- 1. Getting the Caret Position
- 2. Setting the Caret Position
- 3. Copying Text from a Specific Position
- 4. Pasting Text at the Caret Position
- 5. Enhancing User Experience
In JavaScript, the caret, or text cursor, is the flashing bar inside input fields and textareas that indicates where text will be inserted. Manipulating the caret position allows for enhanced text operations, such as specific copy and paste actions. In this tutorial, we'll explore how to leverage the caret's position to copy and paste text in JavaScript.
1. Getting the Caret Position
Before we can use the caret for copy and paste operations, we need to determine its position.
HTML Setup:
<textarea id="textArea"></textarea>
JavaScript:
function getCaretPosition(textElement) {
return textElement.selectionStart;
}
Usage:
const textArea = document.getElementById('textArea');
console.log(getCaretPosition(textArea)); // Returns the caret position
2. Setting the Caret Position
Sometimes you might want to move the caret to a specific position:
function setCaretPosition(textElement, position) {
textElement.selectionStart = position;
textElement.selectionEnd = position;
}
Usage:
setCaretPosition(textArea, 5); // Sets the caret position to 5
3. Copying Text from a Specific Position
To copy text from a specific position, you can utilize the caret's position.
function copyTextFromPosition(textElement, start, end) {
return textElement.value.substring(start, end);
}
Usage:
console.log(copyTextFromPosition(textArea, 0, 5)); // Copies the first 5 characters
4. Pasting Text at the Caret Position
To insert text at the caret's current position:
function pasteTextAtCaret(textElement, text) {
const beforeCaret = textElement.value.substring(0, textElement.selectionStart);
const afterCaret = textElement.value.substring(textElement.selectionEnd, textElement.value.length);
textElement.value = beforeCaret + text + afterCaret;
// Position the caret after the inserted text
setCaretPosition(textElement, beforeCaret.length + text.length);
}
Usage:
pasteTextAtCaret(textArea, "Hello, World!"); // Inserts "Hello, World!" at the current caret position
5. Enhancing User Experience
For better user interaction, you can add buttons to trigger these functions.
HTML:
<textarea id="textArea"></textarea>
<button onclick="copyText()">Copy Text</button>
<button onclick="pasteText()">Paste Text</button>
JavaScript:
let copiedText = '';
function copyText() {
copiedText = copyTextFromPosition(textArea, 0, 5); // Modify as needed
alert(`Copied: ${copiedText}`);
}
function pasteText() {
pasteTextAtCaret(textArea, copiedText);
}
With the power of JavaScript and knowledge about the caret's position, you can create intricate text manipulations in input fields and textareas. By determining and controlling the caret's position, you can customize how text is copied and pasted to enhance user interactions. Whether you're building a custom text editor or a unique user interface, understanding and manipulating the caret is a valuable skill.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
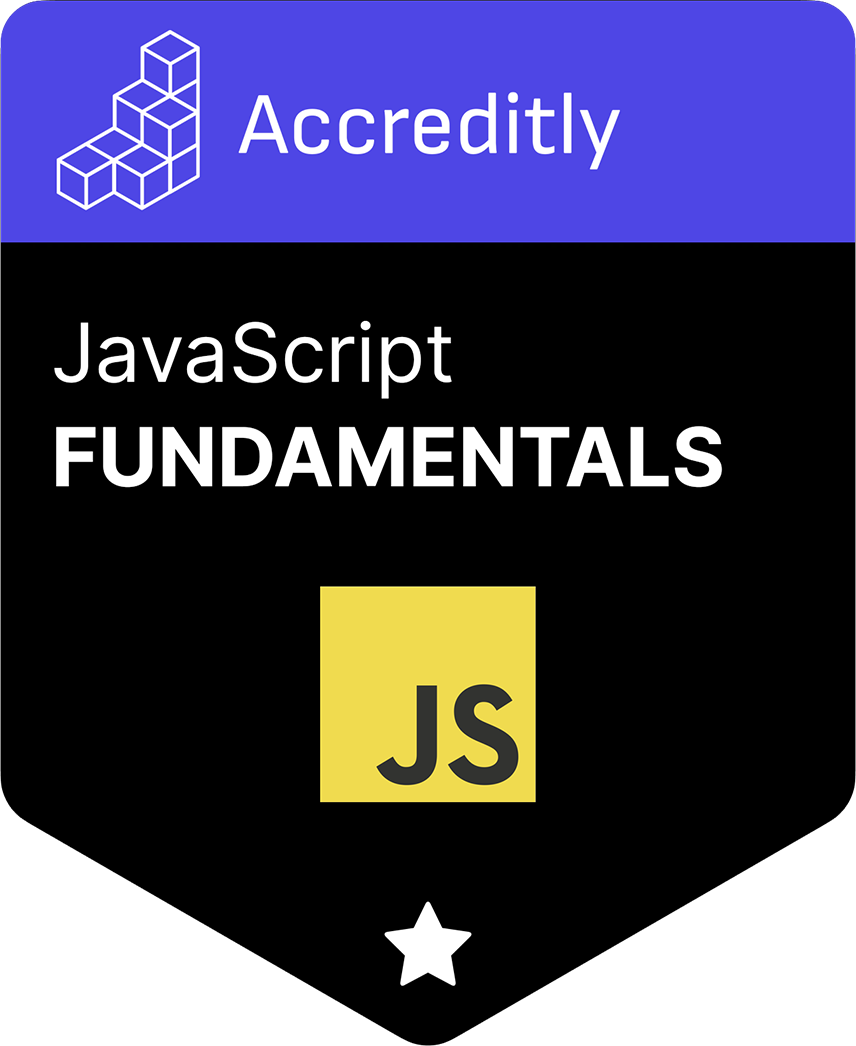