- 1. Understanding declare(strict_types=1)
- 2. Benefits of Using declare(strict_types=1)
- 3. Examples of declare(strict_types=1) Usage
- Wrapping up
In PHP, type declarations can help improve the robustness and maintainability of your code. However, by default, PHP uses weak type checking, which can sometimes lead to unexpected behavior. With the declare(strict_types=1)
directive, you can enforce strict typing in your PHP code, ensuring that type mismatches are caught early on. In this article, we will explore the benefits of using declare(strict_types=1)
and provide examples of how to use it in your PHP projects.
declare(strict_types=1)
1. Understanding By adding the declare(strict_types=1)
directive at the beginning of your PHP file, you can enforce strict type checking for that specific file. This means that PHP will throw a TypeError
when a type mismatch occurs, rather than trying to coerce the value to the expected type.
Here's an example of how to use declare(strict_types=1)
in a PHP file:
<?php
declare(strict_types=1);
function add(int $a, int $b): int {
return $a + $b;
}
$result = add(5, 3); // This will work
$result = add(5, "3"); // This will throw a TypeError
declare(strict_types=1)
2. Benefits of Using There are several advantages to using declare(strict_types=1)
in your PHP code:
a. Improved code reliability:
By enforcing strict typing, you can catch potential issues before they become bugs. This helps improve the overall reliability and maintainability of your codebase.
b. Better communication of intent:
When you use strict typing, you clearly communicate the expected types for function arguments and return values. This makes your code more self-documenting and easier to understand for other developers.
c. Easier debugging:
When a TypeError
is thrown due to a type mismatch, you can quickly identify and fix the issue. This is much easier than trying to track down subtle bugs caused by weak type checking.
declare(strict_types=1)
Usage
3. Examples of Here are a few examples of using declare(strict_types=1)
in your PHP code:
a. Enforcing the correct type for function arguments:
<?php
declare(strict_types=1);
function greet(string $name): void {
echo "Hello, $name!";
}
greet("John"); // This will work
greet(42); // This will throw a TypeError
b. Enforcing the correct type for function return values:
<?php
declare(strict_types=1);
function square(int $number): int {
return $number * $number;
}
$result = square(4); // This will work
$result = square("4"); // This will throw a TypeError
c. Enforcing strict typing for class methods:
<?php
declare(strict_types=1);
class Calculator {
public function add(float $a, float $b): float {
return $a + $b;
}
}
$calc = new Calculator();
$result = $calc->add(2.5, 3.5); // This will work
$result = $calc->add(2.5, "3.5"); // This will throw a TypeError
Wrapping up
Using declare(strict_types=1)
in your PHP code is a simple and effective way to enforce strict typing and improve the reliability of your codebase. By incorporating this directive into your projects, you can catch type mismatches early on, making debugging easier and preventing potential bugs from slipping through unnoticed.
Keep in mind that declare(strict_types=1)
only affects the file it is declared in. If you want to enforce strict typing across your entire codebase, you will need to add the directive to each PHP file.
Additionally, be aware that strict typing can sometimes cause issues when working with third-party libraries or legacy code that was not designed with strict typing in mind. In such cases, you might need to adapt the code or create wrappers to ensure compatibility with strict typing.
By adopting strict typing practices, you can enhance your PHP code's quality, making it easier to maintain and less prone to errors. So, if you haven't already, consider adding declare(strict_types=1)
to your PHP projects and enjoy the benefits of a more robust and maintainable codebase.
The equivalent is also available in other languages, most notably JavaScripts strict mode.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
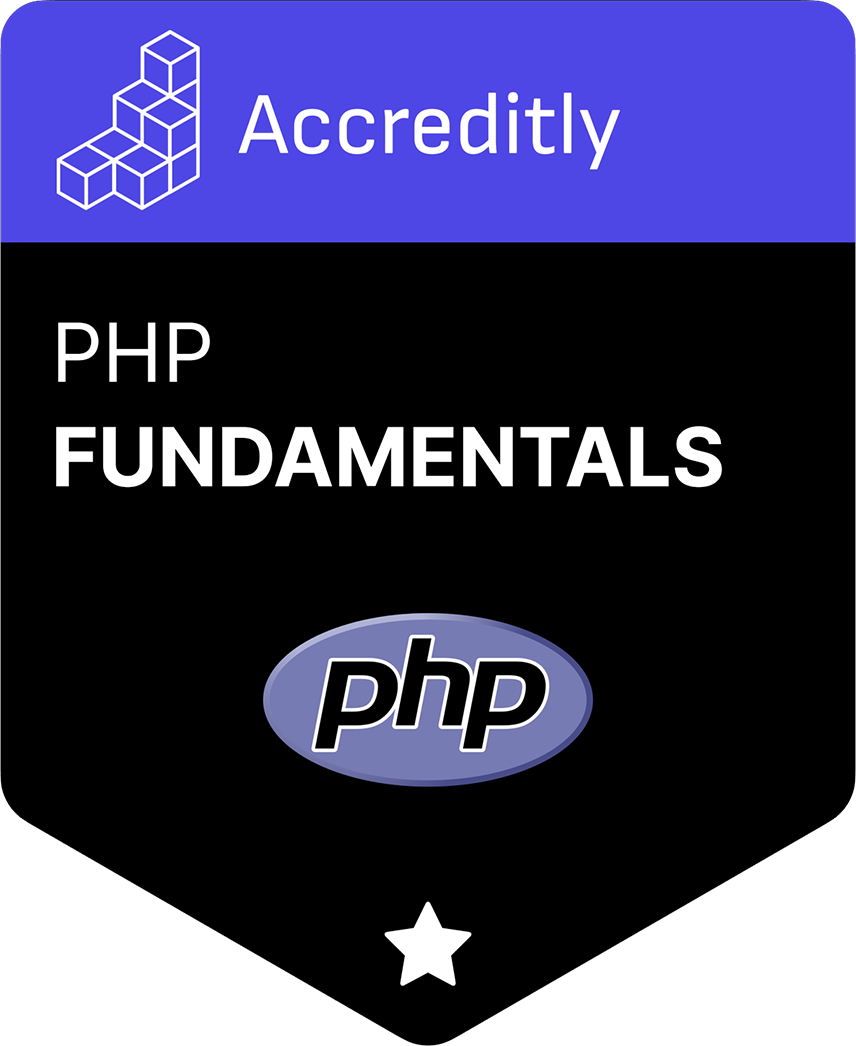