- Understanding DNS-Based Validation
- The Power of Laravel's Validation Rules
- Adding DNS Rule to Your Validation
- Crafting User-Friendly Error Messages
- Handling Edge Cases
- Testing DNS Validation
When users sign up on your Laravel-based application, you want to make sure the email addresses they provide are not only formatted correctly but also hail from a legitimate domain. The Laravel framework makes it a breeze to incorporate comprehensive validation rules right out of the box, including checking DNS records for the email's domain. Let's upgrade your validation game with DNS lookups!
Understanding DNS-Based Validation
DNS (Domain Name System) validation goes beyond checking if an email address looks right ([email protected]). It ensures that the domain (example.com) has MX (Mail Exchange) records, which means it's configured to send and receive emails. This is a critical step to avoid fake or disposable email addresses that can clutter your database and skew your user metrics.
The Power of Laravel's Validation Rules
Laravel provides a fluent way to define validation rules within your form requests. For emails, you typically use the 'email'
rule to validate the format according to the RFC standard. By appending :dns
to it, you instruct Laravel to perform a DNS lookup as part of the validation process.
Adding DNS Rule to Your Validation
When defining your form request validation rules, it's as simple as this:
$request->validate([
'email' => 'required|email:rfc,dns',
]);
This tells Laravel: "Hey, make sure this email adheres to the RFC standard, and oh, check the DNS records while you're at it!"
Crafting User-Friendly Error Messages
In case the DNS lookup fails, you want to provide a clear and helpful error message to your users. Customize your validation messages like so:
$messages = [
'email.dns' => 'The email domain provided cannot be verified. Please use a different email address.',
];
$request->validate([
'email' => 'required|email:rfc,dns',
], $messages);
Handling Edge Cases
DNS lookups are generally reliable, but sometimes, temporary network issues can cause legitimate domains to fail the check. Make sure you handle these exceptions gracefully, perhaps by providing the user with the option to retry or contact support.
Testing DNS Validation
Automated tests are your friends. Ensure your new DNS validation rule works under various scenarios. Write tests for valid and invalid email addresses, and if possible, simulate DNS lookup failures to ensure your application responds correctly.
public function testFormRejectsInvalidEmailDomain() {
// Your test implementation here
}
With just a simple tweak to your validation rules, you can greatly enhance the integrity of the email addresses collected through your Laravel application's forms. It's a quick win for both you and your users, adding robustness and trust to your system's onboarding process.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
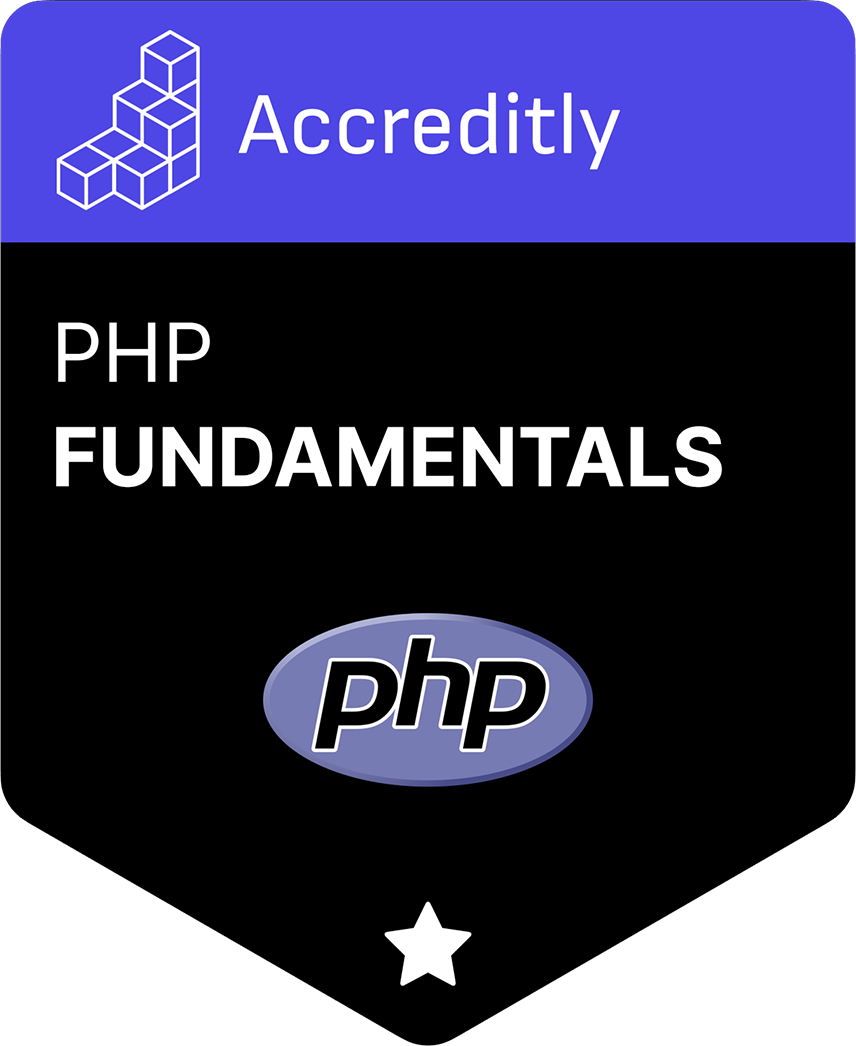