoked Function Expressions (IIFEs): A JavaScript function that runs as soon as it is defined. The syntax to define an IIFE is: (function() { /* code */ })()
. Read more in the MDN Documentation.
Inheritance: The concept of one thing gaining the properties or behaviors of something else. In JavaScript, inheritance is supported by using prototype chaining. Read more in the MDN Documentation.
Iteration: The act of repeating a process usually with the aim of approaching a desired goal or target. You can iterate over arrays and other iterable objects in JavaScript using loops and other methods like map and forEach. Read more in the MDN Documentation.
J
JSON (JavaScript Object Notation): A syntax for storing and exchanging data. It is often used when data is sent from a server to a web page. Read more in the MDN Documentation.
JWT (JSON Web Token): A compact, URL-safe means of representing claims to be transferred between two parties. JWTs encode claims to be transmitted as a JSON object, and they are protected by a digital signature. Read more in the JWT Documentation.
K
Key: In a key/value pair, the part that represents the identifier for the pair. Keys are used to access the corresponding values within the object. Read more in the MDN Documentation.
L
Local Scope: Variables that are declared inside a function or a block are local variables. They can only be accessed within that function or block. Read more in the MDN Documentation.
M
Map: A built-in JavaScript object that stores key-value pairs. Unlike objects, keys in Map can be of any data type. Read more in the MDN Documentation.
Method: A function which is a property of an object. It's a way to include behavior with data. Read more in the MDN Documentation.
Module: Small units of independent, reusable code that is desired to be used as the building blocks in creating a non-trivial Javascript application. Read more in the MDN Documentation.
N
Node: The generic name for any type of object in the Document Object Model (DOM), including elements, text and comments. Read more in the MDN Documentation.
Null: A built-in JavaScript type that has only one value, null
. It's often used to signify that a variable should have no value. Read more in the MDN Documentation.
Number: A numeric data type in JavaScript. It can be written with or without decimals. The Number object represents numerical date, either integers or floating-point numbers. Read more in the MDN Documentation.
O
Object: In JavaScript, an object is a standalone entity, with properties and type. It's a collection of properties, and a property is an association between a name (or key) and a value. Read more in the MDN Documentation.
Object-Oriented Programming (OOP): A programming paradigm based on the concept of "objects", which can contain data, in the form of fields (often known as attributes or properties), and code, in the form of procedures (often known as methods). Read more in the MDN Documentation.
Operator: Symbols that tell the JavaScript engine to perform some sort of mathematical, relational or logical operation. For example, +
is an arithmetic operator that adds two numbers and ===
is a comparison operator that checks equality. Read more in the MDN Documentation.
Option Object Pattern: A way to reduce the number of arguments to a function by making one argument be a JavaScript Object. It's also a way to emulate named parameters in JavaScript. Read more in the MDN Documentation.
P
Promise: A proxy for a value not necessarily known when the promise is created. It allows you to associate handlers with an asynchronous action's eventual success value or failure reason. Read more in the MDN Documentation.
Prototype: Prototypes are the mechanism by which JavaScript objects inherit features from one another. JavaScript’s prototype chain is similar to Ruby’s subclass chains, Python’s metaclasses, and Java’s class-based models. Read more in the MDN Documentation.
Property: A variable that is attached to an object. In JavaScript, objects are collections of key-value pairs. The keys are strings and the values are the JavaScript types. Keys are also called properties. Read more in the MDN Documentation.
R
Recursion: The process where a function calls itself directly or indirectly, in its body. Used for solving problems that can be broken up into easier sub-problems of the same type. Read more in the MDN Documentation.
Regular Expression (RegExp): Patterns used to match character combinations in strings. They are a powerful way to find and replace strings in JavaScript. Read more in the MDN Documentation.
Return: The statement that ends function execution and specifies a value to be returned to the function caller. Read more in the MDN Documentation.
S
Scope: Determines the visibility or accessibility of variables, functions, and objects in some particular part of your code during runtime. In other words, it defines the portion of the code where a variable or a function can be accessed. Read more in the MDN Documentation.
Set: A built-in JavaScript object that stores unique values. Unlike arrays, sets do not allow duplicate values. Read more in the MDN Documentation.
Strict Mode: A way to introduce better error-checking into your code. When you use strict mode, you cannot use implicitly declared variables, or assign a value to a read-only property, or add a property to an object that is not extensible. Read more in the MDN Documentation.
String: A series of characters. In JavaScript, string is a primitive data type and you can create a string using single or double quotes. Read more in the MDN Documentation.
