- 1. Understanding the SPA Architecture
- 2. Initial Setup and Structure
- 3. Implementing a Basic Router
- 4. Managing Views
- 5. Handling Navigation
- 6. State Management
- 7. Fetching and Displaying Data
- 8. Enhancements and Best Practices
- Conclusion
Single Page Applications (SPAs) are a popular choice for creating seamless, dynamic web experiences. While JavaScript frameworks are commonly used for SPA development, Vanilla JavaScript is a powerful tool for building efficient SPAs, offering deeper control and understanding. This guide will walk you through creating an SPA with Vanilla JavaScript, touching on various aspects including router creation.
1. Understanding the SPA Architecture
An SPA loads a single HTML page and dynamically updates content as users interact with the app, contrary to traditional web applications that reload pages from the server. Key components include:
-
Router: Handles URL changes and displays the correct content without reloading the page.
-
View Management: Controls what is displayed on the screen.
-
Data Management: Manages data retrieval, storage, and manipulation.
2. Initial Setup and Structure
Create a basic HTML structure in an index.html
file. This file serves as the container for all SPA views:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My SPA</title>
</head>
<body>
<nav>
<a href="/" class="route">Home</a>
<a href="/about" class="route">About</a>
</nav>
<div id="app"></div>
<script src="app.js"></script>
</body>
</html>
3. Implementing a Basic Router
A simple router in Vanilla JavaScript can manage URL changes:
// Inside app.js
function handleRouteChange() {
const path = window.location.pathname;
// Logic to render the view based on the path
}
window.addEventListener('popstate', handleRouteChange);
// Initialize the router
handleRouteChange();
This basic router listens for URL changes and calls handleRouteChange
to update the view.
Building a comprehensive router is a task in itself, so check out our article on building a router in vanilla JS.
4. Managing Views
Views are the different "pages" or content that users see. Create functions to render each view:
function homeView() {
return '<h1>Home Page</h1>';
}
function aboutView() {
return '<h1>About Page</h1>';
}
// Update handleRouteChange to render views
function handleRouteChange() {
const path = window.location.pathname;
let view;
switch (path) {
case '/about':
view = aboutView();
break;
default:
view = homeView();
}
document.getElementById('app').innerHTML = view;
}
5. Handling Navigation
Intercept click events on navigation links to update the URL without reloading the page:
document.querySelectorAll('.route').forEach(link => {
link.addEventListener('click', function(e) {
e.preventDefault();
history.pushState(null, '', this.href);
handleRouteChange();
});
});
6. State Management
Managing state in an SPA is crucial. Implement simple state management to update and render views based on data changes.
7. Fetching and Displaying Data
Use the Fetch API to retrieve data as needed:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Render view with data
});
8. Enhancements and Best Practices
-
Optimize Performance: Use efficient DOM manipulation and avoid unnecessary re-renders.
-
Improve Router: Enhance the router to handle dynamic routes and query parameters.
-
Organize Code: Modularize your JavaScript code for better maintainability.
-
Accessibility: Ensure your SPA is accessible to all users.
Conclusion
Building an SPA with Vanilla JavaScript is a rewarding endeavor that sharpens your skills in front-end development. By understanding and implementing each part of an SPA, from routing to state management, you can create fast, responsive, and user-friendly web applications without relying on heavy frameworks.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
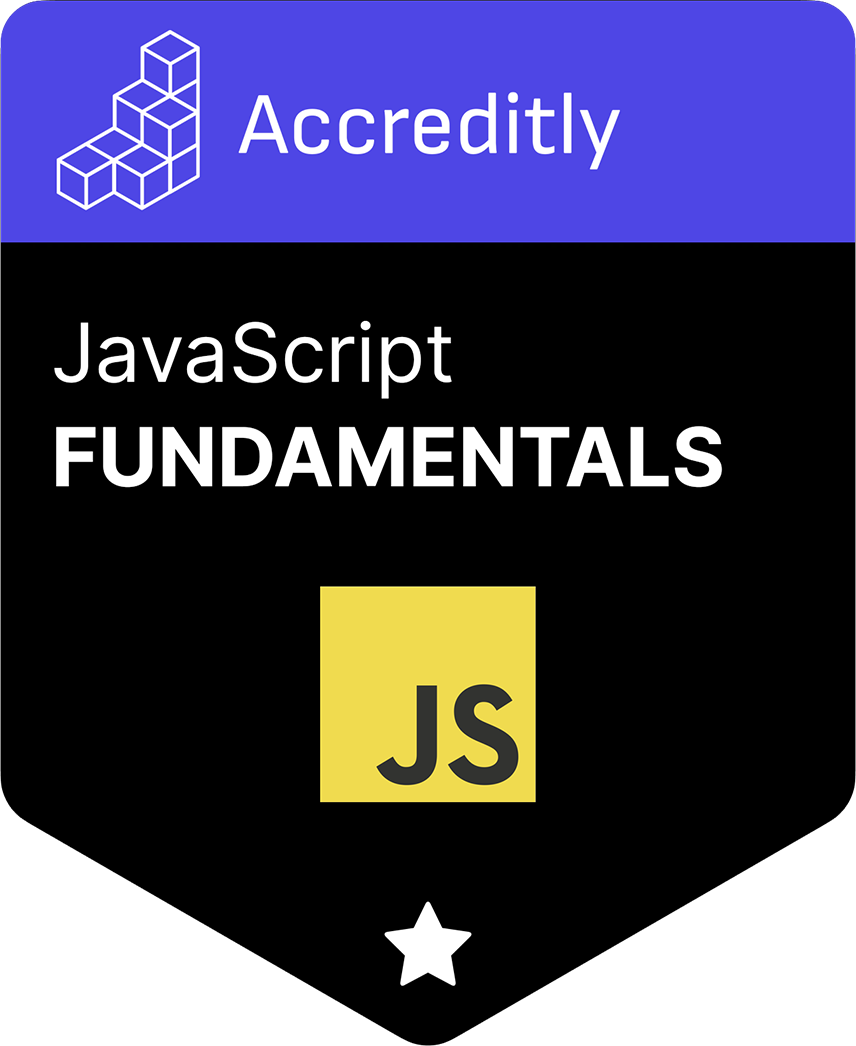