- Getting Started with the Vibration API
- Creating Vibration Patterns
- Cancelling Vibrations
- Practical Use Cases for the Vibration API
- Considerations and Best Practices
- Testing the Vibration API
- Enhancing User Experience with Vibration
The Vibration API is a Web API that allows developers to control the vibration hardware of mobile devices. It enables you to trigger vibrations for a specific duration or pattern, offering an additional layer of feedback to users, especially in scenarios where visual or auditory cues might not be enough.
This API is particularly useful for mobile web applications where tactile feedback can play a significant role, such as in games, notifications, or even form submissions. It's important to note that the Vibration API is not supported on all devices—primarily, it works on mobile devices that have vibration hardware.
Getting Started with the Vibration API
Using the Vibration API is straightforward. The API consists of a single method: navigator.vibrate()
. This method accepts either a single number representing the duration of the vibration in milliseconds or an array of numbers that define a pattern of vibrations and pauses.
Here's a basic example of how to trigger a vibration for 200 milliseconds:
if ("vibrate" in navigator) {
navigator.vibrate(200);
} else {
console.log("Vibration API is not supported on this device.");
}
In this code snippet, we first check if the vibrate
method exists in the navigator
object, ensuring that the API is supported on the device. If it is, we call navigator.vibrate(200)
to vibrate the device for 200 milliseconds. If not, we log a message to the console.
Creating Vibration Patterns
The Vibration API also allows for more complex vibration patterns. By passing an array of values to navigator.vibrate()
, you can create sequences of vibrations and pauses. Each value in the array represents either a vibration duration or a pause duration in milliseconds.
For example, to create a pattern of a short vibration followed by a pause and then a longer vibration, you can use:
if ("vibrate" in navigator) {
navigator.vibrate([100, 50, 200]);
} else {
console.log("Vibration API is not supported on this device.");
}
In this example, the device will vibrate for 100 milliseconds, pause for 50 milliseconds, and then vibrate again for 200 milliseconds.
Cancelling Vibrations
If you need to stop a vibration pattern before it completes, you can pass a 0
or an empty array []
to navigator.vibrate()
. This will immediately cancel any ongoing vibration.
// Start a vibration pattern
navigator.vibrate([200, 100, 200]);
// Cancel the vibration after 300ms
setTimeout(() => {
navigator.vibrate(0);
}, 300);
In this example, we initiate a vibration pattern and then cancel it after 300 milliseconds, effectively stopping the ongoing vibration.
Practical Use Cases for the Vibration API
1. Form Submission Feedback
You can use the Vibration API to provide feedback when a user submits a form. For example, a successful form submission might trigger a short vibration, while an error could trigger a longer or more complex pattern.
document.getElementById("myForm").addEventListener("submit", function(event) {
event.preventDefault();
// Simulate form submission logic here
if (formIsValid()) {
navigator.vibrate(100); // Short vibration for success
} else {
navigator.vibrate([200, 50, 200, 50, 200]); // Pattern for error
}
});
In this scenario, the user gets immediate tactile feedback based on the form's outcome, enhancing the user experience.
2. Game Interactions
In mobile games, you can use the Vibration API to enhance gameplay. For example, a vibration could signal a character's hit, a collision, or reaching a significant milestone.
function onCharacterHit() {
navigator.vibrate([50, 100, 50]);
}
function onMilestoneReached() {
navigator.vibrate(200);
}
By integrating vibration feedback into your game, you make it more immersive, giving players a physical sense of the in-game events.
3. Notifications
Web applications often rely on notifications to keep users informed. Adding a vibration to these notifications, especially for important alerts, can ensure that users don't miss critical information.
if (Notification.permission === "granted") {
new Notification("Important Alert!", {
body: "You have a new message.",
vibrate: [200, 100, 200]
});
}
In this example, the user is alerted with both a visual notification and a vibration, increasing the likelihood that they will notice the message.
Considerations and Best Practices
While the Vibration API is a powerful tool, there are a few best practices to keep in mind to ensure you're using it effectively and responsibly:
1. Respect User Preferences
Not all users appreciate unexpected vibrations. Consider providing an option in your application settings to enable or disable vibrations. Additionally, always respect the device's vibration settings and do not override them.
2. Use Sparingly
Vibrations can be disruptive, especially in quiet environments or when overused. Use vibrations judiciously to avoid frustrating your users. Focus on critical interactions where tactile feedback significantly enhances the user experience.
3. Cross-Browser Compatibility
The Vibration API is supported in most modern mobile browsers, but not all. Ensure you have fallback logic in place for browsers or devices that do not support it. Always check for the API's presence before attempting to use it.
