- Introduction to Tailwind CSS and Blade Components
- Setting Up Tailwind CSS in a Laravel Project
- Creating Blade Components
- Using Tailwind CSS with Blade Components
- Advanced Tailwind CSS Techniques with Blade Components
- Testing and Debugging
- Performance Optimization
- Wrapping Up: Mastering Tailwind CSS and Blade Components
Tailwind CSS and Blade components are a powerful combination for building modern, responsive web applications in Laravel. Tailwind CSS provides utility-first styling, while Blade components offer reusable and customizable HTML elements. Together, they streamline the development process, improve code maintainability, and enhance the user experience. This comprehensive guide will walk you through integrating Tailwind CSS with Blade components, covering installation, configuration, and practical examples.
Introduction to Tailwind CSS and Blade Components
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework for rapidly building custom designs. Unlike traditional CSS frameworks like Bootstrap, which provide predefined components and styles, Tailwind CSS offers low-level utility classes that can be composed to create any design directly in your markup. This approach leads to more maintainable and scalable codebases, as it encourages the reuse of small, atomic classes.
What are Blade Components?
Blade components are reusable pieces of UI code in Laravel's Blade templating engine. They encapsulate HTML, JavaScript, and PHP logic, promoting code reusability and separation of concerns. Blade components can accept data and parameters, making them highly customizable and versatile for different parts of your application.
Setting Up Tailwind CSS in a Laravel Project
To use Tailwind CSS with Blade components, you first need to set up Tailwind in your Laravel project. Follow these steps to get started:
-
Install Laravel:
If you haven't already, create a new Laravel project using Composer:
composer create-project --prefer-dist laravel/laravel tailwind-blade-example
-
Install Tailwind CSS:
Navigate to your project directory and install Tailwind CSS using npm:
cd tailwind-blade-example npm install tailwindcss
-
Initialize Tailwind CSS:
Create the
tailwind.config.js
file by running:npx tailwindcss init
This command generates a configuration file where you can customize Tailwind's default settings.
-
Configure Tailwind CSS:
Open the
tailwind.config.js
file and configure thepurge
option to remove unused styles in production:module.exports = { purge: ['./resources/views/**/*.blade.php', './resources/js/**/*.vue'], darkMode: false, // or 'media' or 'class' theme: { extend: {}, }, variants: { extend: {}, }, plugins: [], }
-
Create Tailwind CSS File:
Create a new CSS file for Tailwind in
resources/css
(e.g.,app.css
) and include the following directives:@tailwind base; @tailwind components; @tailwind utilities;
-
Compile Tailwind CSS:
Update the
webpack.mix.js
file to compile your Tailwind CSS:const mix = require('laravel-mix'); const tailwindcss = require('tailwindcss'); mix.js('resources/js/app.js', 'public/js') .postCss('resources/css/app.css', 'public/css', [ tailwindcss, ]);
Then, run the following command to compile your assets:
npm run dev
Your Laravel project is now set up with Tailwind CSS. Next, we'll create Blade components to utilize these styles effectively.
Creating Blade Components
Blade components help you organize and reuse your UI elements. Let's start by creating a basic Blade component.
-
Generate a Blade Component:
Use the Artisan command to create a Blade component:
php artisan make:component Alert
This command creates two files:
-
app/View/Components/Alert.php
: The component class. -
resources/views/components/alert.blade.php
: The component view.
-
-
Define the Component Class:
Open
app/View/Components/Alert.php
and define the component's properties and logic:<?php namespace App\View\Components; use Illuminate\View\Component; class Alert extends Component { public $type; public $message; public function __construct($type = 'info', $message = 'Default message') { $this->type = $type; $this->message = $message; } public function render() { return view('components.alert'); } }
-
Create the Component View:
Open
resources/views/components/alert.blade.php
and define the HTML structure of the component:<div class="alert alert-{{ $type }}"> {{ $message }} </div>
-
Use the Component in a Blade Template:
Open a Blade template (e.g.,
resources/views/welcome.blade.php
) and include thealert
component:@extends('layouts.app') @section('content') <x-alert type="success" message="This is a success alert!" /> <x-alert type="error" message="This is an error alert!" /> @endsection
This simple component setup allows you to reuse the alert
component throughout your application, passing different types and messages as needed.
