- Understanding the Importance of Sanitization
- Sanitizing Text Inputs
- Sanitizing Email Inputs
- Sanitizing URLs
- Sanitizing Numbers
- Sanitizing HTML Content
- Escaping Output
- Using Nonce for Form Verification
In the world of web development, especially in WordPress, sanitizing input is akin to keeping your digital house clean and orderly. Sanitizing input means scrubbing the data provided by users to ensure it's safe before using it in your website or database. This practice is crucial for preventing security vulnerabilities like SQL injections and cross-site scripting (XSS) attacks. Let's explore how to effectively sanitize input in WordPress.
Understanding the Importance of Sanitization
Input sanitization is about treating all user input as untrusted and potentially harmful. By sanitizing inputs, you ensure that any data used in your site or stored in your database is safe and does not contain malicious code.
Sanitizing Text Inputs
WordPress provides several functions to sanitize text inputs:
-
sanitize_text_field(): This function is ideal for plain text inputs. It strips tags and removes line breaks.
$cleaned_text = sanitize_text_field($_POST['text_input']);
-
sanitize_textarea_field(): Similar to
sanitize_text_field()
, but preserves line breaks, making it suitable for textareas.$cleaned_textarea = sanitize_textarea_field($_POST['textarea_input']);
Sanitizing Email Inputs
To sanitize email addresses, use sanitize_email()
:
$cleaned_email = sanitize_email($_POST['email_input']);
This function ensures the email address is properly formatted and clean.
Sanitizing URLs
For URLs, use esc_url_raw()
for database storage or esc_url()
when echoing a URL in an HTML attribute.
$cleaned_url = esc_url_raw($_POST['url_input']);
Sanitizing Numbers
For integers and floating-point numbers, use intval()
and floatval()
respectively:
$cleaned_integer = intval($_POST['integer_input']);
$cleaned_float = floatval($_POST['float_input']);
Sanitizing HTML Content
If you need to allow some HTML tags (like in a rich text editor), use wp_kses_post()
for post content or wp_kses()
for more specific cases where you define allowed tags and attributes.
$allowed_html = array(
'a' => array(
'href' => array(),
'title' => array()
),
'br' => array(),
'em' => array(),
'strong' => array(),
);
$cleaned_html = wp_kses($_POST['html_input'], $allowed_html);
Escaping Output
While not directly part of sanitization, escaping data before outputting it to the browser is another crucial security practice. Use esc_html()
, esc_attr()
, esc_url()
, and esc_js()
depending on the context where you're displaying the data.
Using Nonce for Form Verification
In addition to sanitizing inputs, use nonces (a "number used once") to verify that the data submitted is intentional and from your site.
wp_nonce_field('my_form_action', 'my_form_nonce');
Verify the nonce when processing the form:
if (!isset($_POST['my_form_nonce']) || !wp_verify_nonce($_POST['my_form_nonce'], 'my_form_action')) {
// Handle the invalid nonce case
}
Sanitizing input in WordPress is not just best practice—it's a critical component of website security and integrity. By using WordPress's built-in functions to clean and verify user input, you significantly reduce the risk of security vulnerabilities and ensure your website remains safe and trustworthy.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
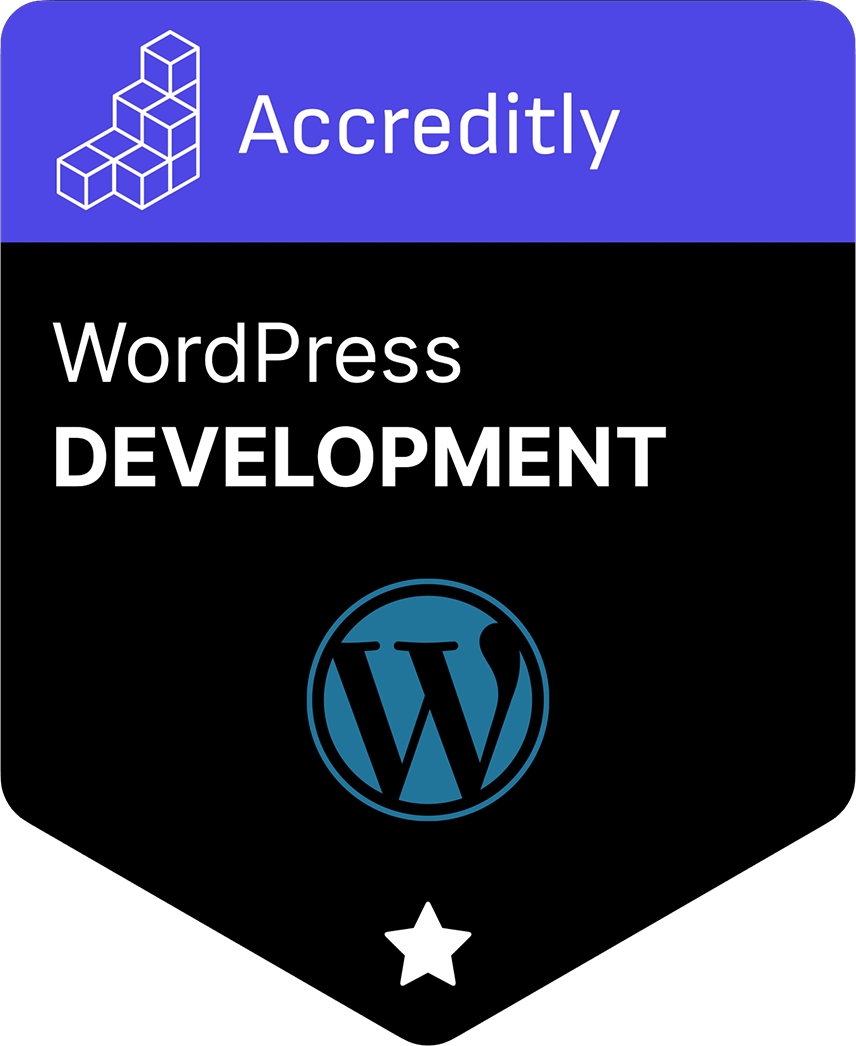