- Why Are Soft Deletes Important?
- When to Use Soft Deletes?
- When Not to Use Soft Deletes?
- Implementing Soft Deletes in Laravel:
- Soft Deleting a Record
- Querying Soft Deleted Records
- Permanently Deleting
Soft deleting is a practice where records are flagged as 'deleted' rather than being removed from the database. This flagged record remains in the database but is hidden from standard queries. Laravel makes implementing soft deletes seamless, offering a built-in solution that allows you to retain data without presenting it in your application.
Why Are Soft Deletes Important?
-
Data Recovery: Accidental deletions happen. Soft deletes act as a safety net, allowing easy recovery of data without resorting to backups.
-
Audit Trail: For regulatory or auditing purposes, you may need a trail of all data, including what was "deleted." Soft deletes help maintain this trail.
-
Relational Integrity: In databases where relationships matter, soft deletes ensure that relational data isn't lost inadvertently.
When to Use Soft Deletes?
-
Critical Data: For data that, once lost, can't be recreated, like user-generated content.
-
E-commerce: In scenarios like order management, you might want to retain a record of every order, even if it's canceled.
-
Regulatory Compliance: When you're legally obligated to retain data for a specific duration.
When Not to Use Soft Deletes?
-
High Volume, Low Impact Data: For frequently changing data with low impact, like cache data or logs, soft deletes may not be necessary. Remember that soft delete queries add a slight overhead.
-
Limited Storage: If storage costs are a concern, retaining every record might not be feasible.
-
Performance: Soft deletes can affect performance as the dataset grows, leading to slower queries.
Implementing Soft Deletes in Laravel:
SoftDeletes
Trait
Step 1: Use the On your Eloquent model, include the SoftDeletes
trait.
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Post extends Model
{
use SoftDeletes;
}
If you're generating a model via the artisan
command you can also add a soft deletes by default by publishing the model stub, but don't forget to remove it if it's not worthwhile on the given model!
deleted_at
Column
Step 2: Add the Your database table needs a deleted_at
column, which Laravel uses to mark a record as 'deleted.'
You can add this column using a migration:
php artisan make:migration add_deleted_at_to_posts_table
In the migration file:
public function up()
{
Schema::table('posts', function (Blueprint $table) {
$table->softDeletes();
});
}
Run the migration:
php artisan migrate
This is adding it separately to the original migration that created the table, you can also just append $table->softDeletes();
to the original migration if it has not been run or submitted to version control yet.
Soft Deleting a Record
Deleting a record will now be a "soft delete":
$post = Post::find(1);
$post->delete();
The record remains in the database, but the deleted_at
column is timestamped.
Querying Soft Deleted Records
To retrieve soft deleted records:
// Retrieve only trashed (soft deleted) records
$posts = Post::onlyTrashed()->get();
// Include trashed records in results
$posts = Post::withTrashed()->get();
To restore a soft-deleted record:
$post->restore();
Permanently Deleting
To permanently delete a record, by-passing the soft deletion:
$post->forceDelete();
Soft deletes are a powerful feature of Laravel, adding an extra layer of data security to applications. By understanding their importance and weighing their pros and cons, you can make informed decisions about when to employ this functionality in your Laravel projects.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
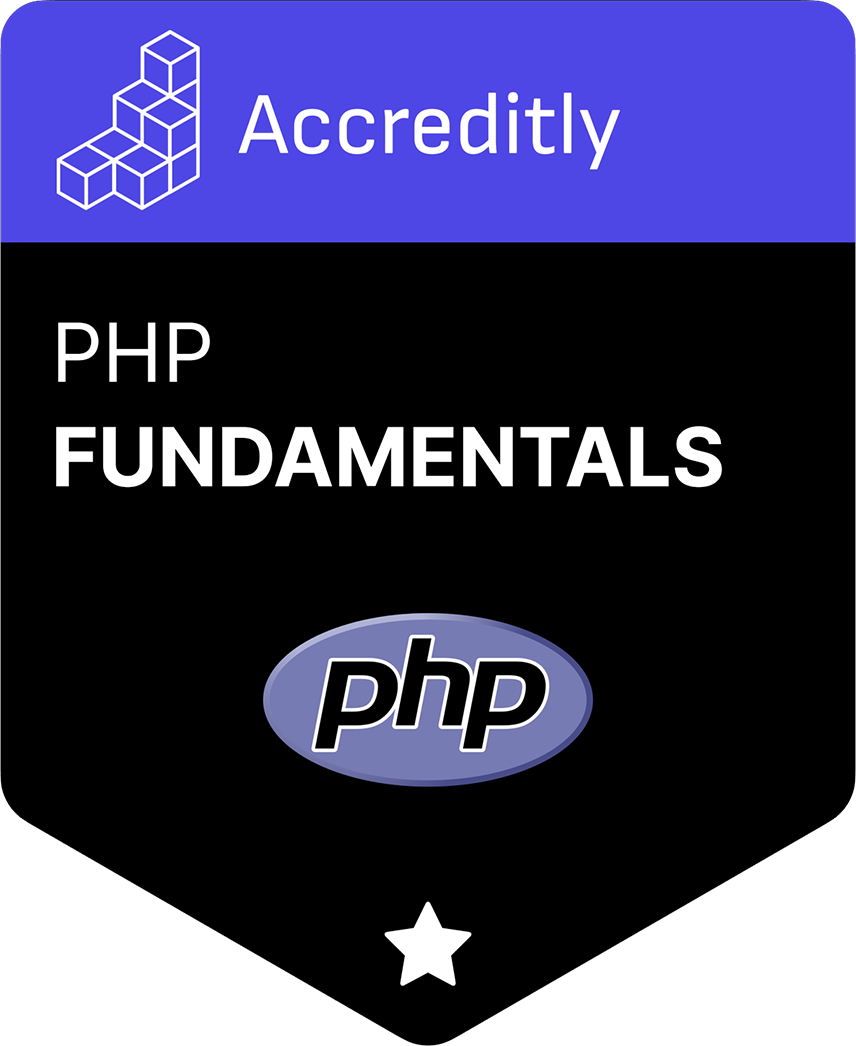