- What is Model::shouldBeStrict?
- Why Use Model::shouldBeStrict?
- Implementing Model::shouldBeStrict on a Per-Model Basis
- Implementing Model::shouldBeStrict Globally
Laravel's Eloquent ORM is designed to make database interactions smooth and intuitive. One of its lesser-known yet powerful features is Model::shouldBeStrict
. This method, when implemented, plays a vital role in data integrity and error handling in your Laravel applications. Let's explore how to implement Model::shouldBeStrict
both per model and globally, and understand why it's beneficial for your Laravel projects.
Model::shouldBeStrict
?
What is In Laravel, Model::shouldBeStrict
is a method that can be enabled to throw an error when an attempt is made to assign a value to a non-existent attribute in a model. This feature ensures that your models only accept defined attributes, preventing silent failures and potential data corruption.
$post = Post::find(1);
$post->title = 'Example post'; // Good
$post->foo = 'Bar'; // Bad, doesn't exist
$post->save();
In the above example, if shouldBeStrict
is false
, which it is by default, then you'll get no errors and everything will appear to work fine. When shouldBeStrict
is true
you'll get an error as foo
doesn't exist on Post
model.
Model::shouldBeStrict
?
Why Use Using Model::shouldBeStrict
prevents silent data loss, catches typos early, ensures data integrity, and facilitates debugging. It's particularly useful in large applications where data consistency is paramount and debugging can be challenging.
Model::shouldBeStrict
on a Per-Model Basis
Implementing You can enable shouldBeStrict
in individual Eloquent models to ensure strict attribute handling. Here's how you can do it:
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $fillable = ['name', 'email'];
protected function shouldBeStrict()
{
return true;
}
}
In this example, the User
model will throw an error if you try to set any attribute that is not name
or email
.
Model::shouldBeStrict
Globally
Implementing To enforce strict attribute checking across all models in your application, you can override the shouldBeStrict
method in a base model and extend all other models from this base model. Here's how to set it up:
1. Create a Base Model:
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class BaseModel extends Model
{
protected function shouldBeStrict()
{
return true;
}
}
2. Extend Other Models from the Base Model:
namespace App\Models;
class User extends BaseModel
{
protected $fillable = ['name', 'email'];
// Other user model methods and properties
}
By extending the User
model from BaseModel
, it inherits the shouldBeStrict
implementation, making it apply globally to all models that extend BaseModel
.
3. Implement in App Service Provider:
Open you app service provider:
public function boot(): void
{
Model::shouldBeStrict();
}
This implements strict models for every model in your app.
Implementing Model::shouldBeStrict
in Laravel, whether on a per-model basis or globally, enhances your application's robustness. It ensures that your models behave predictably, preventing silent data loss and making debugging easier. For any Laravel application, especially those dealing with complex data structures, using Model::shouldBeStrict
is a best practice that contributes significantly to maintaining data integrity and reliability.
If you're interested in deep diving under the hood, then here are the official Laravel API docs for shouldBeStrict
.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
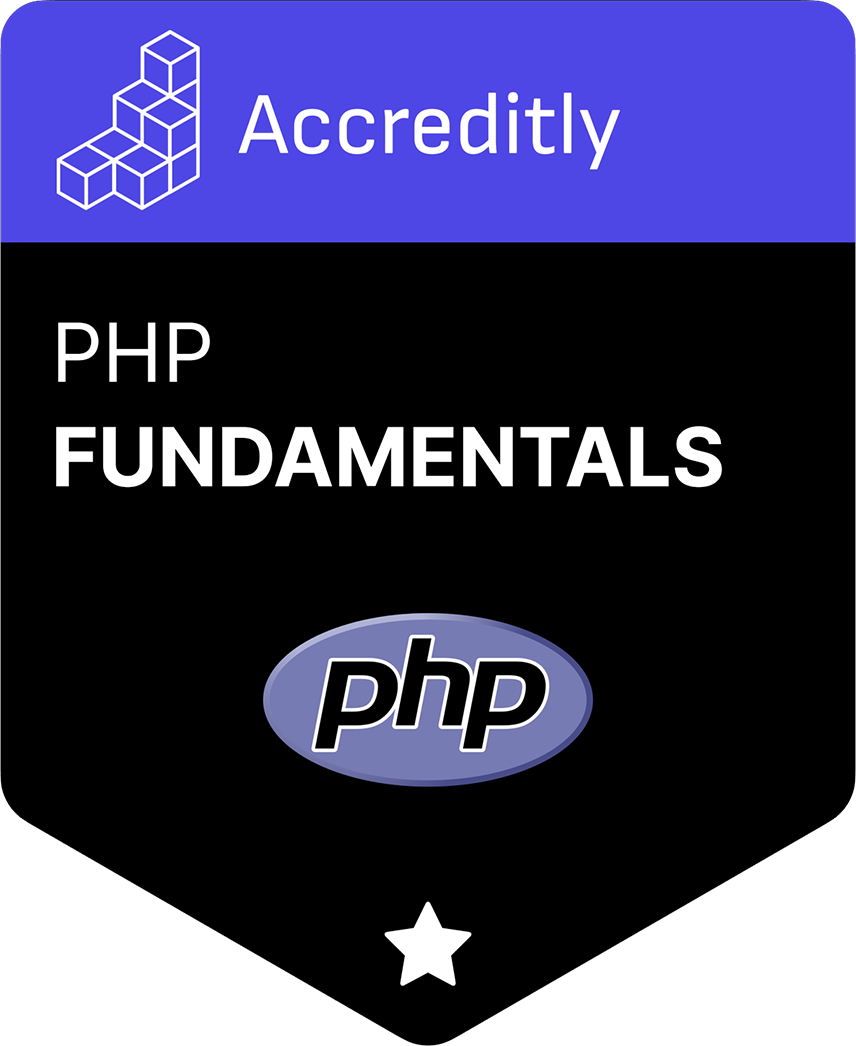