- Understanding the reorder Method in Laravel Eloquent
- Syntax and Usage
- Practical Examples
- Best Practices and Considerations
In the realm of web development, especially when dealing with dynamic content management systems, the ability to reorder data effortlessly is crucial. Laravel, a premier PHP framework, simplifies this task with its Eloquent ORM, providing a seamless approach to manipulating database records. Among its arsenal of features, the reorder
method stands out for its flexibility and ease of use. This article delves into the reorder
method in Laravel Eloquent, showcasing its utility and providing examples to guide you through its implementation.
reorder
Method in Laravel Eloquent
Understanding the Laravel's Eloquent ORM is renowned for its ability to abstract complex SQL queries into simple, fluent PHP methods. The reorder
method is particularly useful when you need to override the default sorting behavior of a query.
By default, Eloquent queries return records in the order they are retrieved from the database, often influenced by the model's $orderBy
attribute or any applied sorting. However, there are scenarios where temporary reordering is required, without altering the global scope or default order criteria. This is where reorder
shines.
Syntax and Usage
The reorder
method resets any existing orderBy
constraints and optionally applies new ordering criteria.
Basic Syntax:
$query->reorder($column, $direction = 'asc');
-
$column
: The column name you wish to order by. -
$direction
: The direction of the sort, either 'asc' for ascending or 'desc' for descending. This parameter is optional and defaults to 'asc'.
Resetting Order:
To simply remove any existing order constraints without applying a new one:
$query->reorder();
Practical Examples
Example 1: Basic Reordering
Imagine you have a Post
model and you typically retrieve posts ordered by their creation date. However, for a specific view, you want to reorder them by title.
$posts = Post::orderBy('created_at')->get(); // Default order
$reorderedPosts = Post::orderBy('created_at')->reorder('title', 'asc')->get(); // Reordered by title
Example 2: Resetting Order
In a scenario where posts are ordered by a sticky flag (where 'sticky' posts appear first), but you need a version without this ordering:
$posts = Post::orderBy('is_sticky', 'desc')->get(); // Default sticky first
$normalPosts = Post::orderBy('is_sticky', 'desc')->reorder()->get(); // Resets order
Example 3: Dynamic Reordering Based on User Input
Consider a blog where the user can choose how the posts are sorted, either by date or by title:
$orderBy = $request->input('order_by', 'created_at'); // Default to 'created_at'
$direction = $request->input('direction', 'asc'); // Default to 'asc'
$posts = Post::reorder($orderBy, $direction)->get();
This example demonstrates how reorder
can dynamically adjust query ordering based on user input, enhancing user experience by providing control over data presentation.
Best Practices and Considerations
- Frequent use of dynamic reordering on large datasets can impact performance. Indexing order-by columns can mitigate this.
- When using user input to determine order criteria, ensure the input is validated to prevent SQL injection or errors from invalid column names.
- Always provide a default ordering to ensure consistent results, especially when the ordering field is dynamically determined.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
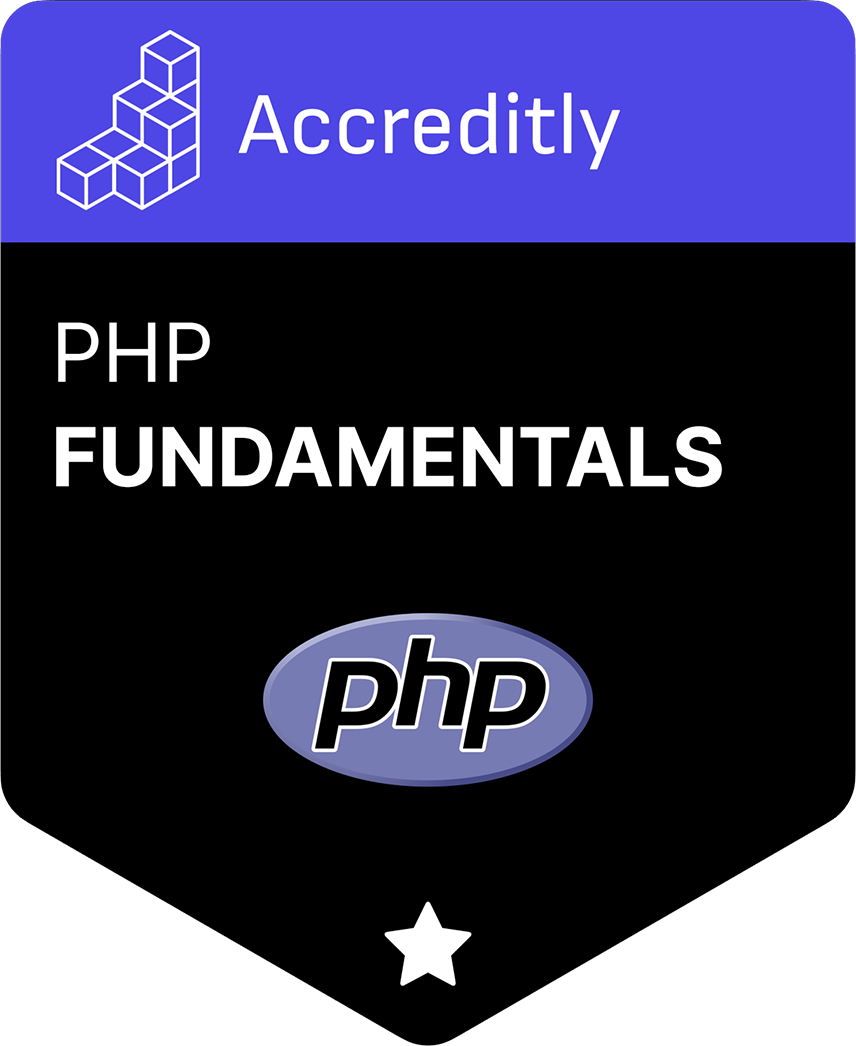