- 1. Set the Default Timezone
- 2. Working with DateTime Objects
- 3. Converting Between Timezones
- 4. Formatting Dates and Times with Timezones
- 5. List of Supported Timezones
- 6. Dealing with Daylight Saving Time
- 7. Storing Dates and Times in a Database
- 8. Calculating Time Differences
- 9. Working with Unix Timestamps
- 10. Date and Time Functions in PHP
- Summing up
Timezone management is an essential aspect of web development, especially when dealing with users from different geographical locations. PHP, a popular server-side scripting language, offers various built-in features to handle timezones effectively. This article will discuss how to properly manage timezones in PHP, including setting the timezone, working with DateTime
objects, and converting between timezones.
Already comfortable dealing with timezones? Take our PHP certification and showcase your knowledge.
1. Set the Default Timezone
Before working with dates and times in PHP, it's essential to set the default timezone. PHP uses this timezone to calculate date and time-related functions when a specific timezone is not provided. You can set the default timezone using the date_default_timezone_set()
function or the date.timezone
directive in the php.ini
configuration file.
date_default_timezone_set()
1.1. Using To set the default timezone in your PHP script, use the date_default_timezone_set()
function with the desired timezone as its parameter. Here's an example:
date_default_timezone_set('Europe/London');
php.ini
Configuration File
1.2. Using the Alternatively, you can set the default timezone in the php.ini
file using the date.timezone
directive. Open your php.ini
file and search for date.timezone
. If it's commented out, uncomment it and set the desired timezone:
date.timezone = "Europe/London"
Don't forget to restart your web server after making changes to the php.ini file.
DateTime
Objects
2. Working with PHP's DateTime
class provides an easy way to work with dates and times, including timezone conversions. To create a new DateTime
object, use the following syntax:
$dateTime = new DateTime('now', new DateTimeZone('Europe/London'));
The first parameter is the date and time, and the second parameter is an instance of the DateTimeZone
class representing the desired timezone. In this example, we created a DateTime
object for the current date and time in the 'Europe/London' timezone.
3. Converting Between Timezones
To convert a DateTime
object between timezones, you can use the setTimezone()
method. This method accepts a DateTimeZone
object representing the target timezone. Here's an example:
$dateTime = new DateTime('now', new DateTimeZone('Europe/London'));
$dateTime->setTimezone(new DateTimeZone('America/New_York'));
In this example, we created a DateTime
object for the current date and time in the 'Europe/London' timezone and then converted it to the 'America/New_York' timezone.
4. Formatting Dates and Times with Timezones
To display a DateTime
object's date and time in a specific format, you can use the format()
method. This method accepts a format string as its parameter. Here's an example:
$dateTime = new DateTime('now', new DateTimeZone('Europe/London'));
echo $dateTime->format('Y-m-d H:i:s T');
This example will output the current date and time in the 'America/New_York' timezone, along with the timezone abbreviation (e.g., '2023-03-23 12:34:56 EDT').
5. List of Supported Timezones
PHP supports a wide range of timezones. To see the complete list of supported timezones, refer to the PHP Manual's list of supported timezones.
6. Dealing with Daylight Saving Time
Daylight Saving Time (DST) can be a source of confusion when dealing with timezones. Fortunately, PHP's DateTime
and DateTimeZone
classes handle DST automatically, so you don't need to worry about making manual adjustments. When you create a DateTime
object or convert between timezones, PHP accounts for any DST changes applicable to the specified timezone and date.
$dateTime = new DateTime('2023-03-12 01:59:59', new DateTimeZone('America/New_York'));
echo $dateTime->format('Y-m-d H:i:s T'); // Output: 2023-03-12 01:59:59 EST
$dateTime->modify('+1 second');
echo $dateTime->format('Y-m-d H:i:s T'); // Output: 2023-03-12 03:00:00 EDT
In this example, we created a DateTime
object for a date and time just before the DST change in the 'America/New_York' timezone. After adding one second, the output reflects the correct time and timezone abbreviation, accounting for the DST change.
7. Storing Dates and Times in a Database
When storing dates and times in a database, it's a good practice to use the UTC timezone to ensure consistency and easy conversion between timezones. Most databases support a TIMESTAMP
or DATETIME
data type that can store timezone-aware dates and times.
To store a DateTime
object in the UTC timezone, convert it to UTC before formatting it for insertion into the database:
$dateTime = new DateTime('now', new DateTimeZone('America/New_York'));
$dateTime->setTimezone(new DateTimeZone('UTC'));
$dateTimeUtc = $dateTime->format('Y-m-d H:i:s');
When retrieving dates and times from the database, you can convert them back to the user's timezone:
$dateTimeUtc = '2023-03-23 12:34:56'; // Retrieved from the database
$dateTime = new DateTime($dateTimeUtc, new DateTimeZone('UTC'));
$dateTime->setTimezone(new DateTimeZone('America/New_York'));
8. Calculating Time Differences
To calculate the difference between two dates and times in PHP, you can use the diff() method of the DateTime
class. This method accepts another DateTime
object as its parameter and returns a DateInterval
object representing the difference.
$dateTime1 = new DateTime('2023-03-23 12:00:00', new DateTimeZone('America/New_York'));
$dateTime2 = new DateTime('2023-03-23 18:00:00', new DateTimeZone('Europe/London'));
$interval = $dateTime1->diff($dateTime2);
echo $interval->format('%h hours and %i minutes'); // Output: 5 hours and 0 minutes
In this example, we calculated the difference between two DateTime
objects in different timezones. The output is the correct time difference, taking into account the timezone offsets.
