- What is the findMany Method?
- Basic Usage of findMany
- How findMany Works Under the Hood
- Advantages of Using findMany
- findMany with Conditional Clauses
- Handling Results from findMany
- findMany vs. find
Laravel's Eloquent ORM is renowned for its elegant and efficient way of interacting with databases. Among its various features, the findMany
method stands out as a powerful tool for retrieving multiple records based on an array of primary keys. Understanding how to use findMany
effectively can streamline your data retrieval processes in Laravel. Let's explore this method in detail.
Before we get started, you can check out findMany
's API documentation, which may shed some light on how to get started on using findMany
.
What is the findMany Method?
The findMany
method in Laravel's Eloquent ORM allows you to retrieve multiple records from a database table by passing an array of primary key values. It's particularly useful when you need to fetch several specific rows without executing multiple queries.
findMany
is one of Laravel's many lesser-known but valuable methods, of which we have compiled a comprehensive list.
Basic Usage of findMany
The findMany
method is used on an Eloquent model instance and takes an array of IDs as its argument. Here's a basic example:
$users = User::findMany([1, 2, 3]);
In this example, Eloquent fetches users with IDs 1, 2, and 3 from the users
table.
How findMany Works Under the Hood
When you call findMany
, Eloquent constructs a query that uses the whereIn
SQL clause. It translates to a query like SELECT * FROM users WHERE id IN (1, 2, 3)
. This is more efficient than fetching each user individually because it consolidates multiple queries into a single database call.
Advantages of Using findMany
1. Performance: Reduces the number of queries to the database, which is beneficial for performance, especially when dealing with a large number of records.
2. Readability: Makes the code more readable and concise, as you can retrieve multiple models with a single line of code.
3. Flexibility: Works with any primary key, not just auto-incrementing integers.
findMany with Conditional Clauses
You can chain other query builder methods before calling findMany
to add conditions to your query. For example:
$activeUsers = User::where('active', 1)->findMany([1, 2, 3]);
This query fetches active users with IDs 1, 2, and 3.
Handling Results from findMany
The findMany
method returns a Laravel collection, which means you can use all the collection methods, such as each
, map
, and filter
, to manipulate the results:
$users = User::findMany([1, 2, 3]);
$users->each(function ($user) {
echo $user->name;
});
findMany vs. find
While find
is used to retrieve a single model by its primary key, findMany
is ideal for fetching multiple models. If you pass a single ID to findMany
, it still returns a collection, whereas find
will return a single model instance or null.
The findMany
method is a testament to Laravel's commitment to simplifying common tasks in web development. By using findMany
, developers can write cleaner, more efficient code for fetching multiple records, making their Laravel applications more robust and maintainable.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
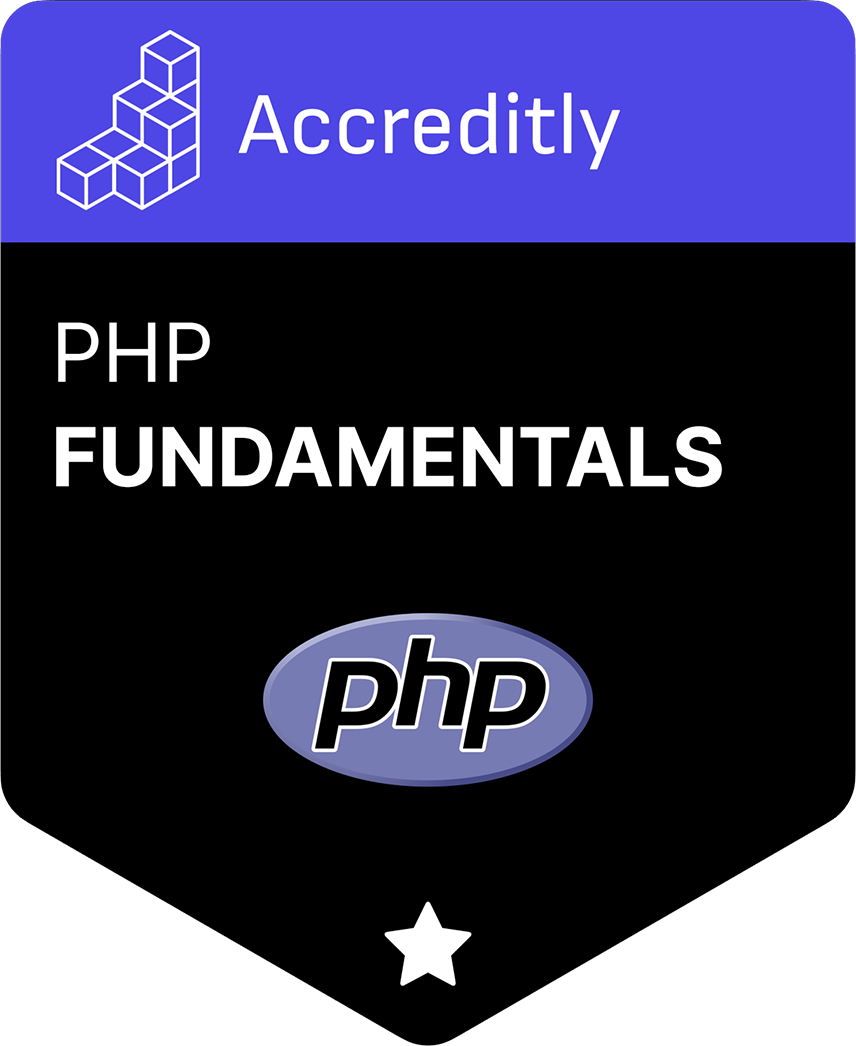