- Creating a Promise
- Resolving a Promise
- Rejecting a Promise
- Handling a Promise
- Chaining Promises
- Conclusion
Promises are a way to handle asynchronous operations in JavaScript. They provide a way to execute code asynchronously and then handle the result when it is available.
Creating a Promise
To create a new Promise, use the Promise
constructor:
const promise = new Promise((resolve, reject) => {
// code to execute asynchronously
});
The Promise
constructor takes a single function as an argument, which is called the executor function. This function takes two arguments: resolve
and reject
. resolve
is a function that is called when the asynchronous operation completes successfully, and reject
is a function that is called when the operation fails.
Resolving a Promise
To resolve a Promise
, call the resolve function with the value you want to return:
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Hello, World!");
}, 1000);
});
This Promise
will wait for 1 second (due to the setTimeout
function) and then resolve with the value "Hello, World!"
.
Rejecting a Promise
To reject a Promise
, call the reject
function with an error object:
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error("Something went wrong!"));
}, 1000);
});
This Promise
will wait for 1 second (due to the setTimeout
function) and then reject with an Error
object containing the message "Something went wrong!"
.
Handling a Promise
To handle the result of a Promise
, use the then
method:
promise.then((result) => {
console.log(result);
}).catch((error) => {
console.error(error);
});
The then
method takes a function as an argument, which will be called with the result of the Promise
when it is resolved. The catch
method takes a function as an argument, which will be called with the error
object when the Promise
is rejected.
Chaining Promises
Promises can be chained together using the then
method. This is useful when you need to perform multiple asynchronous operations in a specific order:
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve(10);
}, 1000);
});
promise.then((result) => {
console.log(result); // 10
return result * 2;
}).then((result) => {
console.log(result); // 20
}).catch((error) => {
console.error(error);
});
This example creates a Promise
that resolves with the value 10
, and then chains two then methods together to multiply that value by 2
and log the result.
Conclusion
Promises are a powerful way to handle asynchronous operations in JavaScript. They allow you to execute code asynchronously and then handle the result when it is available. With Promises, you can write more concise and readable code, and avoid complex nested callbacks.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
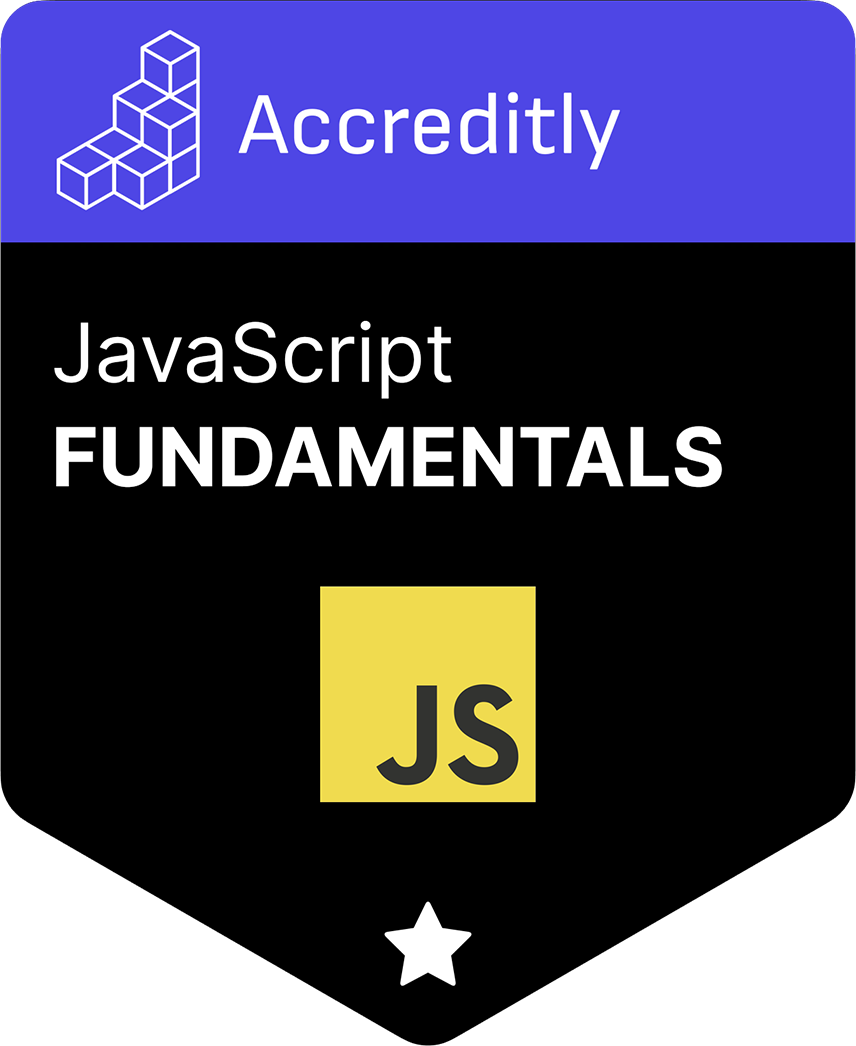