- The if Statement
- The switch Statement
- The match Expression
- Practical Scenarios
- Scenario 1: User Authentication
- Scenario 2: Processing Form Input
- Scenario 3: HTTP Status Codes
- Choosing the Right Tool
- Additional Resources
One of the fundamental aspects of any programming language is its ability to control the flow of the program based on different conditions. PHP offers several ways to accomplish this, with if
, switch
, and match
statements being the most prominent. In this article, we'll delve into each of these conditional statements, exploring their syntax, use cases, and the scenarios in which each should be used.
Conditional statements allow developers to execute different blocks of code based on specific conditions. This ability is crucial for making decisions within a program, handling different input values, and managing various states in an application.
if
Statement
The The if
statement is the most basic and widely used conditional statement in PHP. It evaluates a condition and executes a block of code if the condition is true.
if
Statement
Syntax of if (condition) {
// Code to be executed if condition is true
}
Example
$age = 20;
if ($age >= 18) {
echo "You are an adult.";
} else {
echo "You are a minor.";
}
if
When to Use -
Simple Conditions: Use
if
for straightforward, single-condition checks. -
Multiple Conditions: Combine
if
withelse if
andelse
for more complex decision-making. - Boolean Checks: Ideal for checking true/false values.
switch
Statement
The The switch
statement is an alternative to the if
statement, often used when dealing with multiple possible values for a single variable. It compares the variable against different cases and executes the corresponding block of code.
switch
Statement
Syntax of switch (variable) {
case value1:
// Code to be executed if variable == value1
break;
case value2:
// Code to be executed if variable == value2
break;
default:
// Code to be executed if variable doesn't match any case
}
Example
$day = "Tuesday";
switch ($day) {
case "Monday":
echo "Start of the work week!";
break;
case "Tuesday":
echo "Second day of the work week!";
break;
case "Friday":
echo "Last workday of the week!";
break;
default:
echo "It's the weekend!";
}
switch
When to Use - Multiple Known Values: Ideal when a variable can have one of several known values.
-
Clean Code: Provides a cleaner and more readable alternative to multiple
if-else
statements. - Exact Matches: Best suited for exact value matches rather than range or boolean checks.
match
Expression
The Introduced in PHP 8.0, the match
expression is a more powerful and flexible version of the switch
statement. It returns a value based on the condition that matches.
match
Expression
Syntax of $result = match (variable) {
value1 => result1,
value2 => result2,
default => defaultResult,
};
Example
$status = 404;
$message = match ($status) {
200 => "OK",
404 => "Not Found",
500 => "Internal Server Error",
default => "Unknown status code",
};
echo $message; // Outputs: Not Found
match
When to Use -
Return Values: Use
match
when you need to return values based on conditions. -
Type Safety: Ensures strict type comparisons, avoiding potential pitfalls of loose comparisons in
switch
. - Conciseness: Offers a more concise and expressive syntax for mapping conditions to results.
Practical Scenarios
To understand when to use if
, switch
, and match
, let's look at some practical scenarios:
Scenario 1: User Authentication
When validating user credentials, an if
statement is appropriate because you are dealing with boolean checks.
$username = "admin";
$password = "password123";
if ($username === "admin" && $password === "password123") {
echo "Welcome, admin!";
} else {
echo "Invalid credentials.";
}
Scenario 2: Processing Form Input
When processing a form where a single input can have multiple known values, a switch
statement is ideal.
$action = $_POST['action'];
switch ($action) {
case 'save':
saveData();
break;
case 'delete':
deleteData();
break;
case 'update':
updateData();
break;
default:
echo "Invalid action.";
}
Scenario 3: HTTP Status Codes
When mapping HTTP status codes to messages, the match
expression is the most suitable due to its concise syntax and return value capabilities.
$statusCode = getStatusCodeFromServer();
$message = match ($statusCode) {
200 => "OK",
301 => "Moved Permanently",
404 => "Not Found",
500 => "Internal Server Error",
default => "Unknown status code",
};
echo $message;
Choosing the Right Tool
Choosing between if
, switch
, and match
depends on the specific requirements of your code:
- Use
if
for simple, boolean-based conditions and complex, multi-conditional checks. - Use
switch
for scenarios with multiple known values for a single variable, where readability and maintainability are crucial. - Use
match
for more concise and type-safe condition-result mappings, particularly when returning values directly.
By understanding the strengths and appropriate use cases for each conditional statement, you can write more efficient, readable, and maintainable PHP code.
Additional Resources
- PHP if statement documentation
- PHP switch statement documentation
- PHP match expression documentation
By leveraging these conditional statements appropriately, you can ensure your PHP applications are robust and adaptable to varying conditions.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
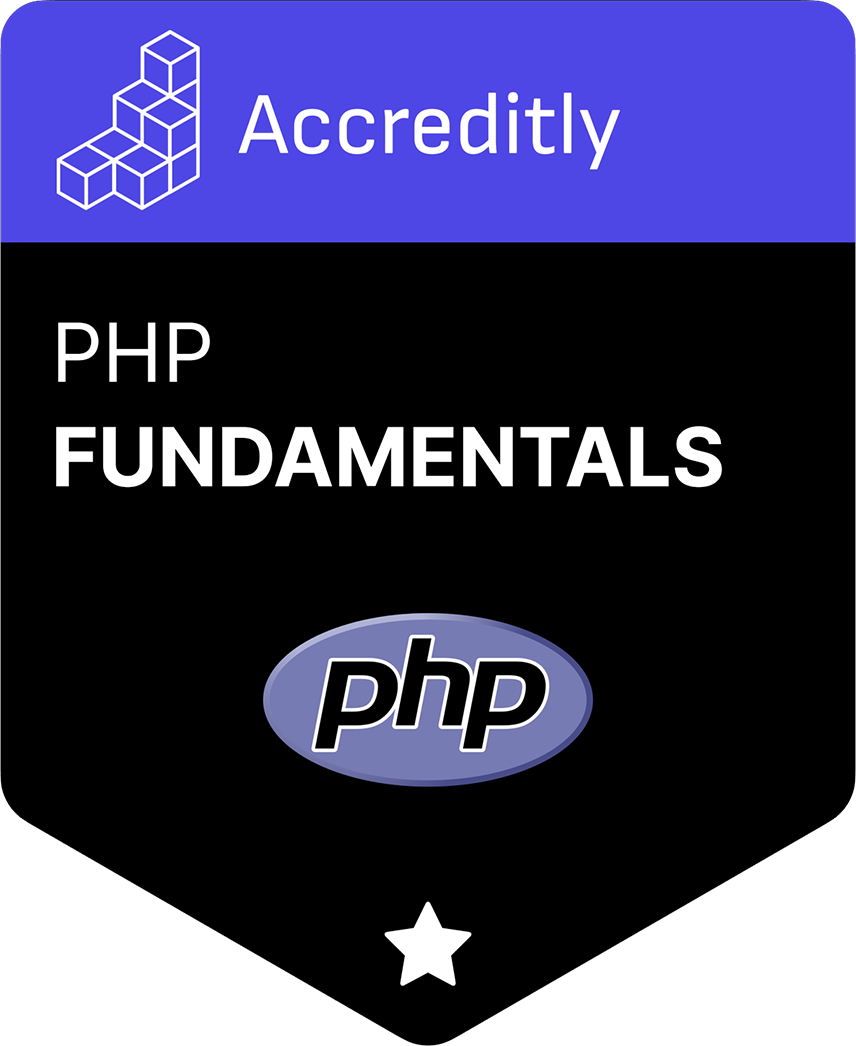