- Understanding Laravel's Pluralizer
- Key Components of the Pluralizer
- How the Pluralizer Works
- Detailed Inner Workings
- Handling Edge Cases
- Pluralizer
Laravel, one of the most popular PHP frameworks, is known for its elegance and simplicity. Among its many features, Laravel provides a handy utility called the pluralizer. This tool is part of Laravel’s Inflector component, used for converting English words from singular to plural and vice versa. But how does it actually work? Let's explore the inner workings of Laravel's pluralizer and see it in action with various examples.
Understanding Laravel's Pluralizer
Laravel's pluralizer is a part of the broader Inflector class, which is responsible for various string transformations. This includes tasks such as pluralization, singularization, and camel casing. The pluralizer, specifically, leverages a set of rules to handle the transformation of words from singular to plural form.
The primary logic of the pluralizer resides in the Illuminate\Support\Pluralizer
class. The pluralization process involves matching words against a predefined set of regular expressions and rules that determine how a word should be pluralized.
Key Components of the Pluralizer
1. Rules and Patterns
The pluralizer uses a set of regular expressions to identify patterns in words. These patterns help the pluralizer determine the correct plural form. For instance, it knows that words ending in "y" often transform by replacing the "y" with "ies" (e.g., "baby" to "babies").
2. Irregular Words
Some words do not follow the standard pluralization rules. Words like "child" and "person" have irregular plurals ("children" and "people"). Laravel's pluralizer includes a list of these irregular words to handle them correctly.
3. Uncountable Words
Certain words have the same form in both singular and plural (e.g., "sheep," "fish"). These words are classified as uncountable, and the pluralizer has a dedicated list to ensure they are not altered incorrectly.
4. The Plural Method
The core method in the pluralizer is the plural
method. This method takes a singular word as input and returns its plural form. The method processes the word through various rules and exceptions to determine the correct plural form.
How the Pluralizer Works
Let's take a closer look at how the pluralizer processes words with different examples.
Example 1: Regular Pluralization
For regular pluralization, the pluralizer applies predefined rules. Here’s how it works:
use Illuminate\Support\Str;
echo Str::plural('cat'); // cats
echo Str::plural('dog'); // dogs
echo Str::plural('car'); // cars
In this example, the words "cat," "dog," and "car" follow a simple rule: add "s" to form the plural.
Example 2: Words Ending in "y"
Words ending in "y" typically replace the "y" with "ies" to form the plural:
echo Str::plural('baby'); // babies
echo Str::plural('lady'); // ladies
echo Str::plural('city'); // cities
Example 3: Irregular Words
Irregular words have specific plural forms that the pluralizer recognizes:
echo Str::plural('child'); // children
echo Str::plural('person'); // people
echo Str::plural('man'); // men
Example 4: Uncountable Words
Uncountable words remain unchanged in their plural form:
echo Str::plural('sheep'); // sheep
echo Str::plural('fish'); // fish
echo Str::plural('deer'); // deer
Example 5: Words Ending in "f" or "fe"
Some words ending in "f" or "fe" change to "ves" in their plural form:
echo Str::plural('wolf'); // wolves
echo Str::plural('life'); // lives
echo Str::plural('knife'); // knives
Detailed Inner Workings
To understand the inner workings, let's delve into the plural
method in the Pluralizer
class:
plural
Method
The The plural
method starts by checking if the word is uncountable. If it is, the method returns the word unchanged:
public static function plural($value, $count = 2)
{
if ((int) $count === 1 || static::uncountable($value)) {
return $value;
}
// Other processing...
}
Checking for Irregular Words
Next, the method checks if the word is irregular:
foreach (static::$irregular as $pattern => $result) {
$pattern = '/' . $pattern . '$/i';
if (preg_match($pattern, $value)) {
return preg_replace($pattern, $result, $value);
}
}
Applying Regular Rules
If the word is neither uncountable nor irregular, the method proceeds to apply regular pluralization rules:
foreach (static::$plural as $pattern => $result) {
if (preg_match($pattern, $value)) {
return preg_replace($pattern, $result, $value);
}
}
These rules are defined in arrays that contain patterns and their corresponding replacements. For example:
protected static $plural = [
'/(quiz)$/i' => "$1zes",
'/^(ox)$/i' => "$1en",
'/([m|l])ouse$/i' => "$1ice",
'/(matr|vert|ind)(?:ix|ex)$/i' => "$1ices",
// More rules...