Using Tailwind CSS with Blade Components
Now that we have our Blade component set up, let's apply Tailwind CSS styles to it. We'll start by replacing the Bootstrap classes with Tailwind utility classes.
-
Update the Component View with Tailwind CSS:
Open
resources/views/components/alert.blade.php
and update it to use Tailwind CSS:@props(['type', 'message']) @php $typeClasses = [ 'success' => 'bg-green-500 text-white', 'error' => 'bg-red-500 text-white', 'info' => 'bg-blue-500 text-white', ]; @endphp <div {{ $attributes->merge(['class' => 'p-4 rounded ' . $typeClasses[$type]]) }}> {{ $message }} </div>
Here, we define a
$typeClasses
array to map thetype
attribute to corresponding Tailwind classes. We then merge these classes with any additional attributes passed to the component. -
Use the Tailwind-Styled Component:
In your Blade template, use the component with different types:
@extends('layouts.app') @section('content') <x-alert type="success" message="This is a success alert!" class="mb-4" /> <x-alert type="error" message="This is an error alert!" class="mb-4" /> <x-alert type="info" message="This is an info alert!" class="mb-4" /> @endsection
The
class
attribute is merged with the component's default classes, allowing you to add custom styles easily.
Advanced Tailwind CSS Techniques with Blade Components
To leverage the full potential of Tailwind CSS and Blade components, you can use advanced techniques like conditional classes, dynamic attributes, and slot customization.
Conditional Classes
Tailwind CSS's utility classes can be conditionally applied based on component attributes.
-
Create a Card Component:
Generate a new Blade component:
php artisan make:component Card
Define the component class in
app/View/Components/Card.php
:<?php namespace App\View\Components; use Illuminate\View\Component; class Card extends Component { public $shadow; public function __construct($shadow = true) { $this->shadow = $shadow; } public function render() { return view('components.card'); } }
-
Define the Component View with Conditional Classes:
Open
resources/views/components/card.blade.php
:@props(['shadow' => true]) <div {{ $attributes->merge(['class' => 'p-6 bg-white rounded ' . ($shadow ? 'shadow-lg' : '')]) }}> {{ $slot }} </div>
-
Use the Card Component with Conditional Classes:
In your Blade template:
@extends('layouts.app') @section('content') <x-card shadow="false"> <h2 class="text-xl font-bold">No Shadow Card</h2> <p>This card does not have a shadow.</p> </x-card> <x-card> <h2 class="text-xl font-bold">Shadow Card</h2> <p>This card has a shadow.</p> </x-card> @endsection
The
shadow
attribute controls
whether the shadow-lg
class is applied.
Dynamic Attributes
Blade components can accept dynamic attributes, allowing for greater customization and flexibility.
-
Update the Alert Component to Use Dynamic Attributes:
Open
resources/views/components/alert.blade.php
:@props(['type', 'message']) @php $typeClasses = [ 'success' => 'bg-green-500 text-white', 'error' => 'bg-red-500 text-white', 'info' => 'bg-blue-500 text-white', ]; @endphp <div {{ $attributes->merge(['class' => 'p-4 rounded ' . $typeClasses[$type]]) }}> {{ $message }} </div>
-
Use Dynamic Attributes in the Blade Template:
In your Blade template:
@extends('layouts.app') @section('content') <x-alert type="success" message="This is a success alert!" class="mb-4 border-2 border-green-700" /> <x-alert type="error" message="This is an error alert!" class="mb-4 border-2 border-red-700" /> <x-alert type="info" message="This is an info alert!" class="mb-4 border-2 border-blue-700" /> @endsection
The
class
attribute merges with the component's default classes, allowing you to add custom styles.
Slot Customization
Slots enable you to pass additional content into Blade components, making them even more flexible.
