- Understanding env() and config()
- Reasons to Avoid Using env() in Application Code
- Best Practices for Using config()
- Example: Refactoring Code to Use config()
- Key points
Laravel is a powerful and flexible PHP framework that provides a variety of tools to help developers build robust applications. One such tool is the ability to manage environment variables using the .env
file. However, a common mistake made by developers is directly using the env()
function throughout their application code. While env()
is useful, it should be limited to configuration files only. This article explores the reasons why you shouldn't use env()
in your Laravel app and why you should use config()
instead.
env()
and config()
Understanding env()
The env()
function in Laravel is used to retrieve values from the .env
file. This file contains key-value pairs that define environment-specific settings, such as database credentials, API keys, and other configuration details.
$databaseHost = env('DB_HOST', 'localhost');
config()
The config()
function, on the other hand, is used to access values from the configuration files stored in the config
directory. These files aggregate environment variables and other settings into a structured format that is easier to manage and more performant.
$databaseHost = config('database.connections.mysql.host');
env()
in Application Code
Reasons to Avoid Using 1. Caching Configuration
One of the primary reasons to avoid using env()
in your application code is related to caching. Laravel provides a command to cache all configuration values into a single file for faster performance.
php artisan config:cache
When you cache your configuration, Laravel reads all environment variables and configuration values and stores them in a single, efficient file. If you use env()
directly in your code, those values will not be cached, potentially leading to inconsistent behavior and performance issues.
Refer to the Laravel documentation on Configuration Caching for more details.
2. Security Concerns
Using env()
directly in your application logic can inadvertently expose sensitive information. Environment variables are meant to be accessed only during the bootstrapping of the application. By using config()
, you ensure that sensitive data is accessed in a controlled manner through the configuration files.
// Not recommended
$apiKey = env('API_KEY');
// Recommended
$apiKey = config('services.api.key');
3. Consistency and Maintainability
Accessing environment variables directly through env()
scattered across your application code makes it harder to maintain and manage configurations. By centralizing the environment variables in configuration files and accessing them using config()
, you ensure that your codebase is consistent and easier to maintain.
// Not recommended
if (env('APP_DEBUG')) {
// Debug mode logic
}
// Recommended
if (config('app.debug')) {
// Debug mode logic
}
4. Testing and Environment Management
When running tests, Laravel allows you to override configuration values easily. If you use env()
directly in your application code, these overrides might not work as expected, leading to unreliable tests. Using config()
ensures that your tests are accurate and that you can easily switch between different environments.
Refer to the Laravel documentation on Environment Configuration for more details.
config()
Best Practices for Using Define Configuration Values in Config Files
Always define your environment-specific settings in the configuration files located in the config
directory. This centralizes your configuration and makes it easier to manage.
// config/services.php
return [
'api' => [
'key' => env('API_KEY'),
],
];
config()
Access Configuration Values Using Use the config()
function to retrieve these values in your application code. This ensures that the values are cached and accessed efficiently.
$apiKey = config('services.api.key');
Use Defaults Where Necessary
Provide default values in your configuration files to handle cases where environment variables are not set. This ensures that your application can still function with reasonable defaults.
// config/app.php
return [
'debug' => env('APP_DEBUG', false),
];
config()
Example: Refactoring Code to Use Let's look at an example of refactoring application code to use config()
instead of env()
.
Before Refactoring
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class ApiController extends Controller
{
public function getApiData()
{
$apiKey = env('API_KEY');
$apiUrl = env('API_URL');
// Use $apiKey and $apiUrl to fetch data from the API
}
}
After Refactoring
First, define the environment variables in a configuration file:
// config/services.php
return [
'api' => [
'key' => env('API_KEY'),
'url' => env('API_URL'),
],
];
Then, update your controller to use config()
:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class ApiController extends Controller
{
public function getApiData()
{
$apiKey = config('services.api.key');
$apiUrl = config('services.api.url');
// Use $apiKey and $apiUrl to fetch data from the API
}
}
Key points
While env()
is a useful function in Laravel, it should be limited to configuration files to ensure optimal performance, security, and maintainability. Using config()
to access environment variables in your application code ensures that your settings are cached, consistent, and easier to manage.
For more information, refer to the Laravel Configuration Documentation. By following these best practices, you can build more robust and maintainable Laravel applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
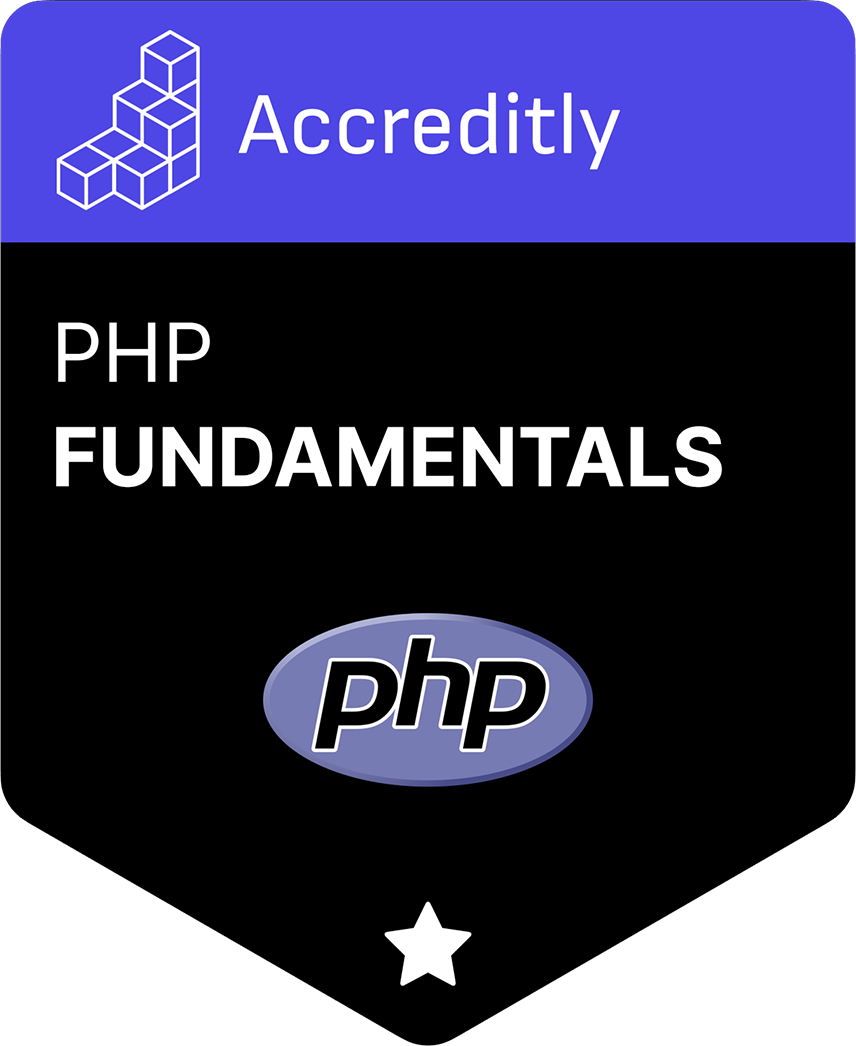