- 1. Understanding Chrome Extensions
- 2. Creating a Basic Chrome Extension
- 3. Adding More Features: Background and Content Scripts
- 4. Example: A Simple Dark Mode Extension
- 5. Deploying Your Extension to the Chrome Web Store
- 6. Installing an Extension Locally
- 7. Advanced Use Cases
Chrome extensions are powerful tools that allow users to extend the functionality of their browser, automate tasks, and integrate services. Whether you're building a productivity tool, a UI enhancer, or even a simple theme, Chrome extensions are a fantastic way to customize browsing experiences.
In this article, we’ll go step by step through building a Chrome extension, covering:
- How Chrome extensions work
- Creating a basic extension
- Adding advanced features (background scripts, content scripts, and storage)
- Deploying your extension to the Chrome Web Store
- Installing locally for private use
- Various real-world use cases
Let’s get started!
1. Understanding Chrome Extensions
A Chrome extension is essentially a small web app running in the browser. It consists of a set of files including:
- A manifest.json file (metadata and permissions)
- JavaScript (logic and interactivity)
- HTML/CSS (UI elements)
- Optional assets (icons, images)
Chrome extensions can:
- Modify webpage content
- Run in the background
- Use Chrome’s built-in APIs
- Communicate with websites and storage
Here’s the basic structure of a Chrome extension:
my-extension/
│── manifest.json
│── popup.html
│── popup.js
│── background.js
│── content.js
│── icons/
│ ├── icon16.png
│ ├── icon48.png
│ ├── icon128.png
Now, let’s build one from scratch.
2. Creating a Basic Chrome Extension
Step 1: Set Up the Files
Create a new folder for your extension (e.g., my-first-extension
) and add a manifest.json
file.
Step 2: Write the Manifest File
The manifest.json
is the heart of your extension. Let’s start with a simple popup extension:
{
"manifest_version": 3,
"name": "My First Chrome Extension",
"version": "1.0",
"description": "A simple Chrome extension with a popup.",
"action": {
"default_popup": "popup.html",
"default_icon": {
"16": "icons/icon16.png",
"48": "icons/icon48.png",
"128": "icons/icon128.png"
}
}
}
Step 3: Create the Popup HTML
Create a popup.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<title>My Extension</title>
<style>
body { width: 200px; text-align: center; font-family: Arial, sans-serif; }
button { margin-top: 10px; }
</style>
</head>
<body>
<h3>Hello!</h3>
<button id="click-me">Click me</button>
<script src="popup.js"></script>
</body>
</html>
Step 4: Add Popup JavaScript
Create popup.js
:
document.getElementById("click-me").addEventListener("click", () => {
alert("You clicked the button!");
});
Step 5: Load Your Extension in Chrome
- Open Chrome and go to
chrome://extensions/
. - Enable Developer mode (top-right corner).
- Click Load unpacked and select your extension folder.
Now, when you click on the extension icon, a popup appears with a button.
3. Adding More Features: Background and Content Scripts
Background Scripts
Background scripts run independently and are used for:
- Managing events
- Listening for browser actions
- Handling alarms and notifications
Update manifest.json
to include a background script:
"background": {
"service_worker": "background.js"
},
"permissions": ["storage", "tabs"]
Create background.js
:
chrome.runtime.onInstalled.addListener(() => {
console.log("Extension Installed");
});
Content Scripts
Content scripts modify web pages dynamically.
Add this to manifest.json
:
"content_scripts": [
{
"matches": ["<all_urls>"],
"js": ["content.js"]
}
]
Create content.js
:
document.body.style.border = "5px solid red";
Now, whenever you load a webpage, it will have a red border!
4. Example: A Simple Dark Mode Extension
A popular use case is dark mode for websites.
manifest.json
Step 1: Update {
"manifest_version": 3,
"name": "Dark Mode Toggle",
"version": "1.0",
"description": "Toggle dark mode on any website",
"permissions": ["storage"],
"action": {
"default_popup": "popup.html"
},
"content_scripts": [
{
"matches": ["<all_urls>"],
"js": ["content.js"]
}
]
}
popup.html
Step 2: Create <!DOCTYPE html>
<html lang="en">
<head>
<title>Dark Mode</title>
</head>
<body>
<button id="toggle-dark-mode">Toggle Dark Mode</button>
<script src="popup.js"></script>
</body>
</html>
popup.js
Step 3: Create document.getElementById("toggle-dark-mode").addEventListener("click", () => {
chrome.tabs.query({ active: true, currentWindow: true }, (tabs) => {
chrome.scripting.executeScript({
target: { tabId: tabs[0].id },
function: toggleDarkMode
});
});
});
function toggleDarkMode() {
document.body.style.backgroundColor = document.body.style.backgroundColor === "black" ? "white" : "black";
document.body.style.color = document.body.style.color === "white" ? "black" : "white";
}
Now, clicking the button toggles dark mode on any webpage.
