- Understanding the Queue Concept
- Implementing a Basic Queue in JavaScript
- Advanced Queue Operations
- Conclusion
A queue is a fundamental data structure that follows the First In, First Out (FIFO) principle. It's particularly useful in programming for managing tasks, operations, or any data that needs to be processed in the exact order it was added. JavaScript, being a versatile language used both on the client-side and server-side, allows for the easy implementation of queues. This guide will explore how to build a queue system in JavaScript, covering both simple and complex scenarios.
Understanding the Queue Concept
A queue operates under FIFO logic where the first element added to the queue is the first one to be removed. This is akin to people queuing for a bus; the person at the front of the line is the first to board. In programming, queues are useful for task scheduling, asynchronous processing, and managing data buffers.
Implementing a Basic Queue in JavaScript
Let's start by implementing a simple queue with basic operations: enqueue (add to the queue), dequeue (remove from the queue), and peek (look at the front item without removing it).
Step 1: Define the Queue Class
You can implement a queue using an array, but wrapping it in a class is a good practice for encapsulation:
class Queue {
constructor() {
this.items = [];
}
// Add an element to the back of the queue
enqueue(element) {
this.items.push(element);
}
// Remove the element from the front of the queue
dequeue() {
if (this.isEmpty()) {
return 'Queue is empty';
}
return this.items.shift();
}
// View the first element
peek() {
if (this.isEmpty()) {
return 'Queue is empty';
}
return this.items[0];
}
// Check if the queue is empty
isEmpty() {
return this.items.length === 0;
}
// View all elements in the queue
printQueue() {
return this.items.toString();
}
}
Step 2: Using the Queue
Now that you have a queue class, here’s how you can use it in a JavaScript application:
const queue = new Queue();
// Adding elements to the queue
queue.enqueue("John");
queue.enqueue("Jane");
queue.enqueue("Joe");
console.log(queue.printQueue()); // Outputs: John,Jane,Joe
// Processing elements in the queue
console.log(queue.dequeue()); // Outputs: John
console.log(queue.peek()); // Outputs: Jane
console.log(queue.printQueue()); // Outputs: Jane,Joe
Advanced Queue Operations
For more complex scenarios, such as prioritizing elements or handling concurrency, you might need to extend the basic queue functionality.
Priority Queue
A priority queue is an extension of the queue concept where each element also has a priority level, and elements are removed based on their priority, not just their order in the queue.
class PriorityQueue extends Queue {
enqueue(element, priority) {
const queueElement = { element, priority };
let added = false;
for (let i = 0; i < this.items.length; i++) {
if (queueElement.priority < this.items[i].priority) {
this.items.splice(i, 0, queueElement);
added = true;
break;
}
}
if (!added) {
this.items.push(queueElement);
}
}
dequeue() {
if (this.isEmpty()) {
return 'Priority Queue is empty';
}
return this.items.shift().element;
}
// Override printQueue to display priorities
printQueue() {
return this.items.map(item => `${item.element} - ${item.priority}`).toString();
}
}
Concurrency Handling
In a Node.js environment, where you might be handling asynchronous tasks, you can use queues to manage concurrency:
async function processQueue() {
while (!queue.isEmpty()) {
const task = queue.dequeue();
await handleTask(task); // Assume handleTask returns a promise
}
console.log('All tasks processed');
}
Conclusion
Building a queue system in JavaScript is a straightforward yet powerful way to manage data and tasks sequentially. By understanding basic implementations and exploring more advanced concepts like priority queues or concurrency management, you can enhance the efficiency and functionality of your JavaScript applications. Whether for simple task management or complex data processing, queues provide a robust structure for organizing and handling data in an orderly fashion.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
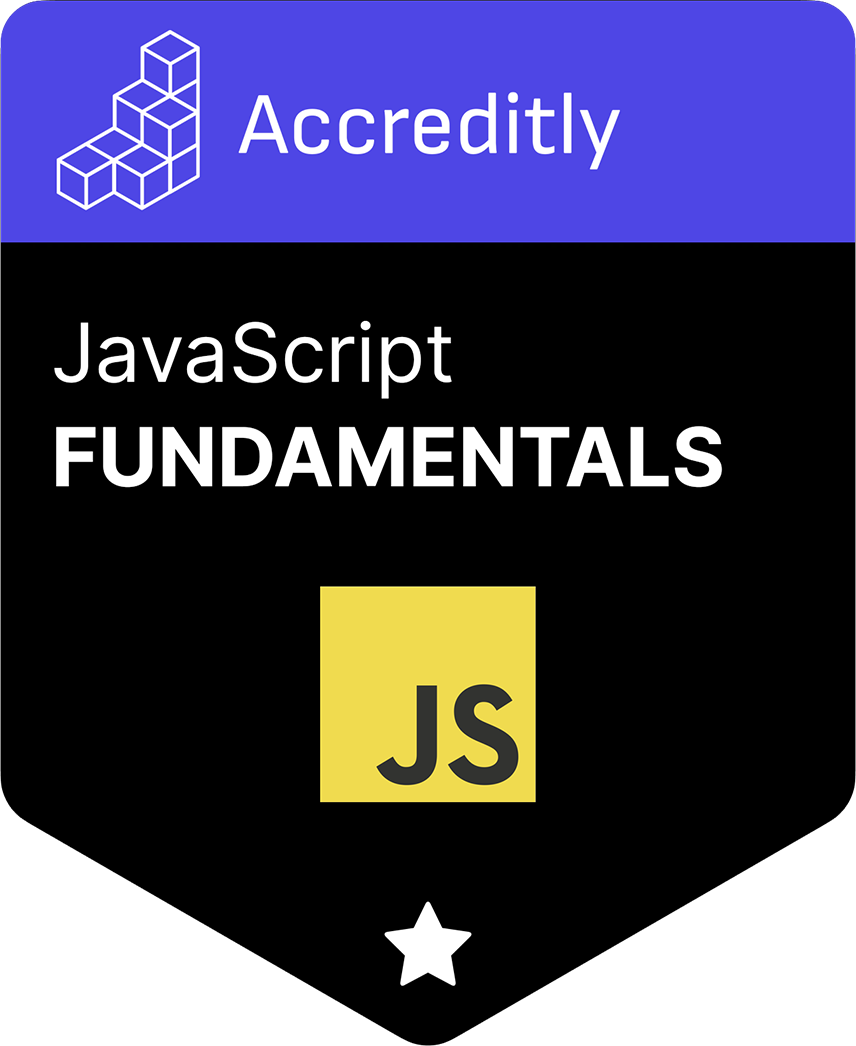