- Understanding the Integration
- Step 1: Setting Up Vue.js
- Step 2: Including Vue in Your Theme
- Step 3: Creating Vue Components
- Step 4: Integrating Vue with WordPress Data
- Step 5: Mounting Vue Instances
- Best Practices and Considerations
Vue.js, with its simplicity and flexibility, has become a popular choice for front-end development. Combining Vue with WordPress can bring interactivity and reactivity to your WordPress themes, making them more dynamic and engaging. However, this integration requires a thoughtful approach to blend WordPress's PHP-driven architecture with Vue's JavaScript framework. Let's explore how to effectively use Vue in your WordPress theme.
Understanding the Integration
The key to integrating Vue.js into a WordPress theme is to recognize that Vue will primarily handle the front-end interactive parts of your website, while WordPress manages the backend and content management.
Step 1: Setting Up Vue.js
First, you need to set up Vue.js. You can do this in two ways:
1. Using Vue Directly: Include Vue.js via a CDN in your theme's header.php
or footer.php
file:
```html
```
2. Using NPM and Webpack: For a more robust development process, you can set up Vue using Node Package Manager (NPM) and bundle your scripts using Webpack. This approach is more complex but offers greater flexibility and control.
We always recommend this method if you're able to.
Step 2: Including Vue in Your Theme
After setting up Vue, you need to include it in your theme. Place your Vue components and initialization scripts in a separate JavaScript file and enqueue it in your theme's functions.php
file:
function mytheme_enqueue_scripts() {
wp_enqueue_script('my-vue-script', get_template_directory_uri() . '/js/vue-app.js', array(), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'mytheme_enqueue_scripts');
Step 3: Creating Vue Components
Create your Vue components in separate .vue
files or as part of your main Vue script. These components can be as simple or complex as needed, depending on what part of your theme you are making interactive.
Step 4: Integrating Vue with WordPress Data
To integrate Vue with WordPress data, you can:
-
Use WordPress's REST API to fetch and display content in your Vue components.
-
Pass data from WordPress to Vue components using
wp_localize_script()
.
Here's an example of using wp_localize_script()
to pass data:
wp_localize_script('my-vue-script', 'wpData', array(
'site_url' => get_site_url(),
// Other data you want to pass
));
In your Vue script, you can access this data as wpData.site_url
.
Step 5: Mounting Vue Instances
Choose where in your theme you want to mount Vue instances. This could be a specific element in your templates where you need interactivity.
<div id="vue-app">
<!-- Vue components go here -->
</div>
Initialize Vue in your JavaScript file:
new Vue({
el: '#vue-app',
// Vue options go here
});
Best Practices and Considerations
-
Do Not Overuse: Integrate Vue into parts of your theme that truly benefit from its reactivity and interactivity.
-
SEO Considerations: Remember that Vue renders content client-side, which can have implications for SEO. Consider server-side rendering for critical content.
-
Performance: Be mindful of the performance impact. Only load Vue on pages that need it, and optimize your Vue assets.
Integrating Vue.js into a WordPress theme allows you to enhance specific parts of your website with sophisticated interactivity that goes beyond what traditional PHP and WordPress can offer. This approach is especially beneficial for interactive user interfaces, such as complex forms, real-time data displays, and dynamic content areas.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
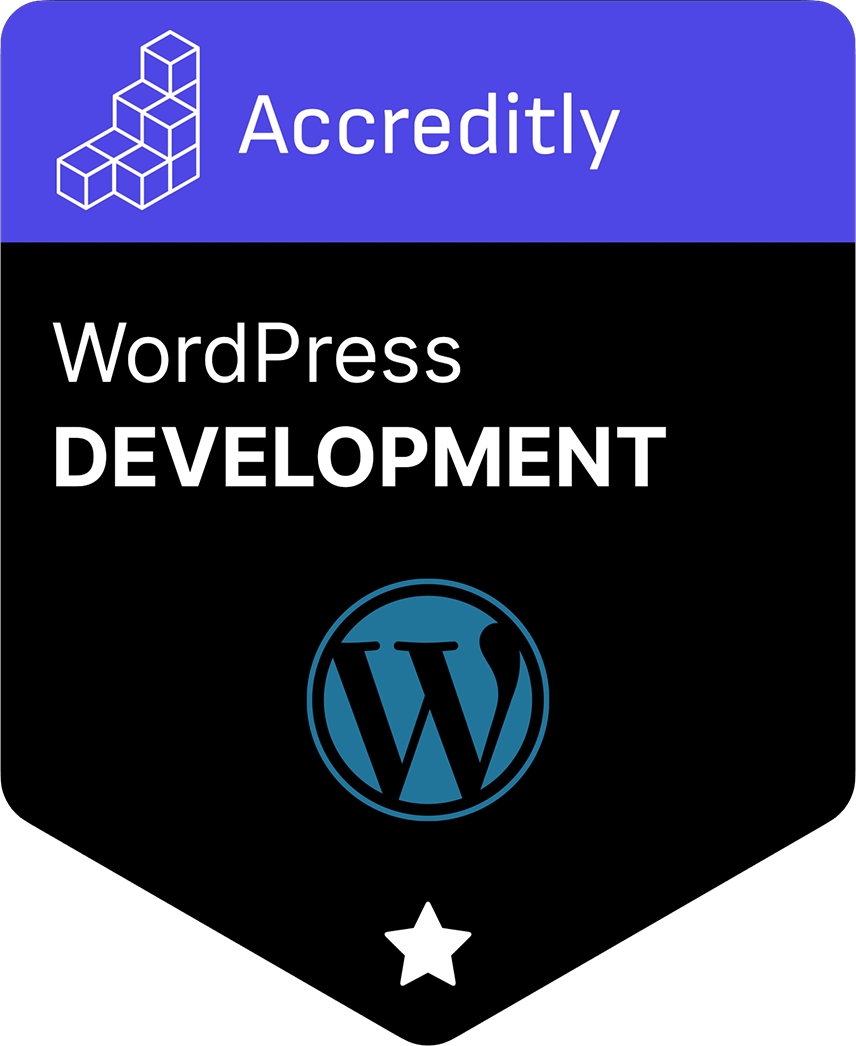