- Understanding Theme Options Pages
- Prerequisites
- Step 1: Create a Theme Options Menu
- Step 2: Building the Options Page
- Step 3: Registering Settings
- Step 4: Adding Fields to Your Options Page
- Step 5: Styling Your Options Page
- Step 6: Using the Options in Your Theme
- Best Practices
WordPress themes often come with a variety of customization options. However, creating a custom theme options page allows for even greater control and personalization. Whether you're a theme developer or a WordPress enthusiast looking to tailor your theme, a custom options page can be a game-changer. Here's how to create one.
Understanding Theme Options Pages
A theme options page is an admin page where users can manage various settings and preferences of a WordPress theme. From layout choices to color schemes, these options allow users to tailor the theme to their needs without diving into code.
Prerequisites
-
Basic understanding of PHP and WordPress development.
-
A WordPress theme you wish to add options to.
Step 1: Create a Theme Options Menu
Start by adding a new menu item for your theme options page. Place this code in your theme's functions.php
file:
function my_theme_menu() {
add_theme_page('Theme Options', 'Theme Options', 'edit_theme_options', 'my-theme-options', 'my_theme_options_page');
}
add_action('admin_menu', 'my_theme_menu');
This code creates a submenu called 'Theme Options' under the 'Appearance' menu in the WordPress admin.
Step 2: Building the Options Page
The my_theme_options_page
function is where you'll structure the HTML output of your options page:
function my_theme_options_page() {
?>
<div class="wrap">
<h1>My Theme Options</h1>
<form method="post" action="options.php">
<?php
settings_fields('my-theme-settings-group');
do_settings_sections('my-theme-settings-group');
submit_button();
?>
</form>
</div>
<?php
}
Step 3: Registering Settings
Before users can save options, you need to register settings using the register_setting()
function:
function my_theme_settings() {
register_setting('my-theme-settings-group', 'some_setting');
}
add_action('admin_init', 'my_theme_settings');
Replace 'some_setting'
with the name of the setting you want to save.
Step 4: Adding Fields to Your Options Page
To add fields to your options page, use the add_settings_field()
function. You can call this function inside my_theme_settings()
:
add_settings_field('my-setting', 'My Setting', 'my_setting_callback', 'my-theme-options', 'my-theme-settings-group');
The my_setting_callback
function will render the HTML for the field:
function my_setting_callback() {
$setting = esc_attr(get_option('my-setting'));
echo "<input type='text' name='my-setting' value='$setting' />";
}
Step 5: Styling Your Options Page
Use CSS to style your options page. You can enqueue a CSS file in your theme specifically for this page, or add inline styles in the PHP file.
Step 6: Using the Options in Your Theme
Retrieve and use the options in your theme with get_option()
:
$my_setting = get_option('my-setting');
echo '<div>' . $my_setting . '</div>';
Best Practices
-
Sanitize and Validate: Always sanitize and validate your theme options to prevent security vulnerabilities.
-
User Experience: Design your options page for clarity and ease of use.
-
Theme Customizer: Consider adding options to the WordPress Customizer (
customize_register
action) for a more integrated experience.
Creating a custom theme options page allows you to provide users with a tailored experience, making your WordPress theme more versatile and user-friendly. By following these steps, you can construct an intuitive options page that elevates your theme to new heights of customization.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
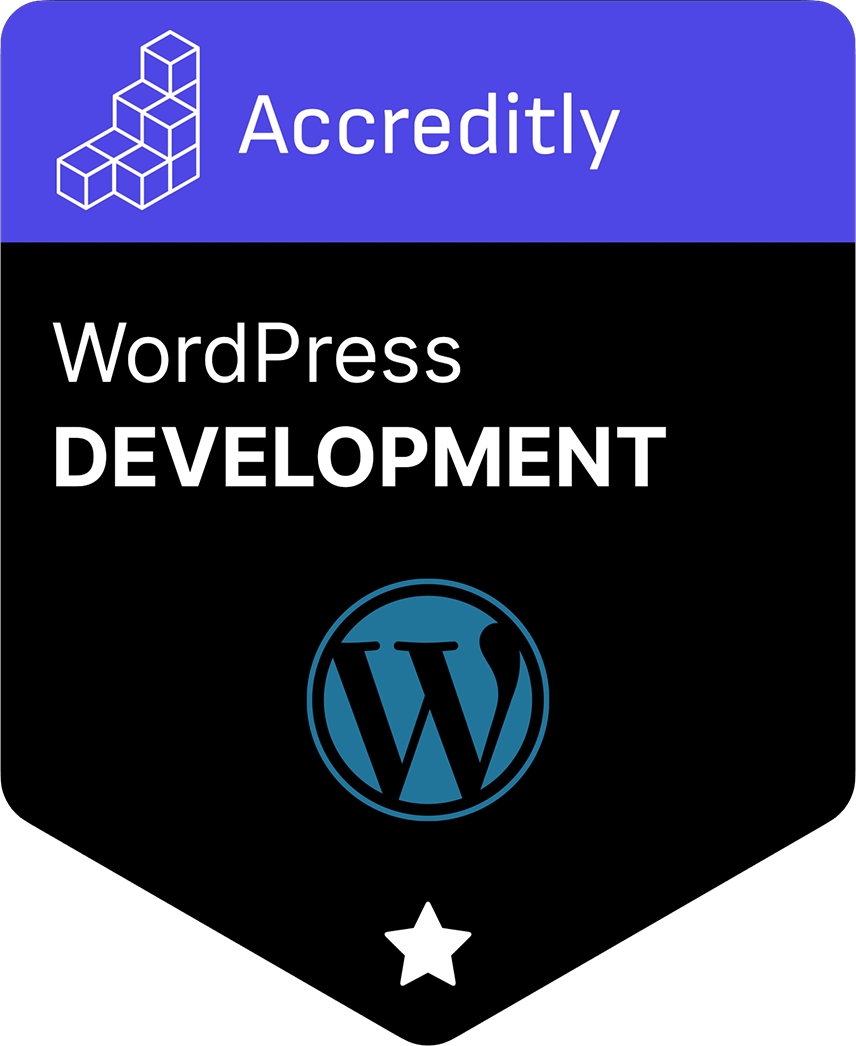