-
Create a Card Component with Slots:
Open
resources/views/components/card.blade.php
:<div {{ $attributes->merge(['class' => 'p-6 bg-white rounded shadow-lg']) }}> <div class="mb-4"> {{ $header }} </div> <div> {{ $slot }} </div> <div class="mt-4"> {{ $footer }} </div> </div>
-
Use Named Slots in the Blade Template:
In your Blade template:
@extends('layouts.app') @section('content') <x-card> <x-slot name="header"> <h2 class="text-xl font-bold">Card Header</h2> </x-slot> <p>This is the main content of the card.</p> <x-slot name="footer"> <p class="text-sm text-gray-600">Card Footer</p> </x-slot> </x-card> @endsection
Named slots (
header
andfooter
) allow you to pass additional content into specific sections of the component.
Testing and Debugging
Thorough testing and debugging are essential to ensure your Tailwind and Blade component integration works as expected.
Testing Blade Components
Laravel provides a robust testing framework to validate your Blade components.
-
Create a Blade Component Test:
Generate a test case using Artisan:
php artisan make:test AlertComponentTest
Open
tests/Feature/AlertComponentTest.php
and define your test:<?php namespace Tests\Feature; use Illuminate\Foundation\Testing\RefreshDatabase; use Tests\TestCase; class AlertComponentTest extends TestCase { public function testAlertComponentRendersCorrectly() { $view = $this->blade('<x-alert type="success" message="Test message" />'); $view->assertSee('bg-green-500'); $view->assertSee('Test message'); } }
-
Run Your Tests:
Execute your tests using PHPUnit:
phpunit
Ensure all tests pass and your components render correctly.
Debugging Tailwind CSS
Debugging Tailwind CSS involves inspecting the generated classes and ensuring they are applied as expected.
-
Use Browser DevTools:
Inspect elements in your browser's developer tools to verify Tailwind classes are correctly applied. Look for conflicts or overrides that might affect styling.
-
Enable JIT Mode:
Tailwind CSS's Just-in-Time (JIT) mode improves development speed by generating styles on demand. Enable JIT mode in
tailwind.config.js
:module.exports = { mode: 'jit', purge: ['./resources/views/**/*.blade.php', './resources/js/**/*.vue'], darkMode: false, theme: { extend: {}, }, variants: { extend: {}, }, plugins: [], }
-
Use Tailwind's Debug Screens Plugin:
Install and configure the
@tailwindcss/debug-screens
plugin to display the current screen size and breakpoint in your application:npm install @tailwindcss/debug-screens
Add the plugin to
tailwind.config.js
:module.exports = { // other configurations... plugins: [require('@tailwindcss/debug-screens')], }
This plugin helps you debug responsive design issues by showing the active breakpoint.
Performance Optimization
Optimizing performance is crucial for a smooth user experience. Tailwind CSS and Blade components offer several techniques for improving performance.
Purge Unused CSS
Tailwind CSS's purge option removes unused styles from your final build, reducing file size.
-
Configure Purge in
tailwind.config.js
:module.exports = { purge: ['./resources/views/**/*.blade.php', './resources/js/**/*.vue'], darkMode: false, theme: { extend: {}, }, variants: { extend: {}, }, plugins: [], }
-
Run Production Build:
Build your assets for production:
npm run production
This command purges unused styles and minifies your CSS.
Cache Blade Templates
Blade templates can be cached to improve performance. Laravel's built-in caching mechanism helps reduce rendering time.
-
Enable Template Caching:
Ensure caching is enabled in your
.env
file:CACHE_DRIVER=file
-
Clear and Rebuild Cache:
Clear and rebuild the cache using Artisan commands:
php artisan cache:clear php artisan view:cache
These commands ensure your templates are cached and optimized for performance.
Use Lazy Loading
Lazy loading defers loading images and other resources until they are needed, improving initial page load times.
