Faker is a well-known PHP library used to generate fake data. Whether you're setting up a set of dummy users for your application or just need some random text for testing, Faker is the go-to for many developers. One of its powerful features is the ability to extend its functionalities by creating custom providers. In this article, we'll guide you through the steps to create your own Faker provider.
1. Setting up Faker
If you haven’t already installed Faker, do so via Composer:
composer require fakerphp/faker
After installation, you can utilize the library as follows:
$faker = \Faker\Factory::create();
echo $faker->name; // Outputs: 'Aurelie McKenzie'
2. Creating a Custom Provider
Let's assume you're developing a gaming application and you need to generate fake names of game characters. There isn't a built-in Faker provider for this, so you decide to create one.
Step 1: Create a new class named GameCharacterProvider
. The class will contain methods that provide the fake data.
namespace CustomFaker;
class GameCharacterProvider
{
protected static $characterNames = [
'Aragon', 'Luigi', 'Zelda', 'Sonic', 'Lara Croft', 'Mario'
];
public function gameCharacter()
{
return static::$characterNames[array_rand(static::$characterNames)];
}
}
Step 2: Now, let’s add the provider to the Faker generator.
$faker->addProvider(new \CustomFaker\GameCharacterProvider($faker));
echo $faker->gameCharacter(); // Outputs: 'Sonic'
3. Extending a Provider
Faker's built-in providers can be extended to give more domain-specific data. For example, if you wish to expand the name provider to include game-related prefixes:
namespace CustomFaker;
class ExtendedNameProvider extends \Faker\Provider\en_US\Person
{
protected static $prefix = ['Lord', 'Lady', 'Sir', 'Master', 'Knight', 'Dame'];
public static function gamePrefix()
{
return static::randomElement(static::$prefix);
}
}
Usage:
$faker->addProvider(new \CustomFaker\ExtendedNameProvider($faker));
echo $faker->gamePrefix(); // Outputs: 'Knight'
4. Sharing Your Provider
If you believe others might benefit from your custom provider, consider sharing it!
- Fork the Faker repository on GitHub.
- Add your custom provider.
- Create a pull request with a brief description of your provider.
While the maintainers of Faker are selective about what makes it into the main branch, having your work out there on your own fork or in the form of a pull request can be helpful to others.
Faker’s flexibility and extensibility make it an excellent tool for all sorts of development needs. By understanding how to create custom providers, you can generate more domain-specific data tailored to your application's requirements.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
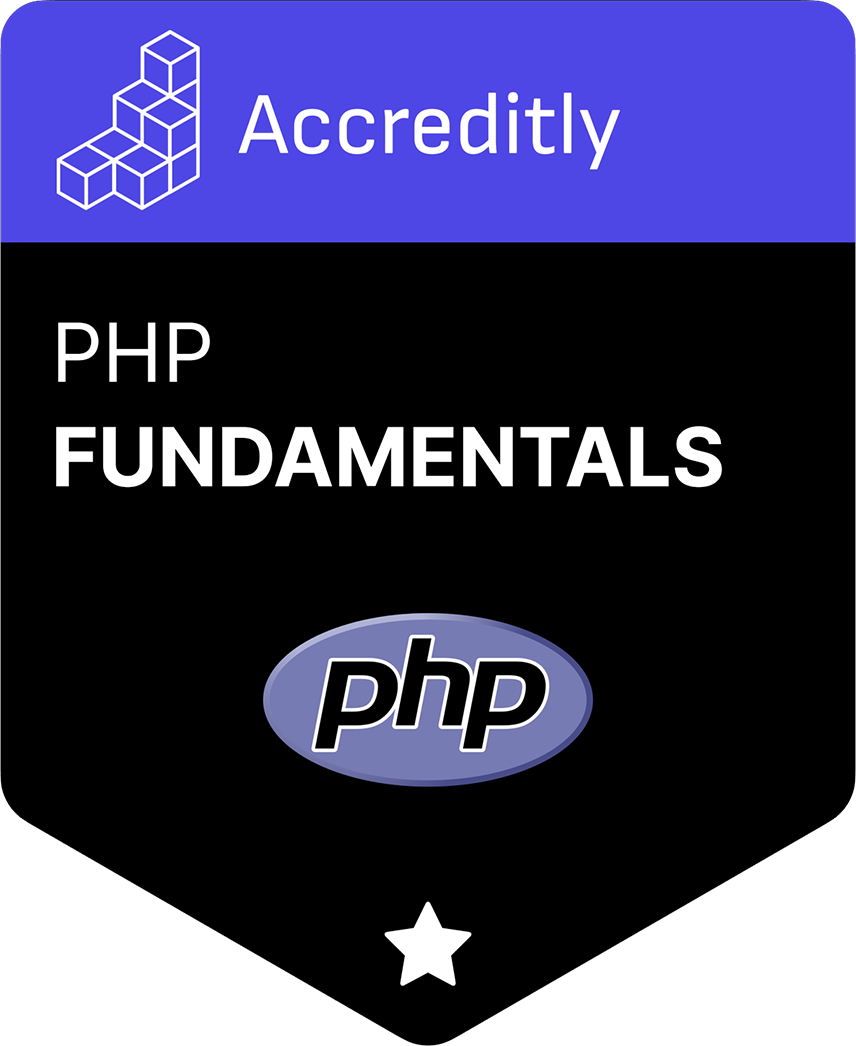
Related articles
Tutorials WordPress PHP Tooling
How to Build an Image Carousel in Gutenberg
Create an image carousel directly within the Gutenberg editor in WordPress. This step-by-step guide provides all the necessary insights and tips to enhance your posts and pages with visually captivating carousels.