- 1. Understanding the Request Object
- 2. Accessing the Request Object
- 3. Key Methods and Accessors
- 4. Special Input Types
- 5. Modifying the Request
At the core of every web application lies the process of handling user requests and returning appropriate responses. In Laravel, the Request object plays a pivotal role in this cycle. This article sheds light on the indispensable Laravel Request object, elucidating its methods and accessors to simplify data handling.
1. Understanding the Request Object
Every HTTP request made to a Laravel application is represented by an instance of the Illuminate\Http\Request
class (API dev docs). This object provides a plethora of methods to access client input, cookies, files, and more.
2. Accessing the Request Object
You can type-hint the Request
object on a route closure or controller method, and Laravel's service container will automatically inject it:
use Illuminate\Http\Request;
Route::get('/user', function (Request $request) {
//
});
3. Key Methods and Accessors
3.1. Retrieving Input Data
-
$request->input('name')
: Get the value ofname
from the request. You can also specify a default if the key doesn't exist:$request->input('name', 'default')
. -
$request->all()
: Get all input data as an associative array. -
$request->only(['username', 'password'])
: Get specific data points from the request. -
$request->except('credit_card')
: Get all input data excluding certain fields.
3.2. Query Parameters
-
$request->query('search')
: Retrieve thesearch
query parameter.
3.3. Path and URL Information
-
$request->path()
: Get the request's path (excluding query string). -
$request->url()
: Get the full URL, without the query string. -
$request->fullUrl()
: Get the full URL, including the query string. -
$request->is('admin/*')
: Determine if the request path matches a given pattern. Useful for conditional logic based on the route.
3.4. HTTP Verbs & Content
-
$request->method()
: Get the HTTP verb for the request (GET, POST, etc.). -
$request->isMethod('post')
: Check if the request uses a specific method. -
$request->ajax()
: Determine if the request is an AJAX request. -
$request->wantsJson()
: Determine if the request expects a JSON response.
3.5. File Handling
-
$request->hasFile('photo')
: Check if a file is present in the request. -
$request->file('photo')
: Access uploaded file instances. -
$request->file('photo')->isValid()
: Check if the uploaded file is valid. -
$request->file('photo')->store('images')
: Store the uploaded file on the server.
3.6. Session Access
-
$request->session()->get('key')
: Directly access session data via the Request object.
4. Special Input Types
-
$request->cookie('name')
: Retrieve a cookie value. -
$request->header('User-Agent')
: Get the value of an HTTP header. -
$request->ip()
: Get the client's IP address. -
$request->user()
: If using Laravel's built-in authentication, retrieve the authenticated user.
5. Modifying the Request
Although not a common practice, you can modify request data:
$request->merge(['name' => 'Modified Name']);
The Request object in Laravel acts as a comprehensive interface to incoming HTTP requests. Understanding and leveraging its numerous methods and accessors ensures efficient and streamlined data handling and will allow you to build more efficient applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
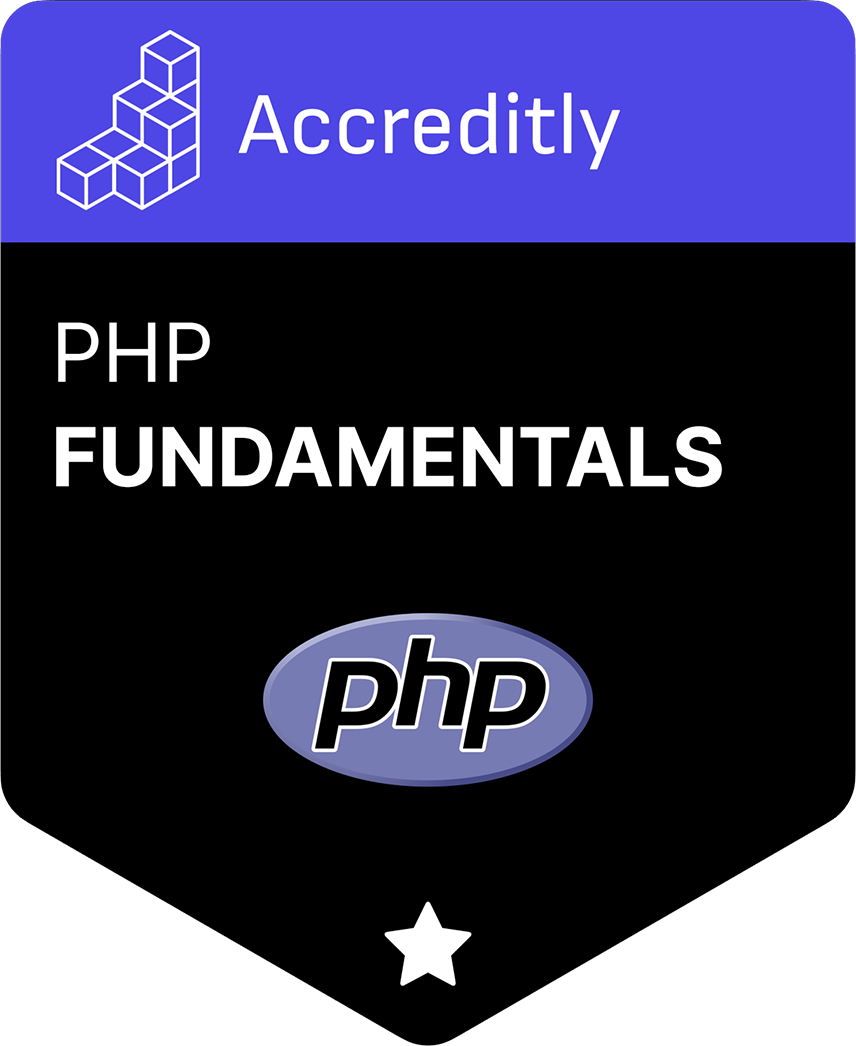