- Understanding Many-to-Many Relationships
- Basic Usage of the sync Method
- Enabling Timestamps
- Using sync with Timestamps
- Best Practices and Considerations
Laravel's Eloquent ORM provides an elegant and efficient way to interact with your database. Among its powerful features, the sync
method stands out for managing many-to-many relationships easily. Typically used with pivot tables, sync
helps in attaching, detaching, and updating relationship data between models without multiple complex queries. This article dives into how to use the sync
method, particularly focusing on handling timestamps, which can be crucial for tracking changes in pivot table entries.
Understanding Many-to-Many Relationships
In a many-to-many relationship, two models are connected by a pivot table. Each entry in this table typically consists of the foreign keys of the connected models. For example, if you have users
and roles
, the pivot table (role_user
) would contain user_id
and role_id
.
sync
Method
Basic Usage of the The sync
method is used to update the pivot table for many-to-many relationships, ensuring that only the specified IDs are present in the table. It automatically adds new entries and removes unmentioned ones, simplifying the process of updating these relationships without manual queries.
Here's a simple example without considering timestamps:
$user->roles()->sync([1, 2, 3]);
This will adjust the role_user
pivot table so that only roles with IDs 1, 2, and 3 are associated with the user, removing any other previously associated roles.
Enabling Timestamps
To track when a pivot table record is created or updated, Eloquent allows you to easily handle timestamps. First, you need to ensure that your pivot table includes created_at
and updated_at
columns. You can add these in your migration like this:
Schema::create('role_user', function (Blueprint $table) {
$table->foreignId('role_id')->constrained();
$table->foreignId('user_id')->constrained();
$table->timestamps(); // Adds created_at and updated_at columns
});
Next, you need to instruct Eloquent to maintain these timestamps when updating pivot table data. This is done by defining the relationship using the withTimestamps
method in your Eloquent model.
public function roles() {
return $this->belongsToMany(Role::class)->withTimestamps();
}
sync
with Timestamps
Using Once you have set up your models and database to handle timestamps, using the sync
method remains straightforward. When you call sync
, Laravel will now also update the updated_at
timestamp each time a pivot table entry is updated, and set created_at
and updated_at
for new entries.
$user->roles()->sync([1, 2]);
This operation ensures that:
- The
updated_at
timestamp is refreshed for the rows whererole_id
is 1 or 2 if they already existed. - New rows are created with both
created_at
andupdated_at
timestamps ifrole_id
1 or 2 were not previously associated with the user.
Best Practices and Considerations
-
Selective Syncing: If you don't want to detach existing relationships that aren't in the provided array, use the
syncWithoutDetaching
method. -
Handling Pivot Data: Apart from timestamps, if your pivot table contains other columns, you can manage them by passing additional details to the
sync
method:$user->roles()->sync([1 => ['expires' => now()->addDays(30)], 2]);
-
Performance: Be mindful of the performance impact when syncing a large number of records, as each
sync
operation could potentially involve multiple database queries.
The sync
method in Laravel Eloquent offers a highly efficient way to manage many-to-many relationships, and the addition of timestamp management further enhances its utility. By properly utilizing timestamps, developers can maintain a detailed audit trail of when relationships are formed or updated, adding robustness and traceability to the application's data handling capabilities. Whether for user roles, tags on posts, or any similar relationships, mastering the sync
method with timestamps will surely elevate your Laravel projects.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
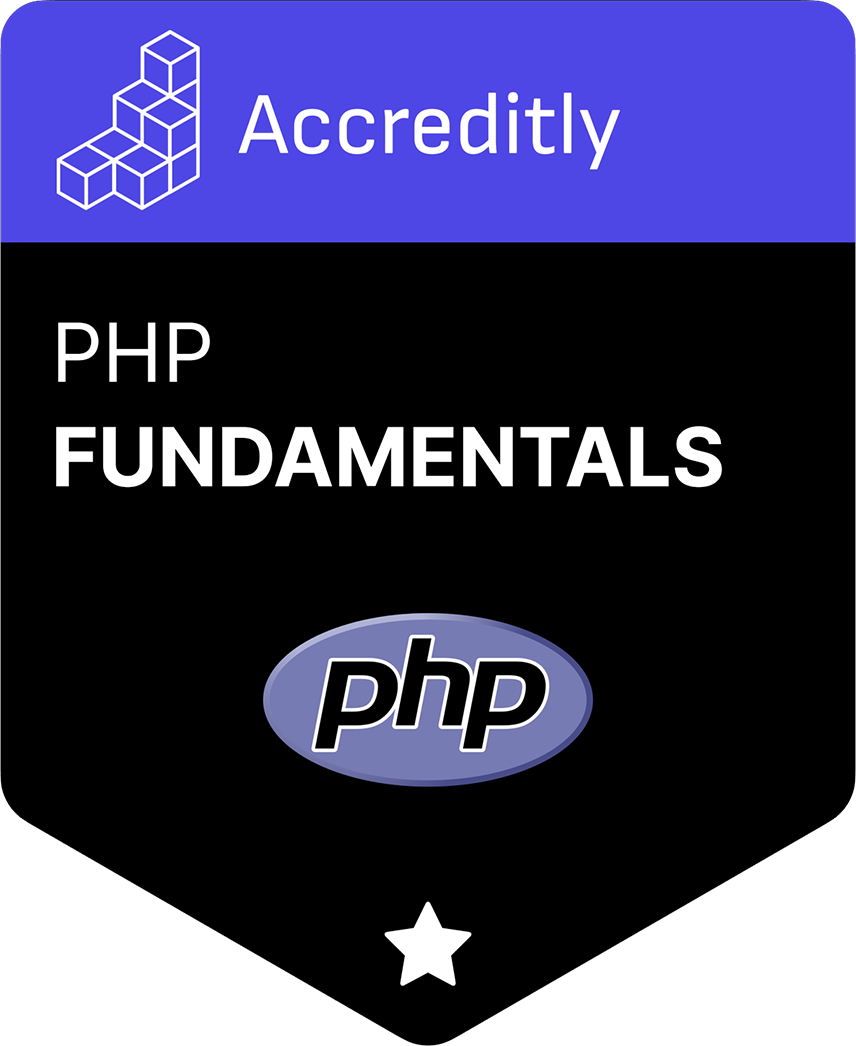