- forceFill
- forceCreate
- When to use forceFill and forceCreate
- Where to find the underlying methods
- Wrapping up
In Laravel, Eloquent is an ORM (Object-Relational Mapping) that makes it simple and enjoyable to interact with databases. Eloquent provides various methods for managing and manipulating data, and two of these methods are forceFill and forceCreate. In this article, we will explore what each method does, when to use them, where to find the underlying methods within Laravel, and provide code examples for each method.
forceFill
The forceFill
method is used to forcefully fill attributes on a model instance without respecting the model's $fillable
property. This method is useful when you need to bypass the model's mass assignment protection and set attributes that are not explicitly defined as fillable.
class User extends Model {
protected $fillable = ['first_name', 'last_name'];
}
$user = new User();
$user->forceFill([
'first_name' => 'John',
'last_name' => 'Doe',
'email' => '[email protected]',
]);
In this example, even though the email attribute is not defined in the $fillable
property, using forceFill
allows us to set its value.
forceCreate
The forceCreate
method is similar to the forceFill
method, but it goes one step further by creating a new model instance and saving it to the database, again without respecting the model's $fillable
property.
class User extends Model {
protected $fillable = ['first_name', 'last_name'];
}
$user = User::forceCreate([
'first_name' => 'John',
'last_name' => 'Doe',
'email' => '[email protected]',
]);
In this example, we use forceCreate
to create a new user record in the database with the provided attributes, including the non-fillable email attribute.
forceFill
and forceCreate
When to use While both forceFill
and forceCreate
can be useful in certain scenarios, it's essential to understand the security implications of bypassing mass assignment protection. Using these methods can potentially expose your application to vulnerabilities if you're not careful.
You should use forceFill
and forceCreate
only when you are sure that the data being passed to the methods is safe and comes from a trusted source. If you're working with user-submitted data, it's recommended to stick with the standard fill
and create
methods and explicitly define fillable attributes using the $fillable
property.
Where to find the underlying methods
Both forceFill
and forceCreate
methods are part of Laravel's Eloquent Model. You can find their implementation in the following files:
-
forceFill
method: TheforceFill
method is located in theIlluminate\Database\Eloquent\Concerns\HasAttributes
trait, which is used by theIlluminate\Database\Eloquent\Model
class. -
forceCreate
method: TheforceCreate
method is a static method on theIlluminate\Database\Eloquent\Model
class itself.
Wrapping up
Understanding the use cases and differences between Laravel's forceFill
and forceCreate
methods can help you make informed decisions when working with Eloquent models in your application. While these methods can be handy in certain situations, it's crucial to remember their security implications and ensure that you're only using them with trusted data sources.
In summary, forceFill
allows you to forcefully fill attributes on a model instance without respecting the model's $fillable
property, while forceCreate
creates a new model instance and saves it to the database, again bypassing the $fillable
property. Always exercise caution when using these methods, and refer to the Laravel documentation for additional information and guidance.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
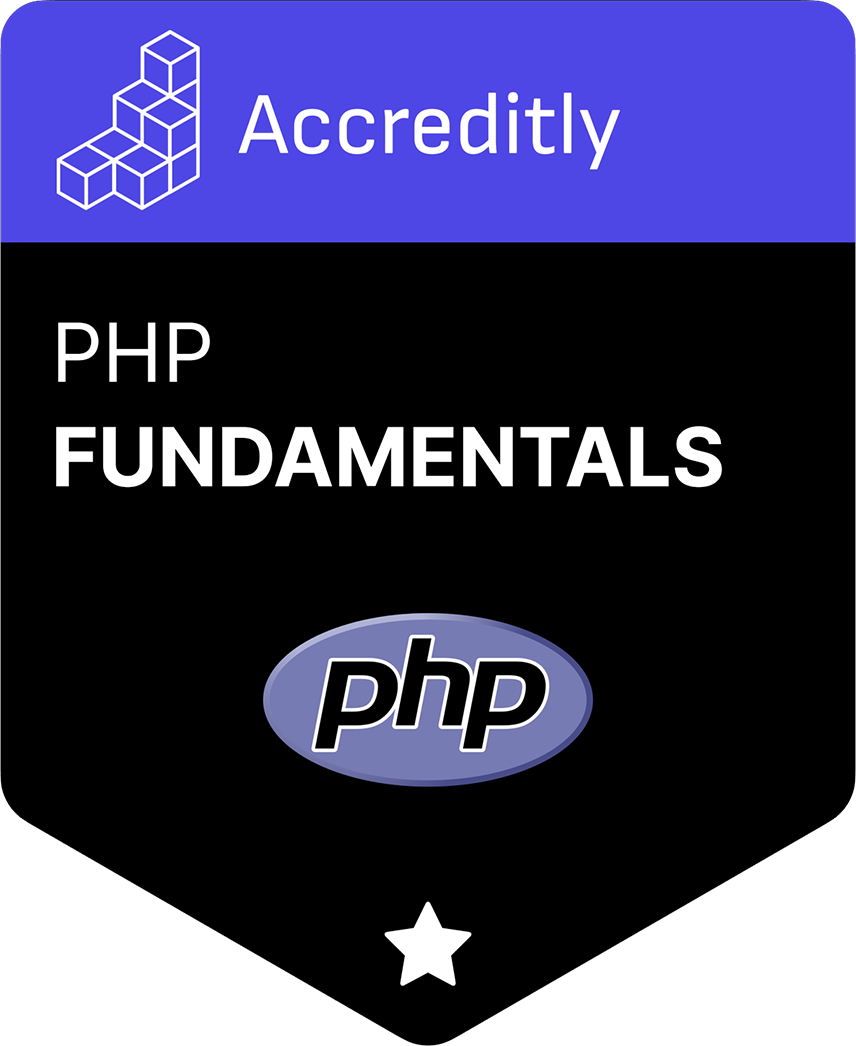