- What is a URL Slug?
- Why Use URL Slugs?
- Steps to Create a URL Slug in PHP
- Putting It All Together
- Handling Accented Characters
- Further reading
Creating URL slugs is a common requirement in web development, especially for SEO-friendly URLs. A URL slug is a user-friendly string derived from a title or name, typically used in web addresses to make them more readable and descriptive. In this article, we'll explore how to create a URL slug string in PHP step-by-step.
What is a URL Slug?
A URL slug is the part of a URL that identifies a particular page on a website in a user-friendly way. For example, in the URL https://example.com/blog/create-url-slug-php
, the slug is create-url-slug-php
. Slugs are typically lowercase, with words separated by hyphens, and devoid of any special characters or spaces.
Why Use URL Slugs?
- URL slugs help search engines understand the content of a page, improving its search ranking.
- They make URLs easier to read and remember.
- Slugs contribute to cleaner, more professional-looking URLs.
Steps to Create a URL Slug in PHP
Creating a URL slug in PHP involves several steps:
- Convert the string to lowercase.
- Remove special characters.
- Replace spaces with hyphens.
- Trim any leading or trailing hyphens.
Let's break down each step with the corresponding PHP functions.
1. Convert the String to Lowercase
The first step is to convert the string to lowercase using the strtolower
function. This ensures consistency in the URL slug.
$title = "How to Create a URL Slug String in PHP";
$lowercaseTitle = strtolower($title);
2. Remove Special Characters
To remove special characters, you can use the preg_replace
function, which performs a regular expression search and replace. We’ll replace any character that is not a letter, number, or space with an empty string.
$slug = preg_replace('/[^a-z0-9\s]/', '', $lowercaseTitle);
3. Replace Spaces with Hyphens
Next, we replace all spaces with hyphens using the str_replace
function. This is crucial for making the URL slug readable and SEO-friendly.
$slug = str_replace(' ', '-', $slug);
4. Trim Leading and Trailing Hyphens
Finally, we trim any leading or trailing hyphens using the trim
function. This ensures the slug doesn't start or end with a hyphen.
$slug = trim($slug, '-');
Putting It All Together
Now, let's combine all the steps into a single function that generates a URL slug from a given string.
function createSlug($string) {
// Convert to lowercase
$slug = strtolower($string);
// Remove special characters
$slug = preg_replace('/[^a-z0-9\s]/', '', $slug);
// Replace spaces with hyphens
$slug = str_replace(' ', '-', $slug);
// Trim leading and trailing hyphens
$slug = trim($slug, '-');
return $slug;
}
// Example usage
$title = "How to Create a URL Slug String in PHP";
$slug = createSlug($title);
echo $slug; // Output: how-to-create-a-url-slug-string-in-php
Handling Accented Characters
If your titles contain accented characters, you need to handle them appropriately. You can use the iconv
function to transliterate accented characters to their ASCII equivalents.
function createSlug($string) {
// Convert to lowercase
$slug = strtolower($string);
// Transliterate accented characters to ASCII
$slug = iconv('UTF-8', 'ASCII//TRANSLIT', $slug);
// Remove special characters
$slug = preg_replace('/[^a-z0-9\s]/', '', $slug);
// Replace spaces with hyphens
$slug = str_replace(' ', '-', $slug);
// Trim leading and trailing hyphens
$slug = trim($slug, '-');
return $slug;
}
// Example usage
$title = "Création d'un Slug URL en PHP";
$slug = createSlug($title);
echo $slug; // Output: creation-d-un-slug-url-en-php
Further reading
Creating a URL slug in PHP involves a series of straightforward steps: converting to lowercase, removing special characters, replacing spaces with hyphens, and trimming hyphens. By following these steps, you can generate clean, SEO-friendly URLs for your web applications.
For more detailed information on the PHP functions used in this article, check out their official documentation:
By incorporating these techniques into your development process, you can enhance the readability and SEO performance of your web application's URLs.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
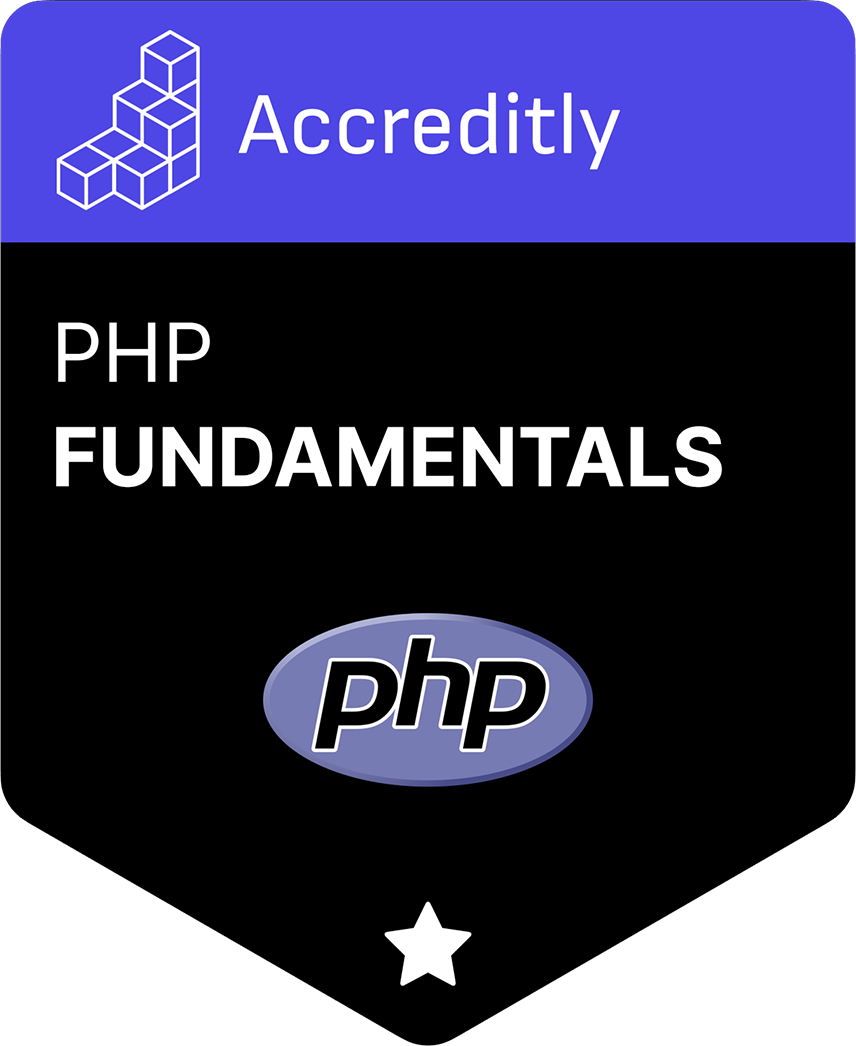