- 1. Understanding Stubs in Laravel:
- 2. Publishing Laravel's Stubs:
- 3. Modifying the Model Stub:
- 4. Modifying the Migration Stub:
- 5. Creating Models and Migrations:
- Rolling Back Changes:
Soft deletes are a powerful feature in Laravel, allowing for "deleted" records to remain in the database but be hidden from normal queries. By default, when creating new models and migrations using Laravel's artisan command, soft deletes are not included. However, by customizing Laravel's stubs, you can alter this behavior.
If you're just looking to enable soft deletes for a single entity, check out the article how to soft delete in Laravel.
1. Understanding Stubs in Laravel:
Laravel uses stubs - templates for various types of classes and files - when scaffolding new classes via Artisan commands. By publishing and modifying these stubs, you can change the default behavior of these commands.
2. Publishing Laravel's Stubs:
Starting from Laravel 7, you can publish the stubs using the following command:
php artisan stub:publish
This will place all of the stubs in a /stubs
directory at your project's root.
3. Modifying the Model Stub:
Navigate to the /stubs
directory, and you will find a file named model.stub
. Open it.
Add the SoftDeletes
trait and the corresponding use declaration:
use Illuminate\Database\Eloquent\SoftDeletes;
class DummyClass extends Model
{
use SoftDeletes;
...
}
4. Modifying the Migration Stub:
Next, let's make sure that our migrations include a deleted_at
column by default. Open the migration.create.stub
in the /stubs
directory.
Add the following line in the up
method:
$table->softDeletes();
Your migration stub should look something like this:
public function up()
{
Schema::create('DummyTable', function (Blueprint $table) {
$table->id();
$table->softDeletes(); // Your soft deletes column
// other columns...
});
}
5. Creating Models and Migrations:
Now, whenever you use the php artisan make:model ModelName -m
command to generate a new model and migration, they will both include soft delete support by default.
Rolling Back Changes:
If at any point you wish to revert to the default Laravel stubs, simply delete the /stubs
directory from your project's root. Laravel will then revert to using its internal stubs.
Customizing Laravel's default scaffolding behavior can save you time and ensure consistency across your application. By modifying stubs, you can tailor the artisan make commands to better suit the specific needs of your project. Always remember to test your changes to ensure they work as expected before pushing them to a production environment.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
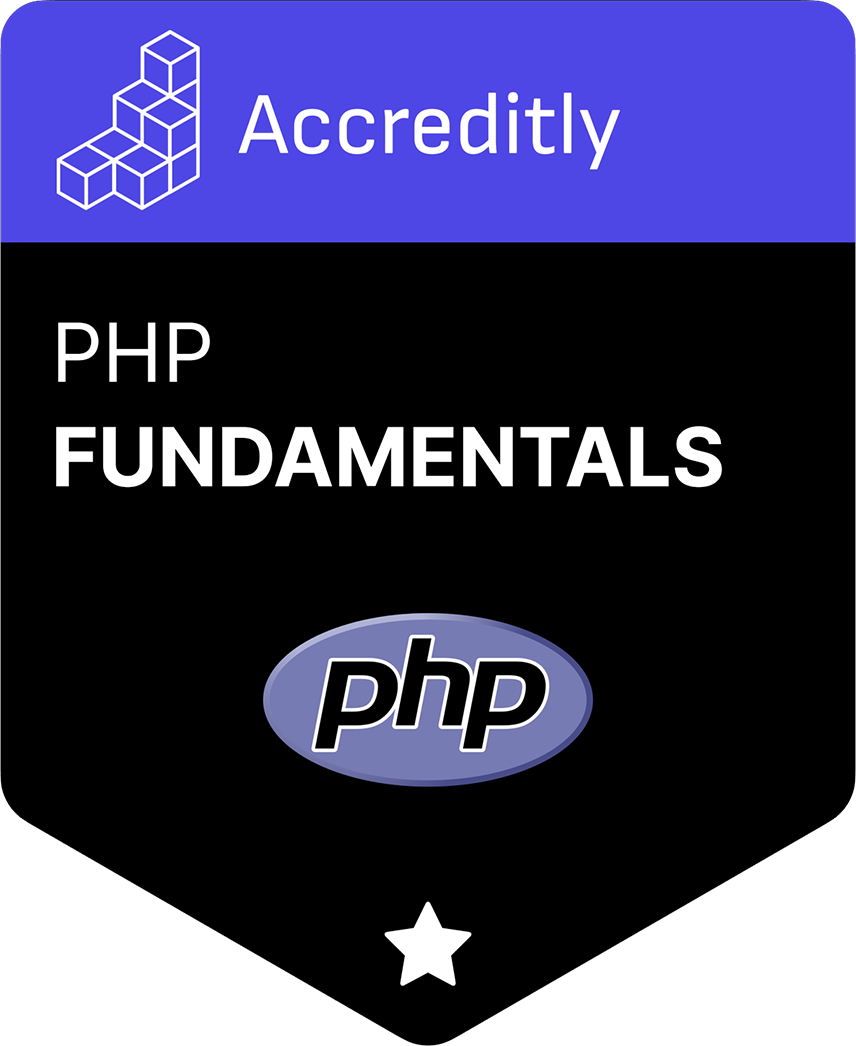