];
Handling Edge Cases
Compound Words
The pluralizer can handle compound words by applying the pluralization rules to the appropriate part of the word. For instance:
echo Str::plural('mother-in-law'); // mothers-in-law
Custom Pluralization
Developers can extend or modify the pluralization rules to handle specific cases not covered by the default rules. Laravel allows you to customize the inflection rules by modifying the Inflector
configuration.
Pluralizer
Laravel's pluralizer is a powerful tool that simplifies the task of word inflection in applications. By understanding its inner workings, you can better appreciate its capabilities and leverage it effectively in your projects. Whether dealing with regular plural forms, irregular words, or uncountable nouns, Laravel's pluralizer has got you covered.
For more details on how to use and customize Laravel's pluralizer, you can refer to the official documentation. This deep dive into the pluralizer should give you a solid foundation to handle word transformations in your Laravel applications efficiently.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
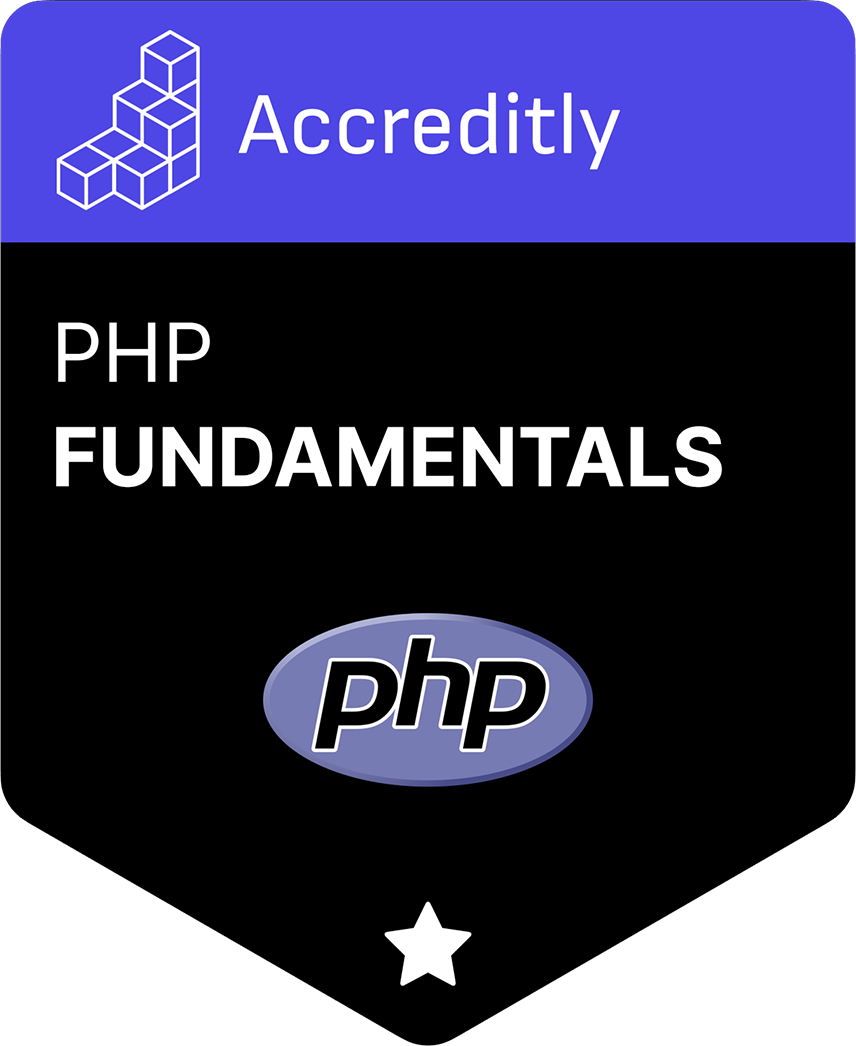