T
Template Literals: Allows you to embed expressions into string literals, using ${expression}
. Template literals can be multi-line and include string interpolation. Read more in the MDN Documentation.
Truthy: A term used in JavaScript to describe a value that is considered true when encountered in a Boolean context. JavaScript uses type coercion in Boolean contexts. Read more in the MDN Documentation.
Type Coercion: The process of converting value from one type to another (such as string to number, object to boolean, and so on). Any operation involving an undefined or null value results in NaN or null respectively. Read more in the MDN Documentation.
U
Undefined: A primitive value automatically assigned to variables that have just been declared, or to formal arguments for which there are no actual arguments. Read more in the MDN Documentation.
URI/URL Encoding: A mechanism for encoding information in a URI under certain circumstances. Read more in the MDN Documentation.
Use Strict Directive: An expression that helps you catch common mistakes in your programs. It can help you write "safer" code by preventing the use of potentially harmful features in JavaScript. Read more in the MDN Documentation.
V
Value: The most basic unit of information in computing and programming. It's the data in its simplest form without any context. Read more in the MDN Documentation.
Variable: An "empty box" that you can store any value in to use later. In JavaScript, you create a variable with the let
, const
or var
keyword. Read more in the MDN Documentation.
Void Operator: The void operator evaluates the given expression and then returns undefined. Read more in the MDN Documentation.
This glossary is by no means exhaustive, but hopefully it helps you navigate through your JavaScript journey a little bit easier. Keep learning and coding!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
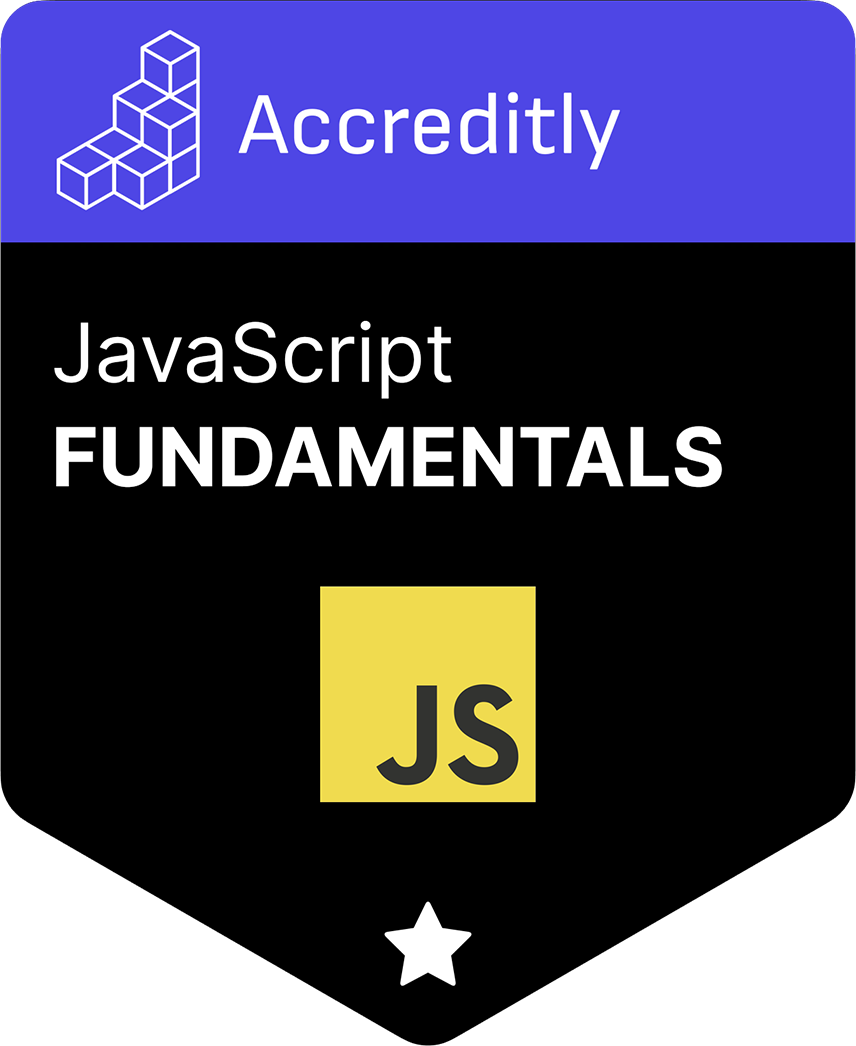