-
Implement Lazy Loading:
Use the
loading="lazy"
attribute on images:<img src="image.jpg" alt="Example Image" loading="lazy" />
-
Use Intersection Observer API:
For more advanced lazy loading, use the Intersection Observer API to load elements as they enter the viewport.
document.addEventListener('DOMContentLoaded', function () { const lazyImages = document.querySelectorAll('img.lazy'); const observer = new IntersectionObserver((entries) => { entries.forEach((entry) => { if (entry.isIntersecting) { const img = entry.target; img.src = img.dataset.src; img.classList.remove('lazy'); observer.unobserve(img); } }); }); lazyImages.forEach((img) => { observer.observe(img); }); });
Wrapping Up: Mastering Tailwind CSS and Blade Components
Integrating Tailwind CSS with Blade components in Laravel provides a powerful and flexible approach to building modern web applications. By leveraging utility-first styling and reusable components, you can create responsive, maintainable, and scalable UIs.
Key Takeaways:
- Tailwind CSS offers utility-first styling for rapid UI development.
- Blade components promote code reusability and separation of concerns.
- Advanced techniques like conditional classes, dynamic attributes, and slot customization enhance component flexibility.
- Testing and debugging ensure your integration works as expected.
- Performance optimization improves user experience and application efficiency.
For further details and best practices, refer to the official Tailwind CSS documentation and the Laravel Blade component documentation.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
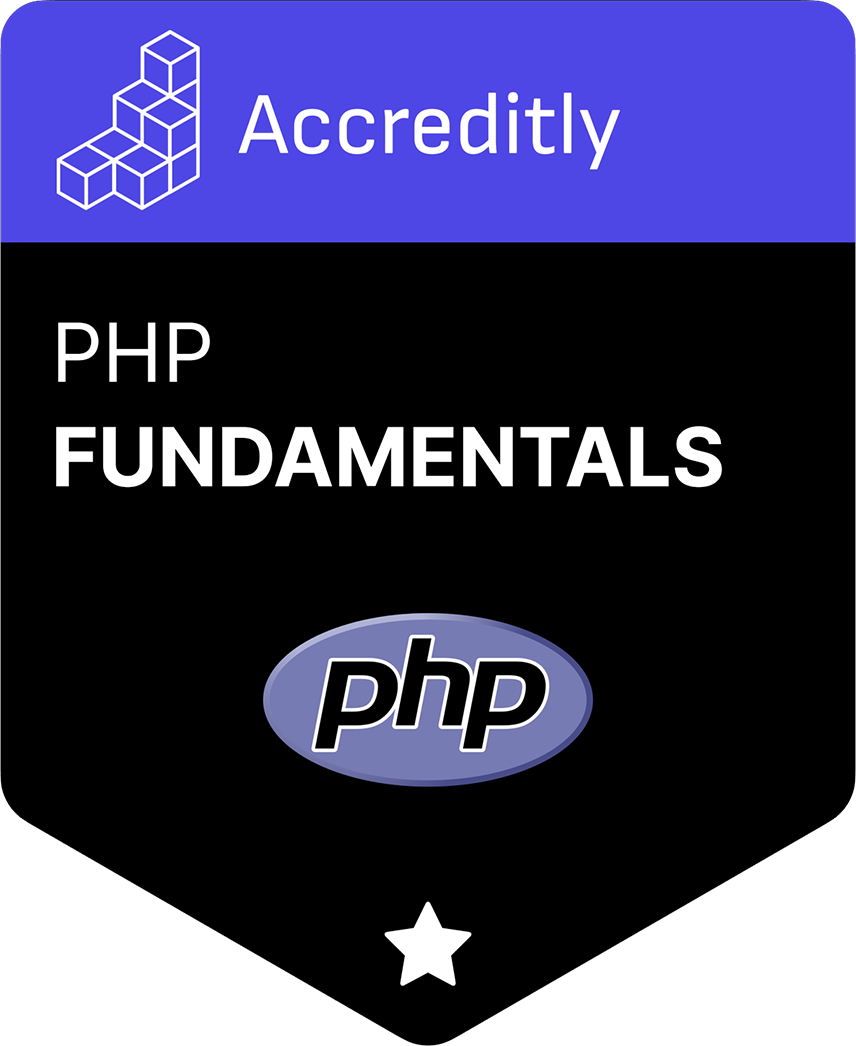