By following these guidelines and using PHP's built-in features, you can effectively manage timezones in your web applications and ensure a smooth user experience for visitors from around the world.
9. Working with Unix Timestamps
Unix timestamps, which represent the number of seconds since January 1, 1970, at 00:00:00 UTC, are another way to work with dates and times in PHP. They are timezone-independent and can simplify some operations. However, keep in mind that they have limitations, such as the inability to represent dates before 1970 or after January 19, 2038, on 32-bit systems.
To create a DateTime
object from a Unix timestamp, use the @
symbol followed by the timestamp:
$timestamp = 1677649200; // Unix timestamp for 2023-03-23 12:00:00 UTC
$dateTime = new DateTime("@$timestamp");
$dateTime->setTimezone(new DateTimeZone('America/New_York'));
echo $dateTime->format('Y-m-d H:i:s T'); // Output: 2023-03-23 08:00:00 EDT
To convert a DateTime object to a Unix timestamp, use the U
format:
$dateTime = new DateTime('2023-03-23 12:00:00', new DateTimeZone('America/New_York'));
$timestamp = $dateTime->format('U');
echo $timestamp; // Output: 1677649200
10. Date and Time Functions in PHP
In addition to the DateTime
and DateTimeZone
classes, PHP offers several built-in date and time functions that can be useful in specific situations. Some of these functions include:
-
time()
: Returns the current Unix timestamp. -
mktime()
: Creates a Unix timestamp from a given date and time. -
strtotime()
: Parses a date string and returns a Unix timestamp. -
date()
: Formats a date and time as a string, given a Unix timestamp. -
gmdate()
: Formats a date and time as a string, given a Unix timestamp, in the UTC timezone.
While these functions can be helpful in some cases, it's generally recommended to use the DateTime
and DateTimeZone
classes for more robust and accurate date and time handling in PHP.
Summing up
Managing timezones correctly in PHP applications is crucial for providing a consistent user experience and avoiding potential issues with date and time calculations. By understanding the basics of timezone handling in PHP and leveraging the power of the DateTime
and DateTimeZone
classes, you can ensure that your application works seamlessly for users around the globe. Remember to store dates and times in a consistent format, such as UTC, in your database, and always convert them to the user's local timezone when displaying them. With these best practices in place, you can confidently build applications that work flawlessly with timezones.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
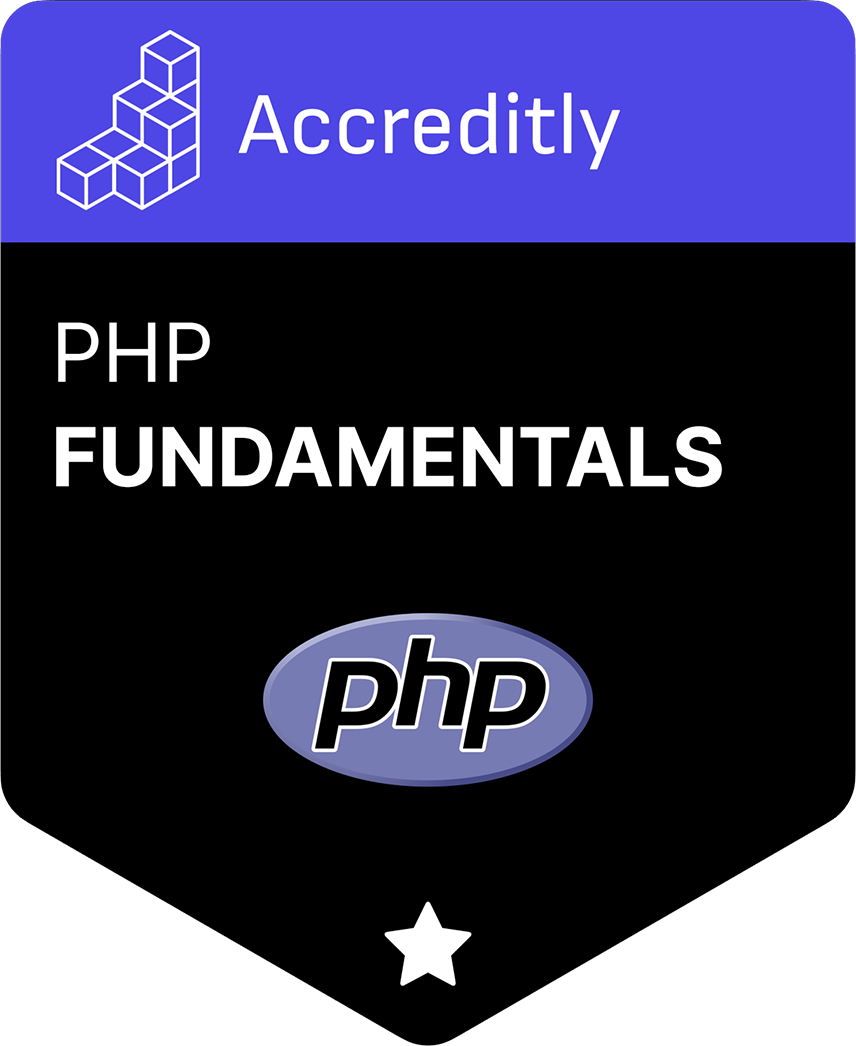