Web development in PHP often involves handling multiple routes or URLs. Whether you're building a content management system or a web application, understanding how to route URLs to specific controllers is essential.
In this article, we'll walk you through building a basic router in PHP, a valuable exercise for understanding the principles behind popular PHP frameworks like Laravel, Symfony, or Slim, which all have powerful routing capabilities.
What is a Router?
In web development, a router is a component responsible for taking a URL and dispatching it to a specific function or method in your code. In other words, the router helps the application determine what code to run based on the URL requested. You'll often find that routers need to handle dynamic values which help identify data. An example of this may be the title of this blog post in the URL, which helps our application identify the post in our database and return the correct content.
Creating a Basic Router in PHP
Let's now look at how to create a simple router using PHP.
Step 1: Enable URL Rewriting
To implement routing in PHP, we first need to ensure that our server redirects all requests to a single PHP file. In an Apache server, we do this with an .htaccess
file in the project root:
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^ index.php [QSA,L]
An example in nginx:
location / {
try_files $uri $uri/ /index.php?$query_string;
}
Effectively this code is telling the web server to run all requests to index.php.
Step 2: Create the Router Class
Next, we'll create a simple router class. The router will store all our routes and dispatch them to the right function when requested. Here's a basic implementation:
class Router
{
private $routes = [];
public function addRoute($route, $function)
{
$this->routes[$route] = $function;
}
public function dispatch()
{
$url = $_SERVER['REQUEST_URI'] ?? '/';
if(isset($this->routes[$url])) {
call_user_func($this->routes[$url]);
} else {
http_response_code(404);
echo "Page not found";
}
}
}
This Router
class provides methods to add routes and dispatch the requested URL to the appropriate function. If no matching route is found, it returns a 404 error.
Step 3: Add Routes
With our router set up, we can add routes. Each route corresponds to a function that will be executed when that route is accessed:
$router = new Router();
$router->addRoute('/', function () {
echo "Welcome to the homepage!";
});
$router->addRoute('/about', function () {
echo "Welcome to the about page!";
});
In this code, we're defining two routes, /
and /about
, each associated with an anonymous function.
Step 4: Dispatch Requests
Finally, we'll tell the router to dispatch requests:
$router->dispatch();
This will call the function associated with the current URL.
This router can be expanded with more features, like handling different request methods (GET, POST), adding variables to routes, or even handling regex patterns.
Building your own router is a great way to understand the inner workings of popular PHP frameworks. However, for larger projects, consider using a full-featured framework like Laravel, Symfony, or Slim, as they provide robust, secure routing capabilities along with many other features to facilitate web development.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
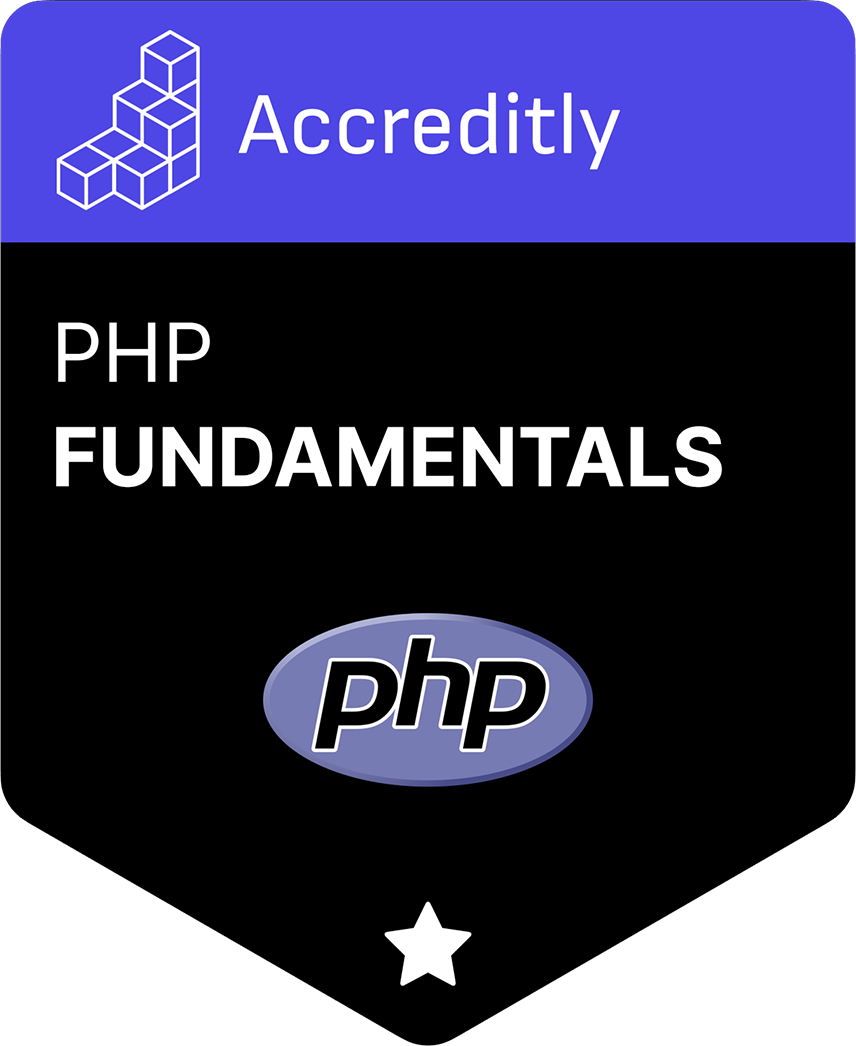