In database management, it's not uncommon to encounter situations where you need to perform operations over a range of dates. Whether it's for financial reports, analytics, or activity logs, having a structured way to access dates can be incredibly helpful. One efficient method to handle such situations in MySQL is to use a Date Table. This article will walk you through creating and utilizing a date table effectively.
What is a Date Table?
A date table, often referred to as a calendar table or a date dimension table, is a separate table in your database that contains a list of dates. The table generally includes one row for each day, making it easier to run date-based queries without missing out on any dates.
Why Use a Date Table?
-
Continuous Dates: There might be missing dates in your primary data, but with a date table, you can ensure that you have a continuous sequence of dates.
-
Efficiency: Joining with a date table can be faster and more straightforward than generating date series on-the-fly, especially for large datasets.
-
Flexibility: Date tables can include additional columns, such as flags for weekends or holidays, making certain queries more straightforward.
Creating a Date Table:
Let's start by creating a basic date table in MySQL:
CREATE TABLE date_table (
date DATE PRIMARY KEY
);
Now, to populate the table with dates, you can use a stored procedure:
DELIMITER //
CREATE PROCEDURE FillDateTable(startDate DATE, endDate DATE)
BEGIN
WHILE startDate <= endDate DO
INSERT INTO date_table (date) VALUES (startDate);
SET startDate = DATE_ADD(startDate, INTERVAL 1 DAY);
END WHILE;
END //
DELIMITER ;
-- Invoke the procedure
CALL FillDateTable('2000-01-01', '2030-12-31');
This procedure will populate your date_table with dates from January 1, 2000, to December 31, 2030. Adjust the range according to your needs.
Querying Using the Date Table:
Now that we have a populated date table, we can use it in our queries. Let's assume we have a sales
table, and we want to fetch the total sales for every day, even if there were no sales on certain days:
SELECT
dt.date,
COALESCE(SUM(s.amount), 0) as total_sales
FROM
date_table dt
LEFT JOIN
sales s ON dt.date = s.sale_date
WHERE
dt.date BETWEEN '2020-01-01' AND '2020-12-31'
GROUP BY
dt.date
ORDER BY
dt.date;
In this example, the LEFT JOIN
ensures that we get a result for every day in 2020, even if there were no sales on that date. Days without sales will show a total_sales
value of 0, thanks to the COALESCE
function.
Date tables can be a powerful tool in your SQL toolkit. They provide a simple way to ensure continuous date ranges, make date-based queries more efficient, and offer a flexible structure for additional date-related information. By integrating a date table into your database, you can streamline many common querying tasks and enhance the accuracy of your results.
Interested in proving your knowledge of this topic? Take the Database Fundamentals certification.
Database Fundamentals
Focusing on SQL and database design, this exam will test your knowledge of database fundamentals.
$99
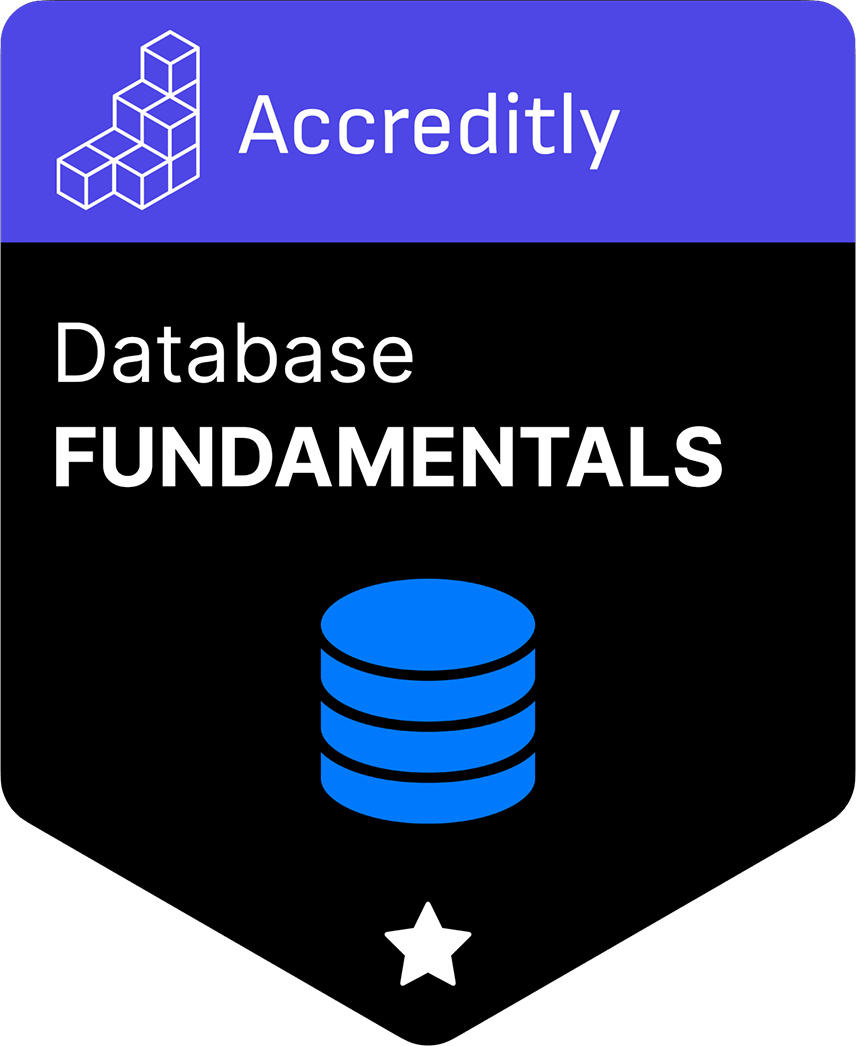