- Understanding WP_Query
- Querying Multiple Post Types
- Including Custom Post Types
- Ordering and Filtering Results
- Paginating Results
- Best Practices
In WordPress, different types of content like posts, pages, and custom post types are fundamental building blocks. There are scenarios where you might want to query and display multiple post types within a single query - for instance, displaying a mix of articles, portfolio items, and testimonials on a homepage. Let's explore how you can efficiently query multiple post types in a single WordPress query.
Understanding WP_Query
WordPress offers WP_Query
, a powerful class that enables you to create custom queries to retrieve posts in various ways. It's flexible enough to handle multiple post types in a single query.
Querying Multiple Post Types
To query multiple post types, you'll use WP_Query
and specify the post types you want in its arguments.
Basic Example:
Here’s how you can query both posts and pages together:
$args = array(
'post_type' => array('post', 'page'),
);
$my_query = new WP_Query($args);
if ($my_query->have_posts()) :
while ($my_query->have_posts()) : $my_query->the_post();
// Display post content
endwhile;
endif;
wp_reset_postdata();
In this example, $args
is an array that defines the query parameters. We specify that we want to retrieve both 'post' and 'page' post types.
Including Custom Post Types
If you've defined custom post types, you can include them in your query just as easily.
Example with Custom Post Types:
Suppose you have a custom post type called 'portfolio'. You can query posts, pages, and portfolio items together:
$args = array(
'post_type' => array('post', 'page', 'portfolio'),
);
$my_query = new WP_Query($args);
// Loop through the posts
Ordering and Filtering Results
You can further customize your query to order or filter the results. For instance, you might want to sort the results by date or include only those in certain categories.
Example with Custom Ordering:
$args = array(
'post_type' => array('post', 'portfolio'),
'orderby' => 'date',
'order' => 'DESC'
);
$my_query = new WP_Query($args);
// Loop through the posts
Paginating Results
If you’re displaying a large number of posts, you might want to paginate the results.
Example with Pagination:
$paged = (get_query_var('paged')) ? get_query_var('paged') : 1;
$args = array(
'post_type' => array('post', 'page'),
'paged' => $paged
);
$my_query = new WP_Query($args);
// Loop through the posts
Best Practices
-
Use
wp_reset_postdata()
: Always reset the post data after a custom query loop to restore the global$post
object. - Be Mindful of Performance: Queries involving multiple post types can be resource-intensive, especially on large databases. Optimize your query and use caching where appropriate.
-
Conditional Tags for Different Types: Inside your loop, use conditional tags like
get_post_type()
to differentiate output based on post type.
Querying multiple post types in a single WordPress query opens up a world of possibilities for displaying varied content types together. By leveraging the power of WP_Query
, you can create complex, dynamic displays of content, tailored to the diverse needs of your website and its audience.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
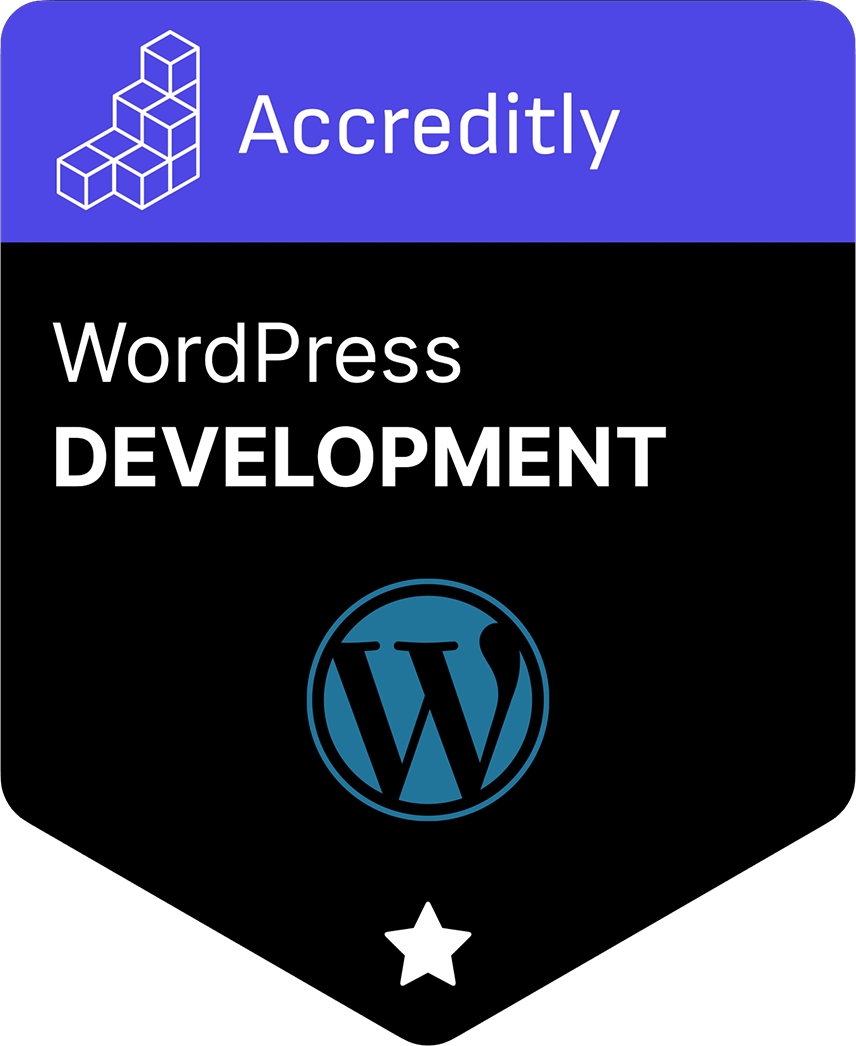