- 1. Importance of Visual Testing
- 2. Tools for Visual Testing
- 3. Setting Up Visual Testing with Puppeteer
- 4. Best Practices
- 5. Challenges and Limitations
Visual testing is a quality assurance activity used to detect differences in the UI appearance of websites. With the proliferation of devices, browsers, and screen sizes, ensuring consistent UI presentation becomes a challenge. JavaScript, being the language of the web, provides several tools and frameworks to simplify visual testing. This article sheds light on the importance and the process of visually testing websites using JavaScript.
1. Importance of Visual Testing
Before diving into the 'how-to', let's understand the 'why'.
-
Ensuring Consistency: Visual testing guarantees that the user experience remains consistent across different environments.
-
Quick Feedback Loop: Automated visual tests provide immediate feedback, allowing for rapid development and bug fixes.
-
Efficient Scaling: As a site grows, manual visual tests become impractical. Automation helps in scaling the testing process.
2. Tools for Visual Testing
JavaScript boasts an extensive ecosystem for visual testing. Some renowned tools include:
-
Puppeteer: A Google Chrome-endorsed tool that allows for headless browsing and screenshot testing.
-
Cypress: While it's primarily an end-to-end testing tool, Cypress offers capabilities for visual testing when integrated with plugins like
cypress-image-snapshot
. -
Storybook: Primarily used for component-driven development, it has plugins that support visual testing of components.
3. Setting Up Visual Testing with Puppeteer
For the sake of this guide, let's use Puppeteer as our primary tool.
Step 1: Install Puppeteer:
npm install puppeteer
Step 2: Write a basic visual test:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://yourwebsite.com');
await page.screenshot({ path: 'screenshot.png' });
await browser.close();
})();
This script launches a browser, captures a screenshot, and saves it as screenshot.png
.
pixelmatch
or looks-same
):
Step 3: Implement image comparison (using tools like By comparing the current screenshot to a baseline, you can identify visual regressions. Two tools to do this are:
- pixelmatch, a tool from Mapbox to detect differences in images based on a defined threshold.
- looks-same, similar to Mapbox's pixelmatch tool, but with some extra options.
4. Best Practices
-
Baseline Management: Regularly update the reference images (baselines) and store them using version control.
-
Responsive Testing: Ensure tests account for various viewport sizes to cover different devices.
-
Continuous Integration: Integrate visual tests within the CI/CD pipeline for real-time feedback.
5. Challenges and Limitations
-
Flakiness: Visual tests can sometimes be flaky due to minor pixel differences, dynamic content, or animations.
-
Maintenance Overhead: With UI updates, baselines need regular updating which can be labor-intensive.
Visual testing ensures your website appears as intended across different environments. While challenges exist, the benefits of detecting UI regressions early in the development cycle are invaluable. With tools like Puppeteer and the vast JavaScript ecosystem, setting up a visual testing workflow is more accessible than ever. Embrace visual testing and ensure a consistent and visually appealing user experience for your audience.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
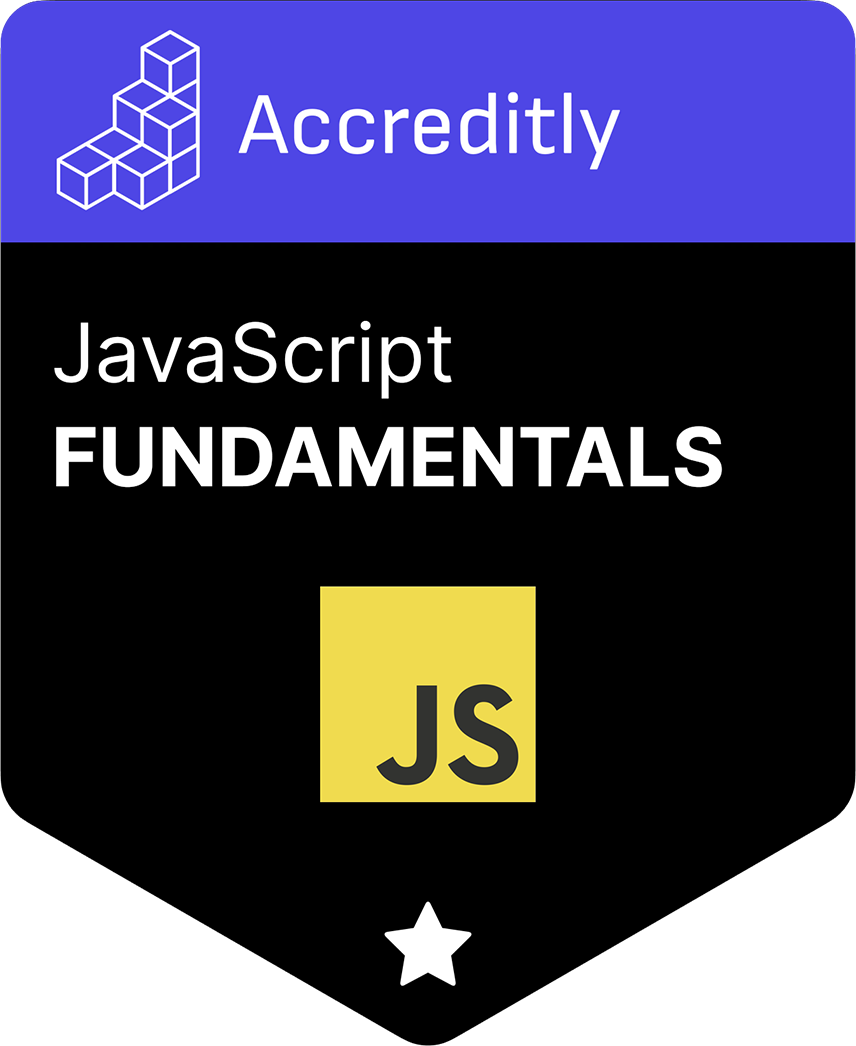