5. Deploying Your Extension to the Chrome Web Store
Step 1: Prepare Your Extension
- Ensure all files are correct.
- Add icons (16x16, 48x48, 128x128).
Step 2: Create a ZIP File
Compress your extension folder (my-extension.zip
).
Step 3: Submit to the Chrome Web Store
- Go to the Chrome Developer Dashboard.
- Click "Add a New Item".
- Upload the ZIP file.
- Fill out details, permissions, and privacy policy.
- Pay the $5 registration fee.
- Submit for review.
6. Installing an Extension Locally
For personal use, load the extension manually:
- Open
chrome://extensions/
- Enable Developer mode
- Click Load unpacked
- Select your folder
Your extension is now installed!
7. Advanced Use Cases
- Ad Blocker: Remove ads dynamically from pages.
- Clipboard Manager: Store copied texts.
- Weather Widget: Display weather info in a popup.
- AI Chatbot Integration: Connect with OpenAI’s API.
- Productivity Timer: Pomodoro-style focus timer.
Building a Chrome extension is easier than you think, and they're not really any more than a basic web page with some elevated permissions. With just HTML, CSS, and JavaScript, you can create powerful tools that enhance the browsing experience.
From basic popup extensions to background scripts and content scripts, the possibilities are endless. Try building your own and deploy it to the Chrome Web Store or use it locally.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
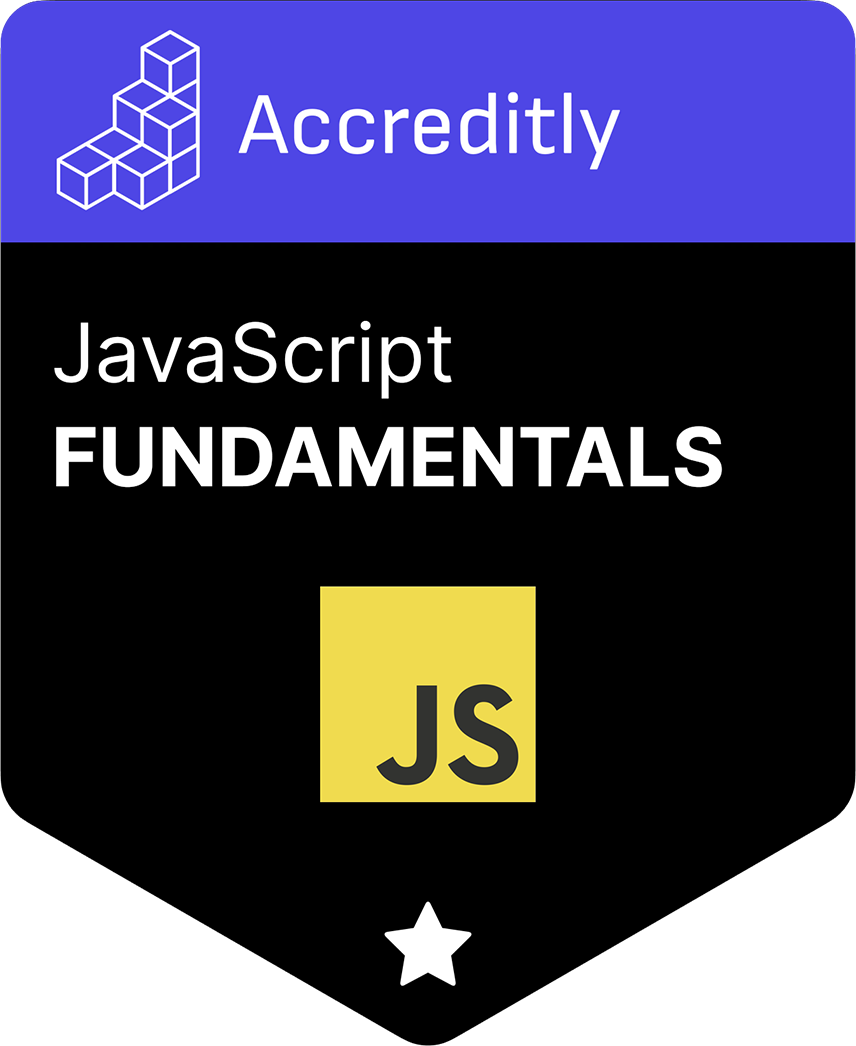