- Understanding Global Scopes
- Creating a Global Scope
- Applying Global Scope to a Model
- Removing Global Scopes
- Conclusion
Global scopes in Laravel allow you to add constraints to all queries for a given model, providing a convenient way to make sure every query for that model includes certain criteria. This tutorial will walk you through how to add a global scope to all queries on a model in Laravel.
Understanding Global Scopes
In Laravel, a global scope is used to constrain all queries for a given Eloquent model. This is particularly useful when you want to ensure that all retrieved models meet certain criteria by default.
Creating a Global Scope
To create a global scope, you should define a class that implements the Illuminate\Database\Eloquent\Scope
interface. This interface requires one method: apply
, which may be used to apply the scope to a given Eloquent\Builder
instance:
<?php
namespace App\Scopes;
use Illuminate\Database\Eloquent\Scope;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Builder;
class AgeScope implements Scope {
/**
* Apply the scope to a given Eloquent query builder.
*
* @param \Illuminate\Database\Eloquent\Builder $builder
* @param \Illuminate\Database\Eloquent\Model $model
* @return void
*/
public function apply(Builder $builder, Model $model) {
$builder->where('age', '>', 200);
}
}
In this example, the AgeScope
global scope is applied to a query builder and constrains the query to only retrieve rows where the age
column is greater than 200
.
Applying Global Scope to a Model
To assign the scope to a model, you should override the booted
method of your model:
<?php
namespace App\Models;
use App\Scopes\AgeScope;
use Illuminate\Database\Eloquent\Model;
class User extends Model {
/**
* The "booted" method of the model.
*
* @return void
*/
protected static function booted() {
static::addGlobalScope(new AgeScope);
}
}
Here, whenever you query the User
model, it will always apply the conditions defined in the AgeScope
global scope.
Removing Global Scopes
To remove a global scope from a model for a query, you can use the withoutGlobalScope
method:
$users = App\Models\User::withoutGlobalScope(AgeScope::class)->get();
This statement retrieves all the User
without applying the AgeScope
global scope.
Conclusion
Global scopes offer an efficient way to apply query constraints to all queries for a given Eloquent model in Laravel. By following the steps outlined in this guide, you can ensure that your global scopes are applied consistently, enhancing the robustness and maintainability of your Laravel applications. Be sure to consider the implications of global scopes and use them judiciously to avoid unexpected behavior in your application's data retrieval logic.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
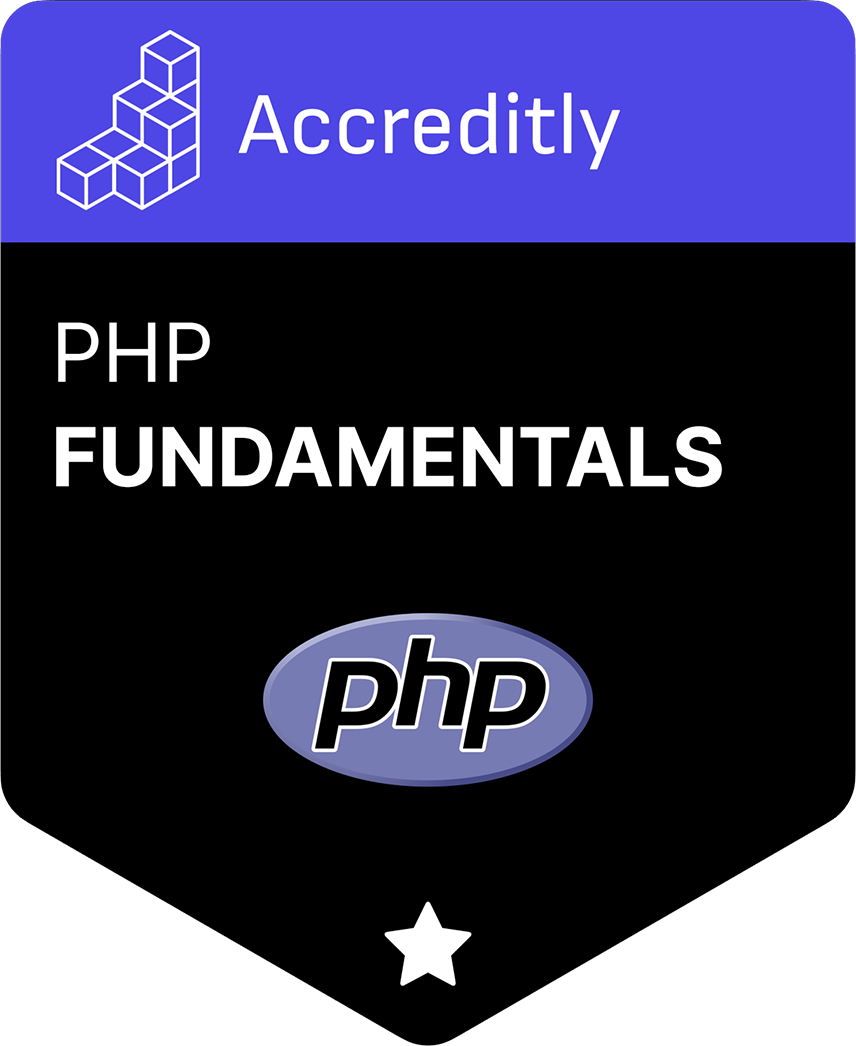