- Step 1: Setting Up JWT Authentication
- Step 2: Creating Authentication Routes
- Step 3: Protecting Routes
- Step 4: Testing Your Protected Routes
In modern web applications, securing routes is paramount to ensuring that resources are only accessible to authenticated and authorized users. JSON Web Tokens (JWT) provide a robust and scalable method to secure routes by ensuring that every request to a server is accompanied by a valid token that can be verified and trusted. Laravel, being a powerful PHP framework for web artisans, facilitates easy integration of JWT-based authentication for its routing system. This guide will walk you through setting up JWT authentication in Laravel and using it to protect your routes.
Step 1: Setting Up JWT Authentication
Before you can protect routes using JWT, you need to integrate JWT authentication into your Laravel application. We'll use the tymon/jwt-auth
package, which is a popular package that provides JWT functionality for Laravel.
Installing the Package
-
Install the package via Composer:
composer require tymon/jwt-auth
-
Publish the configuration file:
php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\LaravelServiceProvider"
-
Generate a new secret key that will be used to sign your tokens:
php artisan jwt:secret
This command updates your
.env
file with a new JWT secret key.
Configure the Auth Guard
Edit your config/auth.php
to set up a guard that uses the jwt
driver:
'guards' => [
'api' => [
'driver' => 'jwt',
'provider' => 'users',
],
],
Make sure you have a provider that manages the users in your application. Typically, it's configured to use the Eloquent User model by default.
Step 2: Creating Authentication Routes
In routes/api.php
, define routes for user authentication. You'll need at least two routes: one for user login and another for user logout.
Route::post('login', 'AuthController@login');
Route::post('logout', 'AuthController@logout')->middleware('auth:api');
Implementing the AuthController
Create an AuthController
that handles login and logout functionality:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use App\Models\User;
class AuthController extends Controller
{
public function login(Request $request)
{
$credentials = $request->only(['email', 'password']);
if (!$token = Auth::guard('api')->attempt($credentials)) {
return response()->json(['error' => 'Unauthorized'], 401);
}
return $this->respondWithToken($token);
}
public function logout()
{
Auth::guard('api')->logout();
return response()->json(['message' => 'Successfully logged out']);
}
protected function respondWithToken($token)
{
return response()->json([
'access_token' => $token,
'token_type' => 'bearer',
'expires_in' => Auth::guard('api')->factory()->getTTL() * 60
]);
}
}
Step 3: Protecting Routes
Now that you have JWT authentication set up, you can protect your routes by using the auth:api
middleware, which utilizes the JWT guard.
Route::group(['middleware' => ['auth:api']], function() {
Route::get('protected', 'ProtectedController@index');
});
Any route within this group requires a valid JWT in the Authorization
header of the request to be accessed.
Step 4: Testing Your Protected Routes
Use a REST client like Postman or cURL to test your protected routes. Ensure that requests without a valid JWT token are denied access, and requests with a valid token in the Authorization
header are granted access.
Securing your Laravel routes using JWT tokens is an effective way to protect sensitive data and services from unauthorized access. By leveraging the tymon/jwt-auth
package, you can implement a robust, stateless authentication system that scales with your application's needs. Always ensure to keep your JWT secret key secure and regularly update your authentication logic as needed to address any emerging security concerns.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
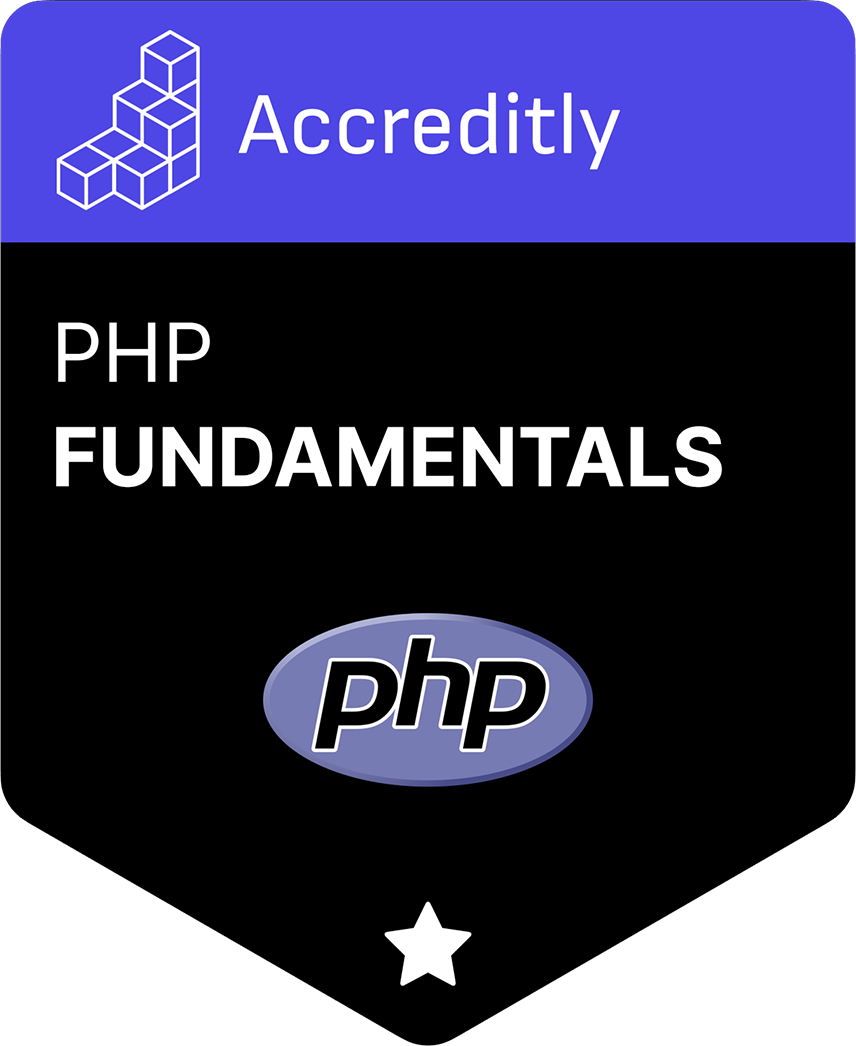