- What is BSON?
- What is GSON?
- BSON vs. GSON: A Comparative Overview
- Conclusion: Choosing the Right Tool
In the world of data interchange formats, JSON (JavaScript Object Notation) is the undisputed king due to its simplicity and readability. However, for specific use cases, JSON isn't always the most efficient option. This is where BSON and GSON come into play, providing alternative formats for different requirements. In this article, we will explore what BSON and GSON are, their advantages, disadvantages, and how they fit into the data interchange ecosystem.
What is BSON?
BSON stands for Binary JSON. It is a binary-encoded serialization of JSON-like documents. BSON was developed to address some of the inefficiencies of JSON, particularly for use with databases and data transfer scenarios where performance and storage are critical.
Key Characteristics of BSON
- Binary Encoding: Unlike JSON, which is a text format, BSON is binary-encoded. This makes it more efficient for both storage and data retrieval.
- Support for More Data Types: BSON supports more data types than JSON, such as Date and BinData (binary data).
- Efficient for Data Size: BSON includes length prefixes for elements and subobjects, making it easier and faster to traverse.
- Ideal for Databases: BSON is particularly useful for databases that need to store large amounts of data and require fast read/write operations.
BSON in Action: MongoDB
MongoDB, a popular NoSQL database, uses BSON as its data storage format. BSON allows MongoDB to encode and decode data quickly, and its extended data types are particularly useful in a database context. Here is an example of BSON usage in MongoDB:
// Insert a document into a MongoDB collection
db.collection.insertOne({
name: "John Doe",
age: 29,
birthdate: new Date("1994-04-12"),
binaryData: new BinData(0, "data")
});
Advantages of BSON
- Performance: Binary encoding makes BSON faster for read/write operations compared to JSON.
- Compactness: Generally results in smaller file sizes, which can save on storage costs and improve transmission speeds.
- Rich Data Types: Supports a wider range of data types.
Disadvantages of BSON
- Readability: Being a binary format, BSON is not human-readable, unlike JSON.
- Complexity: Slightly more complex to handle due to its binary nature and additional data types.
What is GSON?
GSON is a Java library developed by Google for converting Java objects into their JSON representation and vice versa. Unlike BSON, GSON focuses on ease of use and flexibility within Java applications.
Key Characteristics of GSON
- Serialization and Deserialization: GSON provides easy methods to serialize Java objects to JSON and deserialize JSON back to Java objects.
- Custom Serialization: Allows customization of the serialization and deserialization process, making it very flexible.
- Handling of Nulls: Provides options to include or exclude null fields from the JSON output.
- Pretty Printing: Supports formatted (pretty-printed) output for better readability.
GSON in Action: Java Applications
GSON is widely used in Java applications, especially in scenarios where data needs to be converted to and from JSON format for web services, APIs, and configuration files. Here is an example of using GSON in a Java application:
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class GsonExample {
public static void main(String[] args) {
// Creating a sample Java object
User user = new User("John Doe", 29);
// Creating a Gson instance
Gson gson = new GsonBuilder().setPrettyPrinting().create();
// Serializing the Java object to JSON
String json = gson.toJson(user);
System.out.println(json);
// Deserializing the JSON back to a Java object
User deserializedUser = gson.fromJson(json, User.class);
System.out.println(deserializedUser.getName());
}
}
class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Advantages of GSON
- Ease of Use: Simplifies the process of converting between Java objects and JSON.
- Flexibility: Highly customizable for different serialization needs.
- Integration with Java: Designed to work seamlessly with Java applications.
Disadvantages of GSON
- Java-Only: Primarily useful in Java environments, limiting its use across different programming languages.
- Performance: May not be as performant as some other JSON libraries, especially for very large datasets.
BSON vs. GSON: A Comparative Overview
Use Cases
- BSON: Ideal for use cases requiring efficient storage and fast read/write operations, such as databases (e.g., MongoDB).
- GSON: Best suited for Java applications needing flexible and easy JSON serialization/deserialization.
Performance
- BSON: Generally faster and more compact due to its binary nature.
