- What is an IIFE?
- Syntax of an IIFE
- Why Use IIFEs?
- Basic IIFE Example
- IIFE with Parameters
- Use Cases for IIFEs
- Variations of IIFE Syntax
- Summing up
Immediately-Invoked Function Expressions (IIFE, pronounced as "iffy") are a fundamental concept in JavaScript, enabling developers to execute functions as soon as they are defined. IIFE is a design pattern used to encapsulate code within a local scope, providing both privacy and state throughout a program’s lifetime. Let’s unravel the intricacies of IIFEs and understand their significance in JavaScript development.
What is an IIFE?
An IIFE is a JavaScript function that runs as soon as it is defined. It’s a self-executing anonymous function that creates a private namespace, preventing variable and function conflicts between different JavaScript modules and libraries.
Syntax of an IIFE
The basic syntax of an IIFE involves declaring a function and then immediately invoking it:
(function() {
// Code goes here
})();
Here, the function is wrapped in parentheses ()
, and then immediately invoked with another set of parentheses ()
.
Why Use IIFEs?
IIFEs offer several benefits in JavaScript programming:
- Encapsulation: By enclosing variables and functions within an IIFE’s scope, you prevent them from polluting the global scope.
- Privacy: Variables or functions defined within an IIFE are not accessible from the outside scope, ensuring privacy.
- Immediate Execution: IIFEs are executed as soon as they are defined, which is useful for initializing code.
- Management of Global Variables: You can pass global variables as arguments to an IIFE, making them local to the function’s scope.
Basic IIFE Example
Here’s a simple example of an IIFE:
(function() {
var localVar = 'I am local';
console.log(localVar); // Outputs: I am local
})();
console.log(localVar); // Error: localVar is not defined
The variable localVar
is not accessible outside the IIFE.
IIFE with Parameters
IIFEs can also accept parameters. This is particularly useful for aliasing global objects or passing in configuration data:
(function($, window) {
// You can use $ for jQuery and window as a local variable here
})(jQuery, window);
Use Cases for IIFEs
- Avoiding Global Scope Pollution: In a large application, limiting the scope of variables is crucial to avoid conflicts.
(function() {
var name = 'John Doe';
// Code that uses 'name'
})();
- Module Pattern: IIFEs are the foundation of the module pattern in JavaScript, where you can create public and private access levels.
var myModule = (function() {
var privateVar = 'I am private';
return {
publicMethod: function() {
console.log(privateVar);
}
};
})();
myModule.publicMethod(); // Outputs: I am private
console.log(myModule.privateVar); // Undefined
- Immediately Executing Complex Logic: For code that needs to run immediately but also needs to be separated from the global scope.
(function() {
var result = complexCalculation();
// Do something with the result
})();
Variations of IIFE Syntax
While the typical IIFE syntax uses parentheses, there are other variations:
- With a Unary Operator:
!function() {}();
- With a Void Operator:
void function() {}();
These variations also ensure the function is treated as an expression, enabling immediate invocation.
Summing up
IIFEs are a crucial part of JavaScript programming, providing an elegant solution for executing code immediately while maintaining a clean global namespace. Understanding and effectively using IIFEs can greatly enhance the structure, privacy, and performance of your JavaScript code.
In summary, IIFEs are more than just a pattern; they represent a mindset in JavaScript development that values encapsulation, namespace management, and modular coding. Whether you’re building simple scripts or complex web applications, IIFEs provide the groundwork for maintainable and conflict-free JavaScript code.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
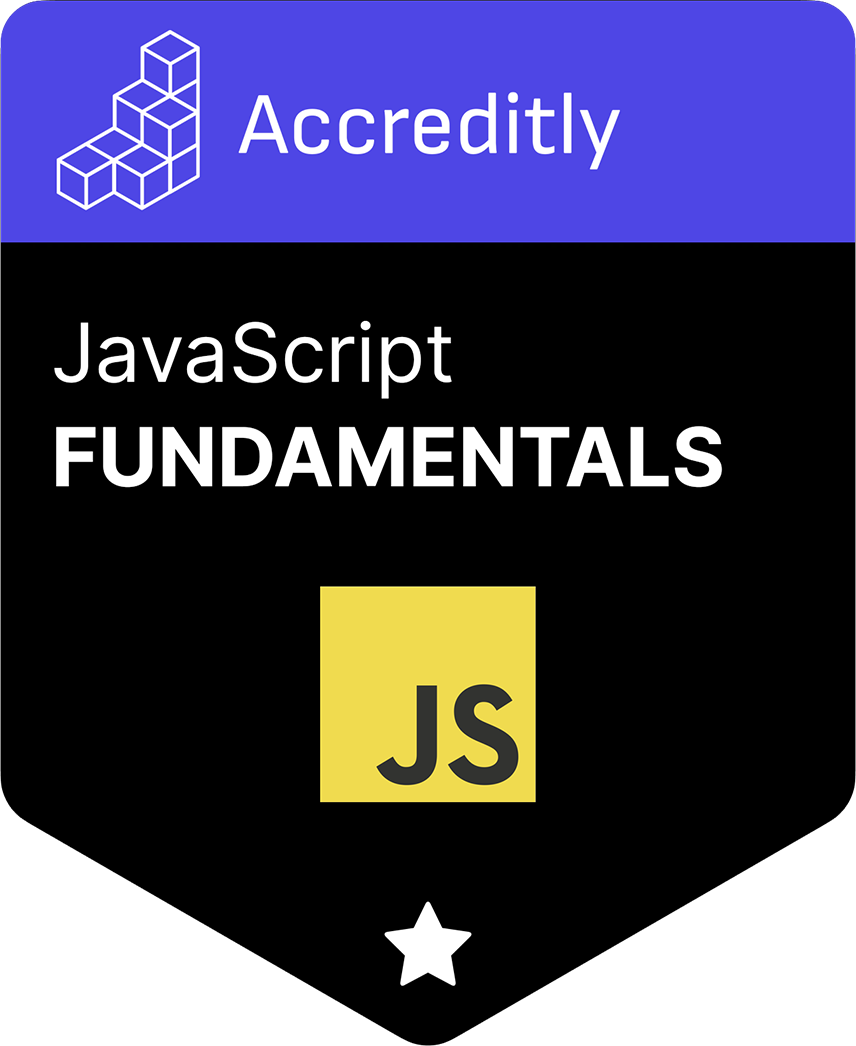