4. Battery Considerations
Continuous or excessive use of vibrations can drain the battery faster. Be mindful of this, especially in applications that are intended to run for extended periods.
Testing the Vibration API
To test the Vibration API, you'll need a device with vibration hardware. Desktop browsers won't support this API unless you're using an emulator with vibration capabilities. The simplest way to test your code is directly on a mobile device using Chrome's DevTools or similar tools for other browsers.
Example Test Scenario
-
Simple Vibration Test:
navigator.vibrate(300);
Expected outcome: The device vibrates for 300 milliseconds.
-
Pattern Vibration Test:
navigator.vibrate([100, 200, 100, 200]);
Expected outcome: The device vibrates for 100 milliseconds, pauses for 200 milliseconds, and repeats this pattern.
-
Cancel Vibration Test:
navigator.vibrate(500); setTimeout(() => { navigator.vibrate(0); }, 200);
Expected outcome: The vibration starts but stops after 200 milliseconds.
Enhancing User Experience with Vibration
The Vibration API in JavaScript provides a simple yet effective way to add tactile feedback to your web applications, enhancing user experience, especially on mobile devices. Whether you're using it for notifications, game interactions, or form submissions, vibrations can make your app feel more responsive and interactive.
However, it's crucial to use this API thoughtfully. Always consider the user's environment, preferences, and device capabilities when implementing vibrations in your web applications. With careful use, the Vibration API can be a valuable tool in creating engaging and user-friendly experiences.
For more information, you can refer to the MDN Web Docs on Vibration API, which provides a comprehensive guide to the API's features and browser compatibility.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
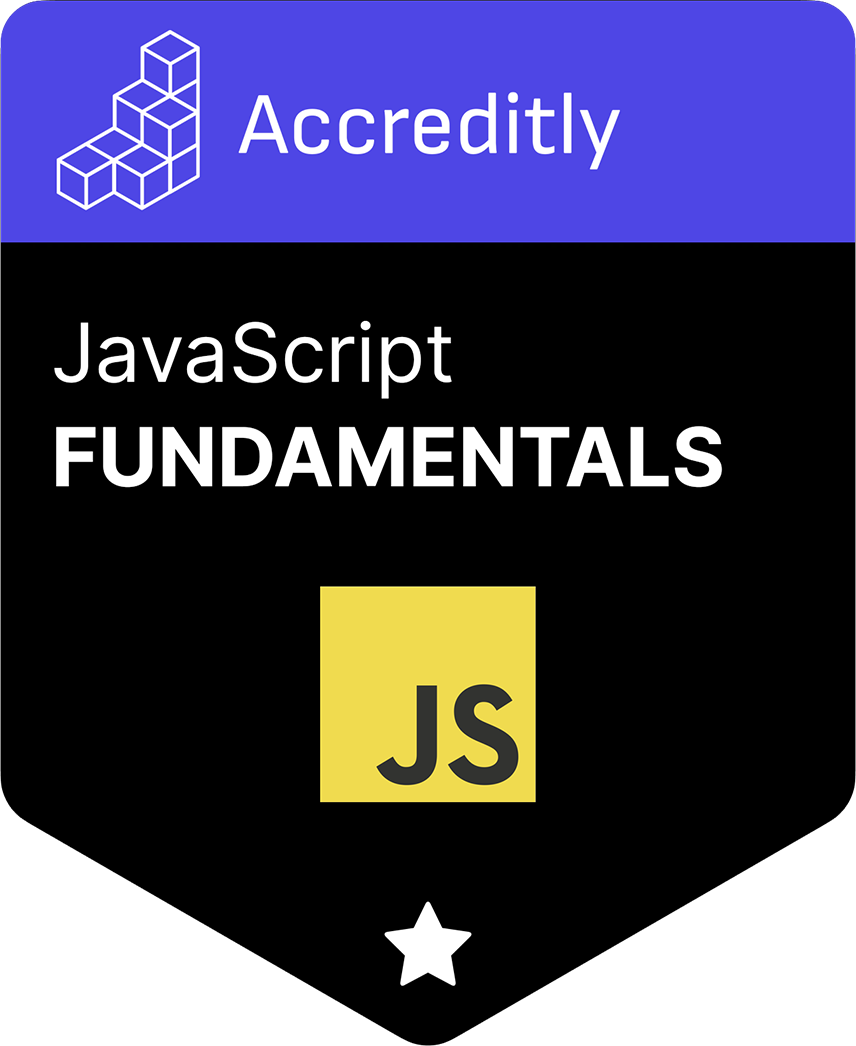