- GSON: Performance is adequate for most Java applications, but not optimized for large-scale data storage or transfer.
Readability
- BSON: Not human-readable due to its binary format.
- GSON: Human-readable, especially with pretty printing enabled.
Supported Data Types
- BSON: Supports a wider range of data types, making it versatile for various applications.
- GSON: Limited to the data types supported by JSON, which are sufficient for many typical uses but not as extensive as BSON.
Conclusion: Choosing the Right Tool
When deciding between BSON and GSON, the choice largely depends on your specific needs:
- BSON: Choose BSON if you need efficient storage and fast operations, particularly in a database context like MongoDB. Its binary format and rich data type support make it ideal for these scenarios.
- GSON: Opt for GSON if you're working within a Java environment and need a flexible, easy-to-use tool for converting Java objects to and from JSON. Its customization options and ease of integration with Java make it a strong choice for application development.
Understanding the strengths and weaknesses of both BSON and GSON will help you make an informed decision that best suits your project's requirements. Whether you need the efficiency of BSON or the flexibility of GSON, both tools provide robust solutions for their respective use cases.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
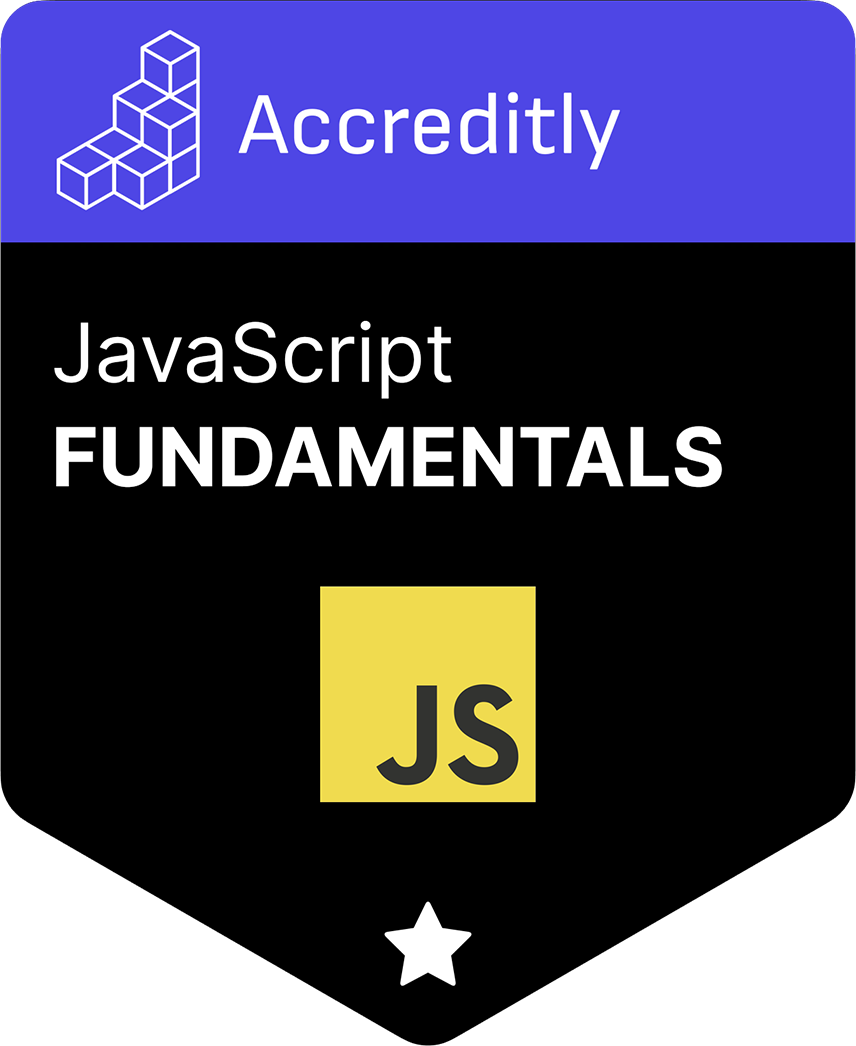