- What Are Abstract Data Types?
- Why Use ADTs in JavaScript?
- Common Abstract Data Types
- 1. Stack
- 2. Queue
- 3. Linked List
- Conclusion
Abstract Data Types (ADTs) are fundamental to understanding structured programming and software engineering, serving as the building blocks for data structure design and implementation. While JavaScript is a dynamically typed language, understanding and implementing ADTs can significantly improve the manageability and scalability of your applications. This article provides an introduction to ADTs, their importance, and examples of how to implement them in JavaScript.
What Are Abstract Data Types?
An Abstract Data Type (ADT) is a mathematical model of a data structure that specifies the type of data stored, the operations available on that data, and the types of behaviors those operations exhibit. ADTs are concerned with what each data type does, rather than how it does it (implementation details).
Why Use ADTs in JavaScript?
Even though JavaScript does not enforce strict data types, using ADTs can help in the following ways:
- Clarity and Maintainability: ADTs provide a clear specification of what operations are allowed on a data type, making code easier to understand and maintain.
- Reusability: ADTs allow developers to create data structures that can be reused across different programs.
- Encapsulation: Implementing ADTs helps encapsulate the data, ensuring that an object's data can only be modified by its methods.
Common Abstract Data Types
Let’s discuss some common ADTs and see how they can be implemented in JavaScript:
1. Stack
A stack is a collection of elements that follows the Last In First Out (LIFO) principle. Operations typically include push
(add an item), pop
(remove an item), and peek
(view the top item).
JavaScript Implementation:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.items.length === 0) {
return "Underflow";
}
return this.items.pop();
}
peek() {
return this.items[this.items.length - 1];
}
isEmpty() {
return this.items.length === 0;
}
}
2. Queue
A queue is a collection where the first element added is the first to be removed, following the First In First Out (FIFO) principle. Key operations include enqueue
(add an item), dequeue
(remove an item), and front
(view the first item).
JavaScript Implementation:
class Queue {
constructor() {
this.items = [];
}
enqueue(element) {
this.items.push(element);
}
dequeue() {
if (this.isEmpty()) {
return "Underflow";
}
return this.items.shift();
}
front() {
if (this.isEmpty()) {
return "Queue is empty";
}
return this.items[0];
}
isEmpty() {
return this.items.length === 0;
}
}
3. Linked List
A linked list is a sequence of elements, where each element links to the next. It allows for efficient insertion and removal of elements.
JavaScript Implementation:
class Node {
constructor(element) {
this.element = element;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.size = 0;
}
add(element) {
let node = new Node(element);
let current;
if (this.head == null) {
this.head = node;
} else {
current = this.head;
while (current.next) {
current = current.next;
}
current.next = node;
}
this.size++;
}
remove(element) {
let current = this.head;
let prev = null;
while (current != null) {
if (current.element === element) {
if (prev == null) {
this.head = current.next;
} else {
prev.next = current.next;
}
this.size--;
return current.element;
}
prev = current;
current = current.next;
}
return -1;
}
}
Conclusion
Understanding and implementing Abstract Data Types in JavaScript can greatly enhance your ability to structure and solve complex programming problems efficiently. While JavaScript does not provide built-in ADTs, the flexibility of the language allows you to implement these structures, providing clarity and efficiency in your code. As you develop more complex applications, the principles of ADTs will enable you to write more organized, maintainable, and scalable code.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
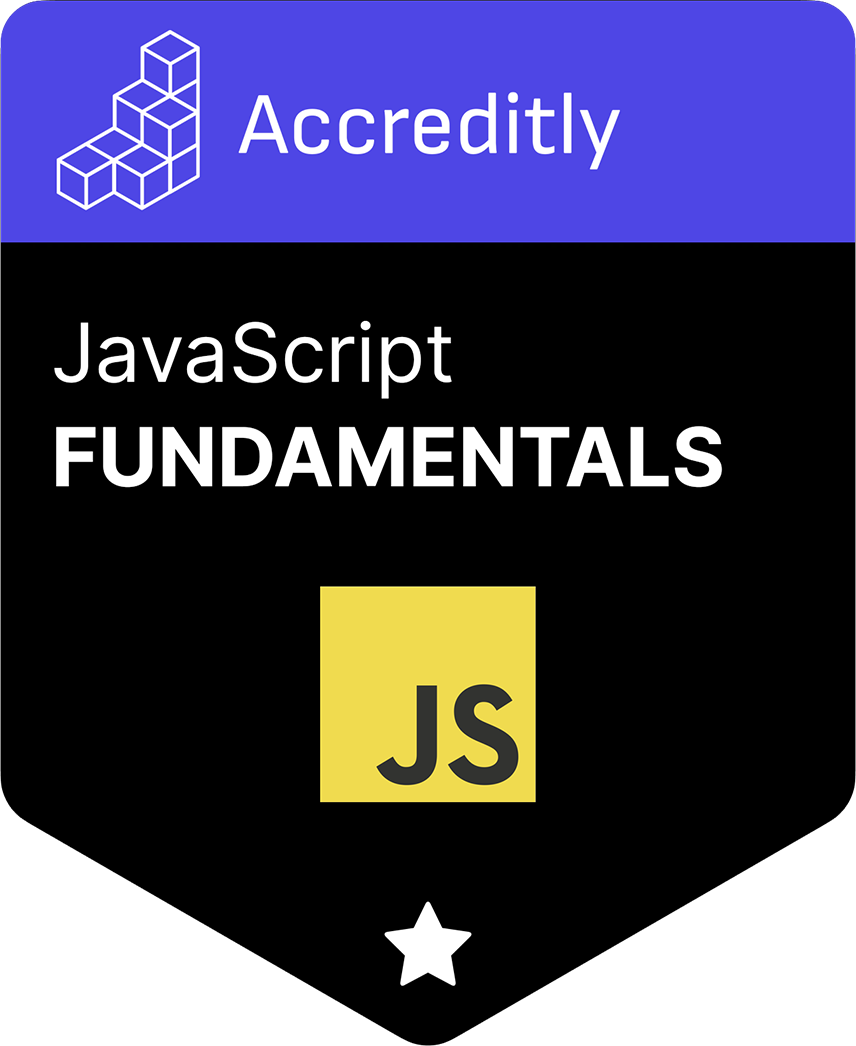