- Understanding User Roles and Capabilities
- Step 1: Create a Custom User Role
- Step 2: Assign Capabilities to the Custom User Role
- Step 3: Add Custom Capabilities
- Step 4: Check for Custom Capabilities
- Conclusion
In WordPress, user roles provide a convenient way to manage access and permissions for different types of users. By default, WordPress comes with five user roles: Administrator, Editor, Author, Contributor, and Subscriber. However, sometimes you may want to create custom user roles to better suit your website's needs. In this post, we will discuss how to add user roles programmatically and how to assign capabilities to those roles. We will also provide code examples and links to the official documentation for further reference.
Understanding User Roles and Capabilities
Before diving into the code, it's essential to understand the concepts of user roles and capabilities in WordPress. A user role is a collection of capabilities that define what actions a user can perform on your website. Capabilities are individual permissions that allow users to perform specific tasks, such as editing posts, publishing pages, or managing plugins.
Learn more about user roles and capabilities in the WordPress Codex.
Step 1: Create a Custom User Role
To create a custom user role, you will need to use the add_role()
function provided by WordPress. This function takes three arguments: the role's unique identifier (slug), the role's display name, and an array of capabilities.
Here's an example of how to create a custom user role called "Content Manager":
function add_custom_user_role() {
add_role(
'content_manager',
'Content Manager',
array(
'read' => true,
'edit_posts' => true,
'delete_posts' => true,
'publish_posts' => true,
'upload_files' => true,
)
);
}
add_action('init', 'add_custom_user_role');
In the example above, we created a new user role called "Content Manager" with the capabilities to read, edit, delete, and publish posts, as well as upload files.
Step 2: Assign Capabilities to the Custom User Role
After creating a custom user role, you may want to assign additional capabilities or modify existing ones. You can do this using the add_cap()
and remove_cap()
functions.
Here's an example of how to assign a new capability to the "Content Manager" role:
function add_capability_to_content_manager() {
$role = get_role('content_manager');
$role->add_cap('edit_others_posts');
}
add_action('init', 'add_capability_to_content_manager');
In the example above, we used the get_role()
function to retrieve the "Content Manager" role and then added the edit_others_posts
capability using the add_cap()
function.
To remove a capability from a role, you can use the remove_cap()
function:
function remove_capability_from_content_manager() {
$role = get_role('content_manager');
$role->remove_cap('delete_posts');
}
add_action('init', 'remove_capability_from_content_manager');
In this example, we removed the delete_posts
capability from the "Content Manager" role.
Step 3: Add Custom Capabilities
If the default WordPress capabilities do not meet your requirements, you can create custom capabilities and assign them to your user roles.
Here's an example of how to create a custom capability and assign it to the "Content Manager" role:
function add_custom_capability() {
$role = get_role('content_manager');
$role->add_cap('manage_custom_content');
}
add_action('init', 'add_custom_capability');
In the example above, we added a custom capability called manage_custom_content to the "Content Manager" role.
Step 4: Check for Custom Capabilities
Once you've added custom capabilities, you may want to check if a user has the required capabilities to perform certain actions. You can use the current_user_can()
function to check if the current user has a specific capability.
Here's an example of how to check for the manage_custom_content
capability:
if (current_user_can('manage_custom_content')) {
// The current user has the 'manage_custom_content' capability.
// Perform the action here.
} else {
// The current user does not have the required capability.
// Show an error message or redirect to a different page.
}
Conclusion
In this tutorial, we've explored how to add user roles programmatically in WordPress, assign capabilities to those roles, and create custom capabilities. By creating custom user roles and capabilities, you can better manage access and permissions for different types of users on your website. Be sure to review the official documentation on roles and capabilities to learn more about the available default capabilities and how to work with them effectively.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
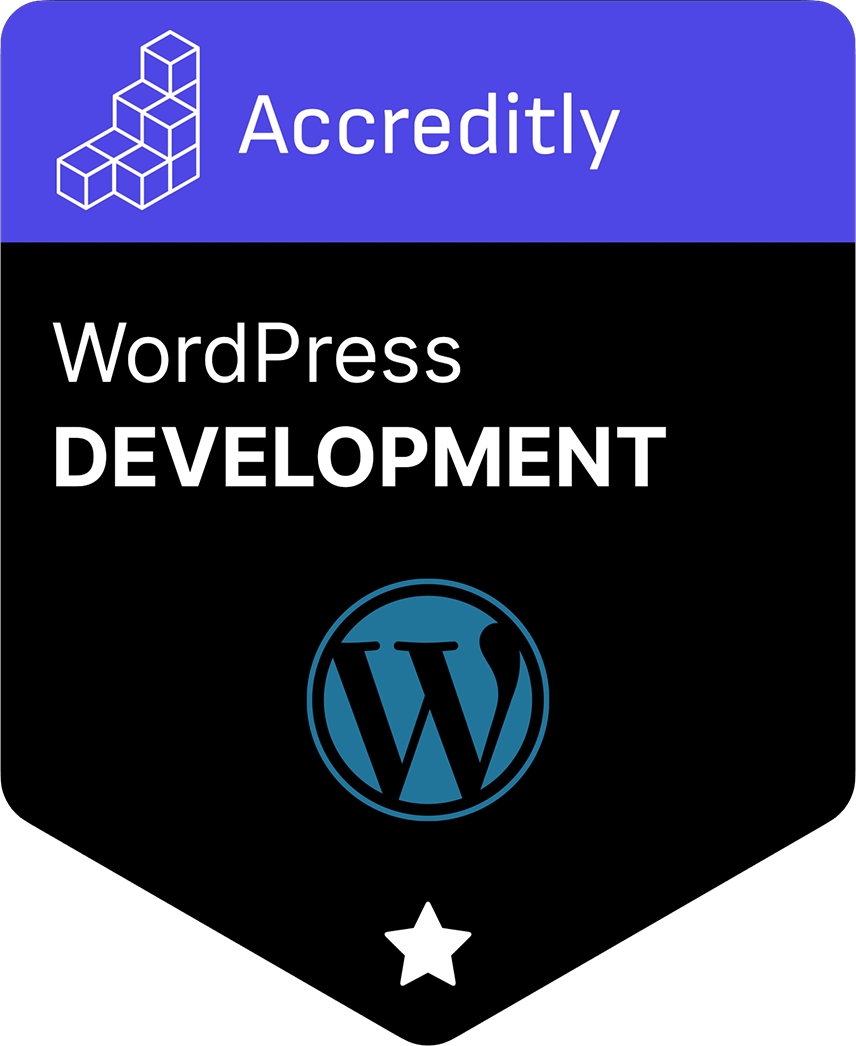